- お役立ち記事
- Learn machine learning models with Python and their application to image classification
Learn machine learning models with Python and their application to image classification
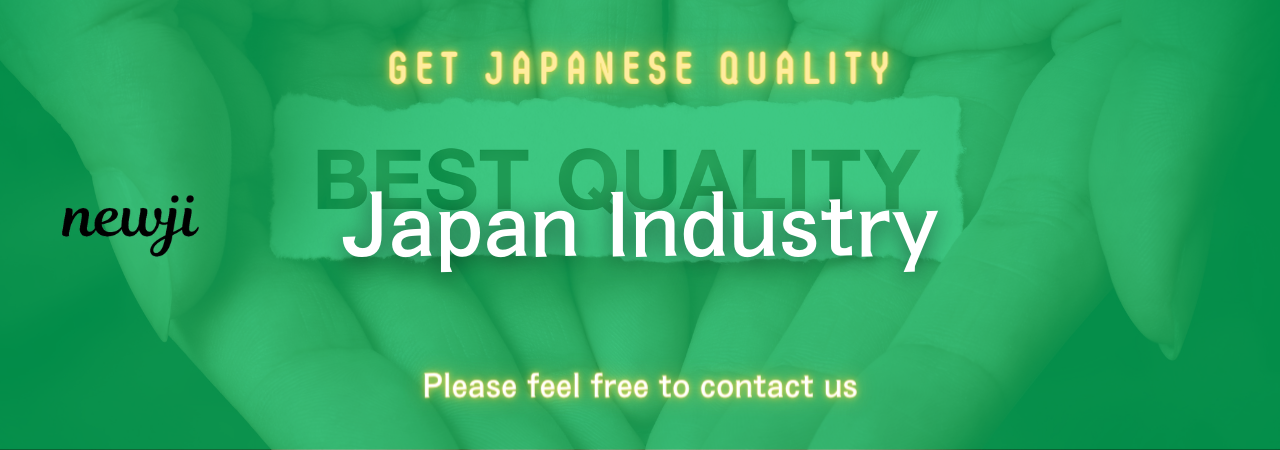
目次
Introduction to Machine Learning and Python
Machine learning is a fascinating branch of artificial intelligence that focuses on building systems that can learn from data and improve their performance over time without being explicitly programmed.
It’s a methodology that allows computers to derive patterns or trends from data, and make data-driven decisions.
Python, on the other hand, is a versatile programming language widely used for machine learning, thanks to its simplicity and the abundance of powerful libraries and frameworks.
Python’s rich ecosystem makes it an ideal language for beginners and experts alike.
By combining machine learning with Python, one can execute complex tasks, such as image classification, with greater ease and efficiency.
Understanding Image Classification
Image classification involves categorizing and labeling groups of pixels or vectors within an image based on specific rules.
It is a core task in computer vision and involves the process of assigning a label to an entire image or parts of an image.
For example, you might use image classification to identify objects in photos, such as distinguishing cats from dogs or apples from oranges.
This technique has various applications across different fields, including self-driving cars, medical diagnoses, monitoring security systems, and even social media.
With the advancement of deep learning and neural networks, image classification models have become incredibly accurate, performing tasks that were once thought impossible for machines.
Popular Machine Learning Models for Image Classification
1. Logistic Regression
Though traditionally used for binary classification, logistic regression can be extended to multi-class classification problems.
It operates by measuring the relationship between the categorical dependent variable and one or more independent variables.
2. Decision Trees
Decision trees are a non-parametric supervised learning algorithm used for classification and regression tasks.
They split the data into subsets through decisions based on the value of a feature.
The process continues until the algorithm finds a subset that is pure or meets a pre-defined stopping criterion.
3. Support Vector Machines (SVM)
Support vector machines are another powerful classification technique and are excellent for high-dimensional spaces.
They aim to find a hyperplane in an N-dimensional space that distinctly classifies the data points.
These models are particularly effective when the number of features is large compared to the sample size.
4. Convolutional Neural Networks (CNNs)
Convolutional Neural Networks (CNNs) are a class of deep learning models specifically designed for processing structured grid data such as images.
They make use of a specialized technique known as convolution, which is particularly effective at detecting patterns in visuals, such as edges and textures.
CNNs are the go-to model for image classification due to their ability to automatically and adaptively learn spatial hierarchies of features.
Building an Image Classification Model in Python
To build an image classification model in Python, you’ll typically need to follow these general steps:
1. Importing Libraries and Modules
Python offers a rich set of libraries that make building machine learning models simpler and more efficient.
For image classification, popular libraries include TensorFlow, Keras, PyTorch, and scikit-learn.
“`python
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
from tensorflow.keras import layers, models
“`
2. Loading and Preprocessing the Data
Image datasets usually come in large volumes and may require preprocessing.
This may include resizing images, normalizing pixel values, or augmenting data through transformations to boost the model’s robustness.
“`python
# Example preprocessing
def preprocess_images(image):
image = tf.image.resize(image, [128, 128])
image = image / 255.0
return image
“`
3. Building the Model
For this step, you’ll define the layers of your machine learning model.
In CNNs, layers like convolutional layers, pooling layers, and fully connected layers play a critical role.
“`python
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation=’relu’, input_shape=(128, 128, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation=’relu’),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(64, activation=’relu’),
layers.Dense(10, activation=’softmax’)
])
“`
4. Compiling the Model
In this phase, you select the optimizer, loss function, and metrics to be used during training.
“`python
model.compile(optimizer=’adam’,
loss=’sparse_categorical_crossentropy’,
metrics=[‘accuracy’])
“`
5. Training the Model
The model is now ready to be trained using the prepared dataset.
“`python
history = model.fit(training_dataset, epochs=10, validation_data=validation_dataset)
“`
6. Evaluating and Testing the Model
After the model has been trained, it’s crucial to evaluate its performance on unseen test data.
“`python
test_loss, test_acc = model.evaluate(test_dataset)
print(f’Test accuracy: {test_acc}’)
“`
Applications of Image Classification
Image classification models have numerous applications in the real world:
1. Healthcare
In the medical field, image classification assists in diagnosing diseases by classifying medical images such as X-rays and MRIs.
This can lead to early detection of conditions like cancer, potentially saving lives.
2. Autonomous Vehicles
Self-driving cars use image classification to identify road signs, pedestrians, and obstacles, ensuring safe navigation.
3. Social Media
Platforms like Facebook and Instagram use image classification to automatically tag people and categorize images based on content.
4. Retail
Retailers use image classification to track inventory and analyze customer preferences by evaluating product images.
Conclusion
Machine learning models, particularly those using Python, have revolutionized the field of image classification.
With ongoing advancements in machine learning algorithms and computational power, the accuracy and efficiency of these models will continue to improve, opening new avenues and applications across various domains.
Whether you’re just starting with machine learning or looking to implement image classification in a project, Python provides the tools necessary to transform your ideas into something tangible, powerful, and practical.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)