- お役立ち記事
- Basics of image processing using OpenCV and Python and application to system development
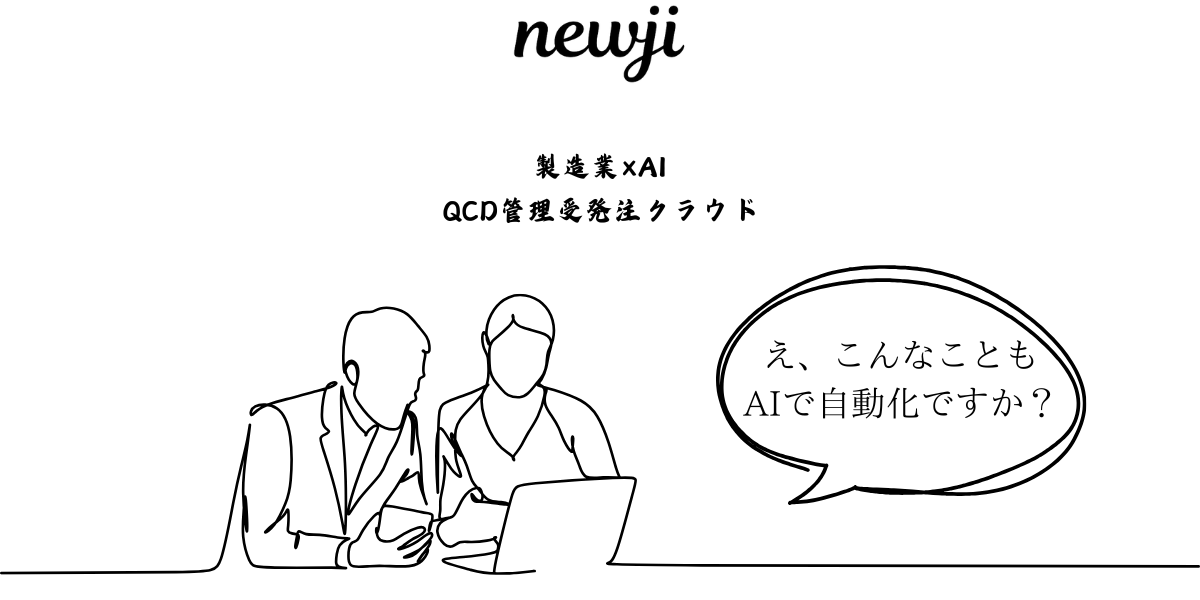
Basics of image processing using OpenCV and Python and application to system development
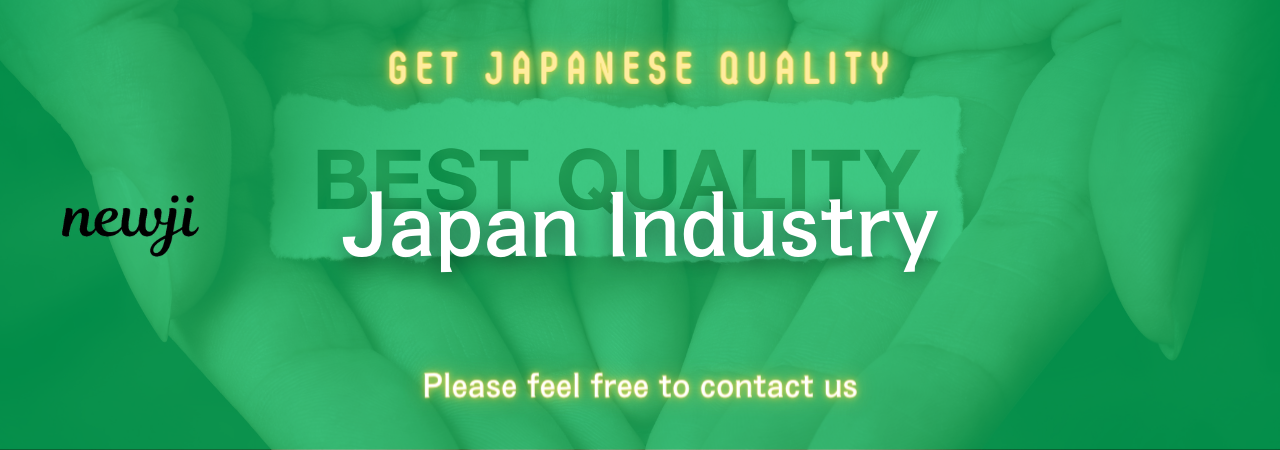
目次
Introduction to Image Processing
Image processing is a crucial aspect of computer vision and is widely utilized across various industries, from healthcare to automotive systems.
