- お役立ち記事
- Basics of anomaly detection using data and practical points using Python
Basics of anomaly detection using data and practical points using Python
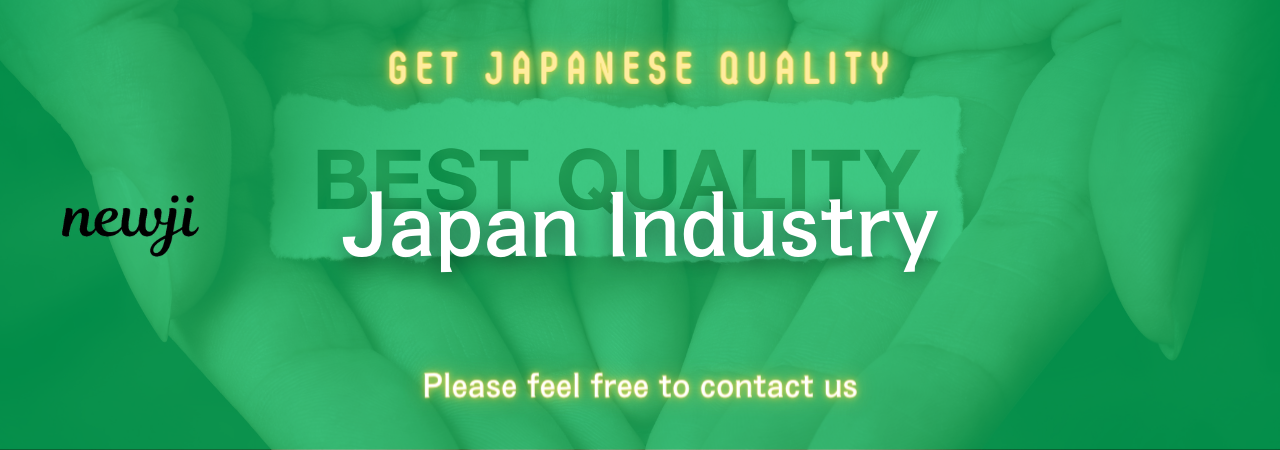
目次
Understanding Anomaly Detection
Anomaly detection is a crucial aspect of data analysis, especially in fields where unusual patterns or outliers can signify important changes or critical conditions.
To put it simply, anomaly detection is the process of identifying unexpected items, events, or observations that differ significantly from the majority of the data.
These outliers can point to significant insights or issues that need addressing.
For instance, in finance, an abnormal transaction might indicate fraud.
In healthcare, it could suggest a deviation in a patient’s vital signs that require attention.
Why Anomaly Detection Matters
In today’s data-driven world, accurate anomaly detection can save time, resources, and even lives.
It helps in identifying potential risks before they escalate into problems.
By automating the detection process, organisations can efficiently monitor their data in real-time, reducing the need for manual oversight and minimising error rates.
Anomaly detection also helps improve system reliability and enhances decision-making processes by providing trustworthy insights into the data being analysed.
Understanding and implementing effective anomaly detection mechanisms allows businesses to maintain a competitive edge in their industry.
Approaches to Anomaly Detection
There are various approaches to detect anomalies, each with its own strengths and applications.
Statistical Methods
Statistical methods rely on building a model of data using its variance, mean, distribution, and other related statistical traits.
If a new data point deviates significantly from this established model, it is flagged as an anomaly.
These methods are quite effective for data that follows a known distribution but may struggle with datasets lacking clear statistical patterns.
Machine Learning Models
Machine learning models are more flexible and powerful when dealing with complex and large datasets.
These methods include supervised, unsupervised, and semi-supervised learning.
– **Supervised Anomaly Detection**: Requires a labeled dataset with both normal and anomalous samples to train the model.
This can be costly and time-consuming as it requires a comprehensive labelled dataset.
– **Unsupervised Anomaly Detection**: Does not require labeled data and is ideal when a model must detect novel patterns or outliers across varying data distributions.
It often uses clustering or dimensionality reduction techniques.
– **Semi-Supervised Anomaly Detection**: Focuses on training a model primarily on normal data so that it can recognize anomalous points that deviate from the learned patterns.
Distance-Based Methods
These methods calculate the distance measurements between data points.
If a point is far from its neighbors beyond a threshold, it is considered an anomaly.
These methods are effective for small-scale datasets and are computationally simple.
Domain Knowledge-Based Methods
Incorporating domain knowledge in the detection process ensures high accuracy and relevance.
This approach is especially useful for specific industries where anomalies are defined by domain-specific attributes and context.
Implementing Anomaly Detection Using Python
Python offers a suite of libraries that are particularly useful for anomaly detection tasks, thanks to its extensive and flexible data analysis capabilities.
Here’s a basic guide to implementing anomaly detection in Python using popular libraries.
Using SciPy for Statistical Methods
SciPy provides a range of statistical functions that can be used for anomaly detection.
For instance, you can calculate z-scores to identify data points that deviate significantly from the mean:
“`python
from scipy import stats
data = [10, 12, 12, 13, 13, 14, 14, 15, 15, 100]
z_scores = stats.zscore(data)
anomalies = [data[i] for i in range(len(z_scores)) if abs(z_scores[i]) > 2]
print(“Anomalies:”, anomalies)
“`
Here, the number `100` appears as an outlier because its z-score exceeds the threshold of `2`.
Using Scikit-Learn for Machine Learning Methods
Scikit-Learn includes multiple robust methods for anomaly detection, such as the Isolation Forest and One-Class SVM:
“`python
from sklearn.ensemble import IsolationForest
data = [[-1.1], [0.2], [101.1], [0.3]]
model = IsolationForest(contamination=0.1) # You can adjust contamination to define the percentage of outliers
model.fit(data)
anomalies = model.predict(data)
print(“Anomalies:”, [data[i] for i in range(len(anomalies)) if anomalies[i] == -1])
“`
In this script, the Isolation Forest model is utilised to detect anomalies in the given dataset.
Visualizing Anomalies with Matplotlib
Visualisation of anomalies can provide intuitive insights.
Matplotlib can be used alongside detection methods to plot data and highlight anomalies:
“`python
import matplotlib.pyplot as plt
import numpy as np
data = np.array([10, 12, 12, 13, 13, 14, 14, 15, 15, 100])
z_scores = stats.zscore(data)
anomalies = [data[i] for i in range(len(z_scores)) if abs(z_scores[i]) > 2]
plt.plot(data, ‘b.’)
plt.plot(anomalies, ‘ro’)
plt.title(‘Anomalies in Data’)
plt.show()
“`
This code snippet highlights anomalies in red, making it easier to visually discern outliers from normal data points.
Practical Considerations for Anomaly Detection
When implementing anomaly detection, it’s essential to keep certain key considerations in mind.
Define Criteria for Anomalies
Clearly defining what constitutes an anomaly within your specific dataset is crucial.
This definition can significantly vary based on the domain and context of the data.
Regularly Update Detection Models
Data environments are dynamic and often evolve over time.
Therefore, models need continual updates to adapt to new patterns and maintain accuracy.
Consider Computational Resources
The choice of techniques might vary with the system’s computational constraints.
While some methods are resource-lighter, machine learning techniques can demand significant computational power.
Evaluate Performance
Regular evaluation of model performance is important to refine and improve accuracy.
Employ metrics like precision, recall, and the F1 score to assess the effectiveness of your anomaly detection system.
In conclusion, anomaly detection is a pivotal tool for analysing data-driven insights and ensuring operational efficiency.
By employing Python’s versatile libraries, one can effectively manage and implement detection methodologies tailored to quantify and qualify anomalies in real-world datasets.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)