- お役立ち記事
- How to implement deep learning and image recognition models with PyTorch
How to implement deep learning and image recognition models with PyTorch
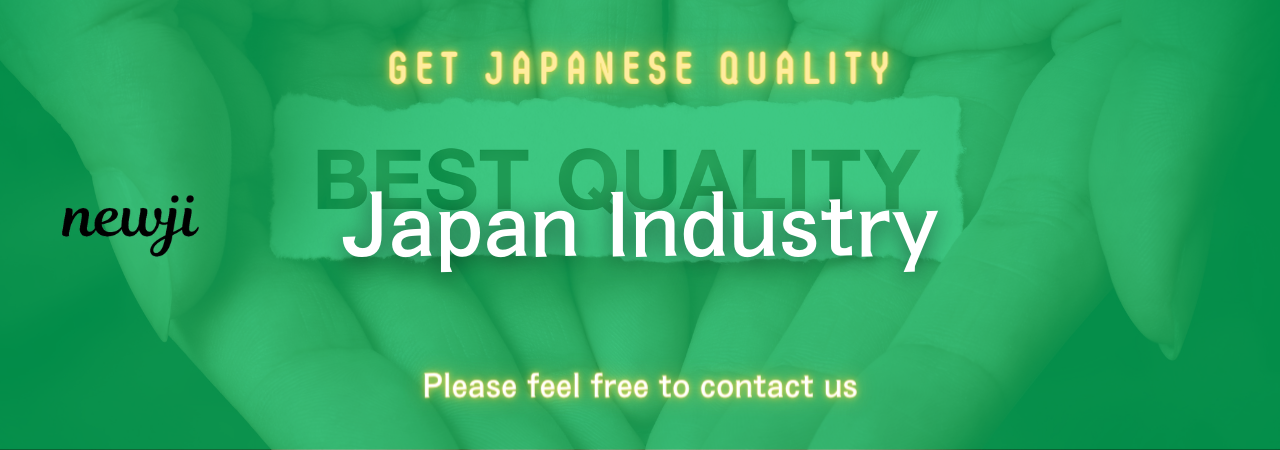
目次
Introduction to Deep Learning and Image Recognition
Deep learning has revolutionized the field of artificial intelligence by enabling machines to learn complex patterns from vast amounts of data.
One of the most exciting applications of deep learning is image recognition, which allows computers to identify and classify objects in images with remarkable accuracy.
To implement deep learning and image recognition models, PyTorch is a popular and powerful framework that many developers and researchers use.
This article will guide you through the process of using PyTorch to build effective deep learning models for image recognition.
Getting Started with PyTorch
PyTorch is an open-source deep learning framework developed by Facebook’s AI Research lab.
It provides a flexible and dynamic way to build and train neural networks, making it an ideal choice for both beginners and advanced users.
To get started with PyTorch, you’ll need to install it on your computer.
The easiest way to install PyTorch is by using the pip package manager.
Open a terminal or command prompt and type the following command to install PyTorch:
“`
pip install torch torchvision
“`
The `torchvision` package contains popular datasets, model architectures, and image transformations for computer vision tasks, which will be helpful for building image recognition models.
Understanding Neural Networks
Before implementing a deep learning model, it’s essential to understand the basic concepts of neural networks.
A neural network is composed of layers of interconnected nodes, known as neurons.
Each neuron takes an input, performs a computation, and passes it to the next layer.
Neural networks learn by adjusting the weights of these connections to minimize the error between the predicted output and the actual target.
In the context of image recognition, convolutional neural networks (CNNs) are commonly used.
CNNs have specialized layers that help them process visual data effectively, making them ideal for tasks like object detection and classification.
Building an Image Recognition Model with PyTorch
Loading Data
The first step in building a deep learning model is to load and preprocess the data.
PyTorch provides the `torchvision.datasets` module, which contains several datasets that can be used for training image recognition models.
For this example, we’ll use the CIFAR-10 dataset, a popular benchmark dataset that contains 60,000 32×32 color images in 10 different classes.
Here’s how to load the CIFAR-10 dataset using PyTorch:
“`python
import torch
import torchvision
import torchvision.transforms as transforms
# Define a transform to normalize the data
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))])
# Load the training and test datasets
train_set = torchvision.datasets.CIFAR10(root=’./data’, train=True, download=True, transform=transform)
train_loader = torch.utils.data.DataLoader(train_set, batch_size=4, shuffle=True)
test_set = torchvision.datasets.CIFAR10(root=’./data’, train=False, download=True, transform=transform)
test_loader = torch.utils.data.DataLoader(test_set, batch_size=4, shuffle=False)
“`
The `transform` variable defines a series of transformations to be applied to the images, including converting them to PyTorch tensors and normalizing them.
Defining the Model
Next, we’ll define a convolutional neural network model using the `torch.nn` module.
PyTorch allows us to create custom models by subclassing the `torch.nn.Module` class.
“`python
import torch.nn as nn
import torch.nn.functional as F
class SimpleCNN(nn.Module):
def __init__(self):
super(SimpleCNN, self).__init__()
self.conv1 = nn.Conv2d(3, 16, 3, 1)
self.conv2 = nn.Conv2d(16, 32, 3, 1)
self.fc1 = nn.Linear(32 * 6 * 6, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, 2, 2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, 2, 2)
x = x.view(-1, 32 * 6 * 6)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
net = SimpleCNN()
“`
This `SimpleCNN` model consists of two convolutional layers followed by three fully connected layers.
The `forward` method defines the forward pass of the network.
Training the Model
After defining the model, we need to specify a loss function and an optimizer to train the model.
The loss function measures how well the model’s predictions match the target labels, and the optimizer updates the model’s weights to minimize this loss.
“`python
import torch.optim as optim
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(net.parameters(), lr=0.001, momentum=0.9)
for epoch in range(10): # Loop over the dataset multiple times
running_loss = 0.0
for i, data in enumerate(train_loader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 2000 == 1999: # Print every 2000 mini-batches
print(f’Epoch {epoch + 1}, Batch {i + 1}, Loss: {running_loss / 2000:.6f}’)
running_loss = 0.0
print(‘Finished Training’)
“`
In this training loop, we iterate over the dataset for multiple epochs, computing the loss, performing backpropagation, and updating the model’s weights.
Evaluating the Model
Once the model is trained, we can evaluate its performance on the test dataset to see how well it generalizes to unseen data.
“`python
correct = 0
total = 0
with torch.no_grad():
for data in test_loader:
images, labels = data
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f’Accuracy: {100 * correct / total:.2f}%’)
“`
By comparing the predicted labels with the true labels, we can calculate the model’s accuracy.
Conclusion
Implementing deep learning and image recognition models with PyTorch is a powerful way to harness the capabilities of artificial intelligence.
With its flexible architecture and a vast range of tools, PyTorch makes it easy to build, train, and evaluate complex models.
By following the steps outlined in this article, you can create your own image recognition models and explore more advanced deep learning architectures to tackle various computer vision challenges.
Whether you’re a student, a researcher, or a developer, PyTorch remains an invaluable tool for your deep learning journey.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)