- お役立ち記事
- Time series data analysis and practical points using Python
Time series data analysis and practical points using Python
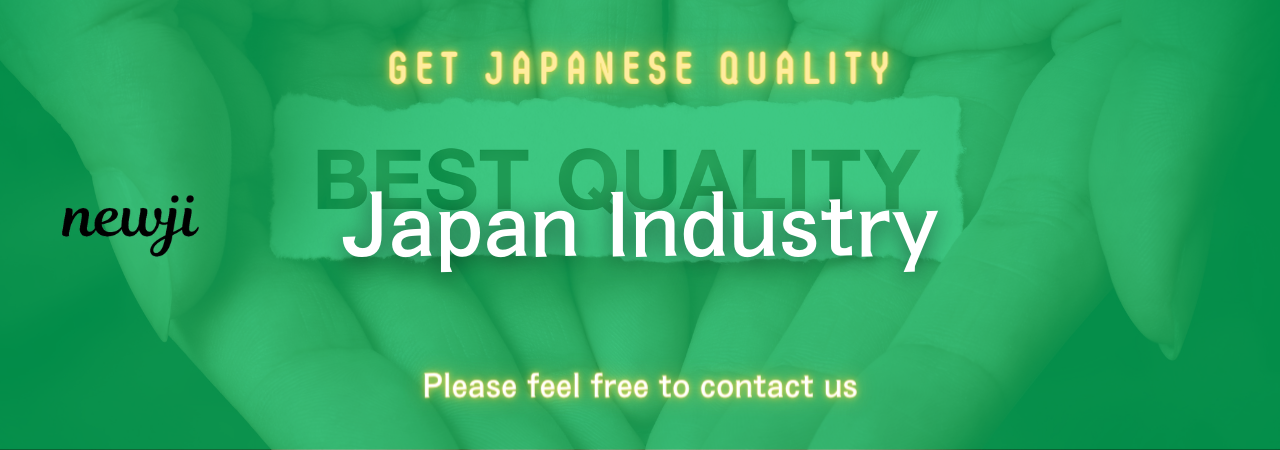
目次
Understanding Time Series Data
Time series data is a sequence of data points collected over time, typically at consistent intervals.
This type of data allows us to analyze patterns, trends, and correlations over time.
It’s fundamental in various fields like finance, economics, weather forecasting, and even in sectors like health and retail.
A good example of time series data is the stock market prices, where each price corresponds to a different time period.
Another example is temperature recordings at different times of the day.
Such data is valuable because it can help predict future outcomes based on historical patterns.
Characteristics of Time Series Data
Time series data has unique characteristics that set it apart from other types of data:
1. **Time Dependency**: The value of a data point often depends on previous points.
2. **Seasonality**: This refers to predictable and repeating patterns over a specific period, like the increased retail sales during holidays.
3. **Trend**: This occurs when there’s a long-term increase or decrease in the data.
4. **Noise**: This is the random variation in the data that does not appear to have any pattern.
Understanding these features is crucial for effective analysis and forecasting.
It can help in choosing the right models and methods for analysis, leading to more accurate results.
Why Use Python for Time Series Analysis?
Python is an excellent choice for time series analysis for several reasons:
– **Extensive Libraries**: Python offers diverse libraries like Pandas, NumPy, Matplotlib, and more, which provide ready-to-use functions for data manipulation, visualization, and analysis.
– **Ease of Use**: With its simple syntax and versatility, Python is user-friendly for both beginners and experts.
– **Community Support**: A large online community contributes to Python’s growth, providing ample resources, forums, and guides.
– **Integration Capabilities**: Python can easily integrate with other data processing and visualization tools.
Overall, Python’s capabilities make it a powerful tool for handling and analyzing time series data efficiently.
Practical Steps in Time Series Analysis Using Python
Now, let’s explore the process of performing time series analysis using Python.
Step 1: Importing the Necessary Libraries
Start by importing essential libraries, as shown below:
“`python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from pandas.plotting import lag_plot
import statsmodels.api as sm
“`
These libraries will help manage data, perform statistical operations, and visualize results.
Step 2: Load and Prepare Your Data
Loading data into Python is a straightforward process, typically done using Pandas:
“`python
df = pd.read_csv(‘your_dataset.csv’)
df[‘Date’] = pd.to_datetime(df[‘Date’])
df.set_index(‘Date’, inplace=True)
“`
Setting a column as the index, particularly when it involves time or date, is crucial as it makes accessing and plotting time series data easier.
Step 3: Visualize Your Data
Visualization is key to understanding time series patterns:
“`python
plt.figure(figsize=(10, 6))
plt.plot(df[‘Value’])
plt.title(‘Time Series Data Plot’)
plt.xlabel(‘Time’)
plt.ylabel(‘Values’)
plt.show()
“`
This initial plot gives a broad look at the data’s trend, seasonality, and potential anomalies.
Step 4: Decompose the Time Series
Decomposition involves breaking down the time series into its constituent components:
“`python
from statsmodels.tsa.seasonal import seasonal_decompose
decompose_result = seasonal_decompose(df[‘Value’], model=’additive’)
decompose_result.plot()
plt.show()
“`
Decomposition helps identify trends, seasonal patterns, and the residual or noise component.
This is crucial for understanding underlying patterns in time series data.
Step 5: Stationarity Check
Stationarity is a property of time series data where statistical properties do not change over time.
This is important for accurately modeling time series:
“`python
from statsmodels.tsa.stattools import adfuller
result = adfuller(df[‘Value’])
print(‘ADF Statistic:’, result[0])
print(‘p-value:’, result[1])
“`
If the p-value is below a certain threshold (commonly 0.05), the data can be considered stationary.
Step 6: Model Selection and Forecasting
Choose an appropriate model like ARIMA, which is often used for time series forecasting:
“`python
from statsmodels.tsa.arima.model import ARIMA
model = ARIMA(df[‘Value’], order=(1, 1, 1))
model_fit = model.fit()
forecast = model_fit.forecast(steps=10)
print(forecast)
“`
Ensure to evaluate the model’s accuracy and revise as necessary.
Performing a train-test split can assist in validating your model.
Handling Common Challenges
Dealing with Missing Data
Time series datasets often contain missing values.
Pandas offers several techniques for handling this issue:
“`python
df[‘Value’] = df[‘Value’].fillna(method=’ffill’) # Forward fill missing values
“`
Depending on the context, different strategies like forward fill, backward fill, or interpolation may be employed.
Avoiding Overfitting
Overfitting occurs when a model is too complex, capturing noise rather than the underlying pattern.
Remedies include simplifying the model, cross-validation, and pruning unimportant variables.
Conclusion
Time series analysis is a powerful tool for uncovering insights and making predictions based on temporal data.
Utilizing Python simplifies this process thanks to its extensive libraries and supportive community.
By applying systematic methods like time series decomposition, stationarity checks, and appropriate modeling, one can effectively analyze time series data and forecast future trends.
Keep these practical points in mind and continue to refine your skills through practice and exploration of new datasets.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)