It involves the manipulation and analysis of images to extract meaningful information and enhance visual quality.
With the advancement in machine learning and artificial intelligence, image processing has become more accessible and powerful.
One of the most popular libraries for image processing is OpenCV (Open Source Computer Vision Library), which, when combined with Python, offers a robust framework for developing image-based applications.
Python’s simplicity and OpenCV’s comprehensive functions make them an ideal combination for beginners and professionals alike.
Getting Started with OpenCV and Python
Before diving into image processing, ensure that you have both Python and OpenCV installed on your machine.
You can install OpenCV using Python’s package manager, pip, with the command:
“`bash
pip install opencv-python
“`
Once installed, you can begin by importing the OpenCV library in your Python script:
“`python
import cv2
“`
OpenCV provides a range of functionalities from reading and writing images to transforming them in various ways.
Here’s a simple example to read and display an image:
“`python
import cv2
# Load an image
image = cv2.imread(‘your-image-file.jpg’)
# Display the image
cv2.imshow(‘Display Window’, image)
# Wait for a key press and close the window
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
This basic setup is the foundation on which you can build more complex image processing tasks.
Basic Image Processing Techniques
1. Image Resizing
Resizing images is a common preprocessing step, especially when working with deep learning models that require input images of a specific size.
OpenCV offers simple methods to resize images:
“`python
resized_image = cv2.resize(image, (width, height))
“`
Replace `width` and `height` with the desired dimensions for the image.
2. Converting Images to Grayscale
Color images have a lot of data, which sometimes is not necessary for certain tasks like edge detection.
Converting images to grayscale reduces this complexity:
“`python
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
“`
3. Gaussian Blurring
Blurring is used to reduce noise and detail in an image, which can be helpful in various applications like edge detection and face detection:
“`python
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
“`
The kernel size (5, 5) can be adjusted to increase or decrease the amount of blurring.
Advanced Image Processing Techniques
1. Edge Detection
Detecting edges in an image is a fundamental technique that helps in understanding the geometry and structure of objects within an image.
The Canny edge detection algorithm is one of the most effective methods available in OpenCV:
“`python
edges = cv2.Canny(gray_image, threshold1, threshold2)
“`
You can experiment with `threshold1` and `threshold2` to find the optimal values for your specific use case.
2. Contour Detection
Contours are curves joining all the continuous points along a boundary with the same color or intensity.
Contour detection is vital in shape analysis and object detection:
“`python
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
“`
You can then use these contours to draw or measure different shapes in your image.
Application to System Development
1. Automated Inspection Systems
One of the practical applications of image processing with OpenCV is in developing automated inspection systems.
These systems are used in manufacturing industries to detect defects in products on the assembly line.
By using techniques such as edge detection and contour analysis, software can identify and filter out defective items.
2. Facial Recognition Systems
Facial recognition is another popular application enabled by image processing.
With OpenCV, you can develop systems that can detect and recognize faces in real-time.
This is achieved by combining face detection algorithms with pre-trained classifiers.
3. Augmented Reality
Augmented reality overlays digital content on real-world images, creating immersive experiences.
OpenCV’s powerful image processing capabilities allow developers to track, detect, and map real-world images onto which augmented content can be superimposed.
Conclusion
The basics of image processing with OpenCV and Python open up a world of opportunities for system development.
By understanding key techniques such as image resizing, blurring, and edge detection, developers can create sophisticated applications across various domains.
The versatility of OpenCV makes it an invaluable tool in any developer’s arsenal, capable of transforming how we interpret and interact with digital images.
As you continue to refine your skills in image processing, you’ll discover even more advanced techniques and applications that can revolutionize industries yet to fully embrace the digital transformation possibilities offered by OpenCV and Python.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)