- お役立ち記事
- Basics of Rust programming, points for effective use, and its practice
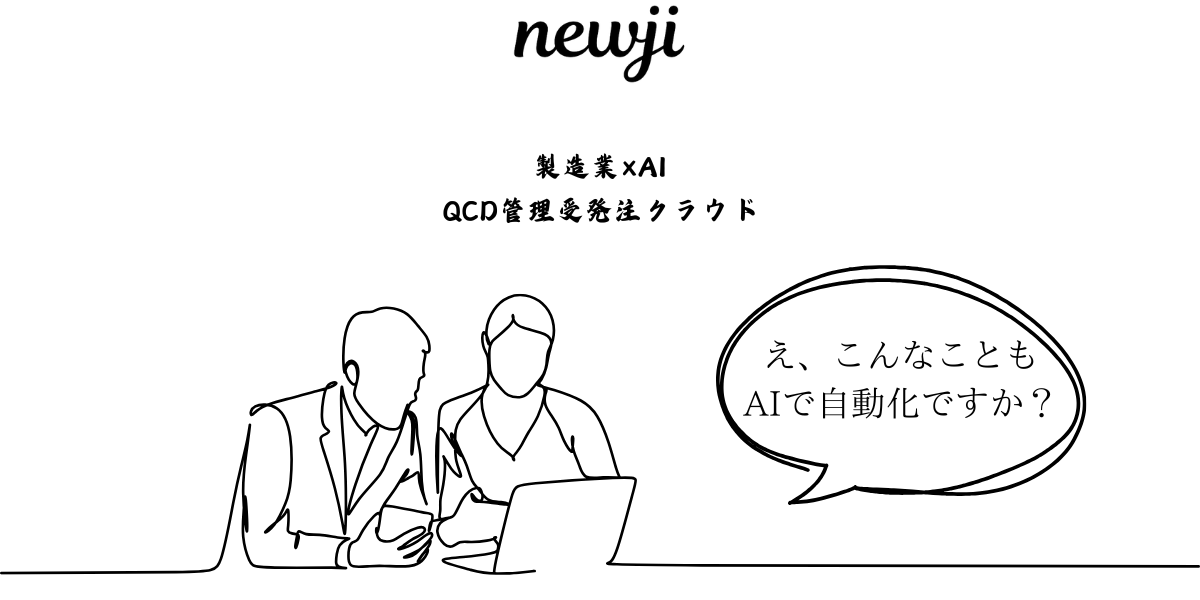
Basics of Rust programming, points for effective use, and its practice
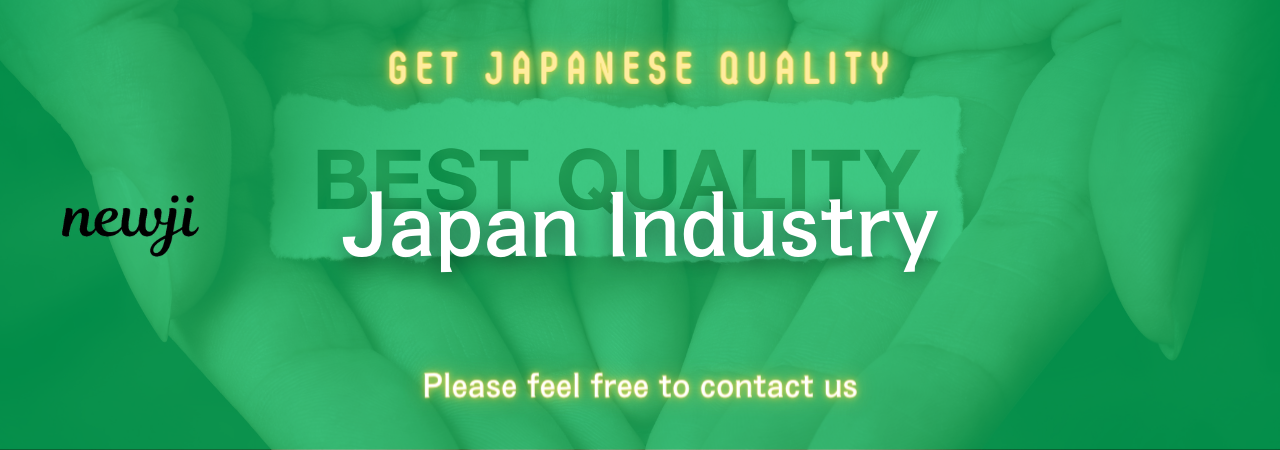
目次
What is Rust Programming?
Rust is a systems programming language that was developed by Mozilla Research.
It was designed to be fast, safe, and concurrent, with a strong focus on memory safety.
Unlike other programming languages like C and C++, Rust eliminates common bugs and vulnerabilities caused by memory-related errors.
With Rust, developers can build reliable and efficient software.
Its syntax is similar to that of C++, making it easier for those who are familiar with C-based languages to learn.
However, Rust introduces unique features that enhance safety and performance.
Key Features of Rust
Memory Safety
One of Rust’s standout features is its ability to manage memory safely.
This is achieved through a system of ownership with a set of rules that the compiler checks at compile time.
This system prevents data races and null pointer dereferencing, which are common issues in languages like C and C++.
Concurrency
Rust offers excellent support for concurrent programming.
It enables developers to write programs that execute multiple processes simultaneously without causing data races.
This is crucial for building high-performance applications that can take advantage of modern multi-core processors.
Zero-Cost Abstractions
Rust provides abstractions without sacrificing performance.
Unlike some languages that abstract away details at the cost of speed, Rust allows developers to write high-level code that compiles down to efficient machine code.
Pattern Matching
Rust includes a powerful pattern matching feature that allows developers to match complex patterns in data structures.
This simplifies many tasks and leads to more readable and maintainable code.
Points for Effective Use of Rust
Understand Ownership and Borrowing
To effectively use Rust, understanding the concepts of ownership, borrowing, and lifetimes is crucial.
These unique features of Rust help manage memory safety and are foundational to the language.
Ownership ensures that each value in Rust has a single owner, and when that owner goes out of scope, the value is automatically deallocated.
Borrowing allows multiple parts of the code to access a piece of data without taking ownership.
This must be done in a way that adheres to Rust’s borrowing rules.
Utilize Rust’s Powerful Type System
Rust has a robust type system that helps catch errors at compile time.
By leveraging this system, you can create safer and less error-prone applications.
Understanding how to use enums, structs, and traits can help you effectively express your program’s logic and improve code maintainability.
Embrace Rust’s Tooling
Rust comes with a package manager named Cargo, which simplifies managing dependencies, building projects, and running tests.
Getting comfortable with Cargo and Rust’s integrated testing tools will streamline your development workflow.
Additionally, Rust’s documentation and community resources are invaluable for learning and troubleshooting.
Practice: Building a Simple Application in Rust
To get hands-on experience with Rust, let’s walk through building a simple command-line application.
Setting Up Your Environment
Start by installing Rust and Cargo.
Visit the official Rust website for installation instructions that suit your operating system.
Once installed, verify the installation by running `rustc –version` and `cargo –version` in your terminal.
Creating a Rust Project
Open your terminal and navigate to the directory where you want to create your project.
Run the command `cargo new my_project` to create a new Rust project named `my_project`.
This command will generate a directory with a basic structure, including a `Cargo.toml` file for managing dependencies and a `src` folder containing a `main.rs` file.
Writing Your First Rust Program
Navigate to the `src` folder and open `main.rs`.
This file will contain the main logic of your application.
To start, replace the existing code with the following:
“`rust
fn main() {
println!(“Hello, world!”);
}
“`
This simple program prints “Hello, world!” to the console.
Building and Running Your Application
Return to the terminal and run `cargo build` to compile your program.
To execute it, use the command `cargo run`.
You should see “Hello, world!” printed in the terminal.
Enhancing Your Program
Let’s expand this program to perform a task, such as adding two numbers.
Modify the `main.rs` file to include a function for addition:
“`rust
fn main() {
let sum = add(5, 10);
println!(“The sum of 5 and 10 is: {}”, sum);
}
fn add(a: i32, b: i32) -> i32 {
a + b
}
“`
This program now defines a function `add` that takes two integers and returns their sum.
When you run the program, it will output the result of adding 5 and 10.
Conclusion
Rust is a powerful language for developers looking to create fast and safe applications.
Its unique features like memory safety, concurrency, and zero-cost abstractions set it apart from other programming languages.
By understanding Rust’s core concepts and practicing building simple applications, you can effectively harness the potential of Rust.
Remember to explore further with Rust’s comprehensive documentation and engage with its supportive community for continual learning.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)