- お役立ち記事
- Smart contract implementation with Solidity
Smart contract implementation with Solidity
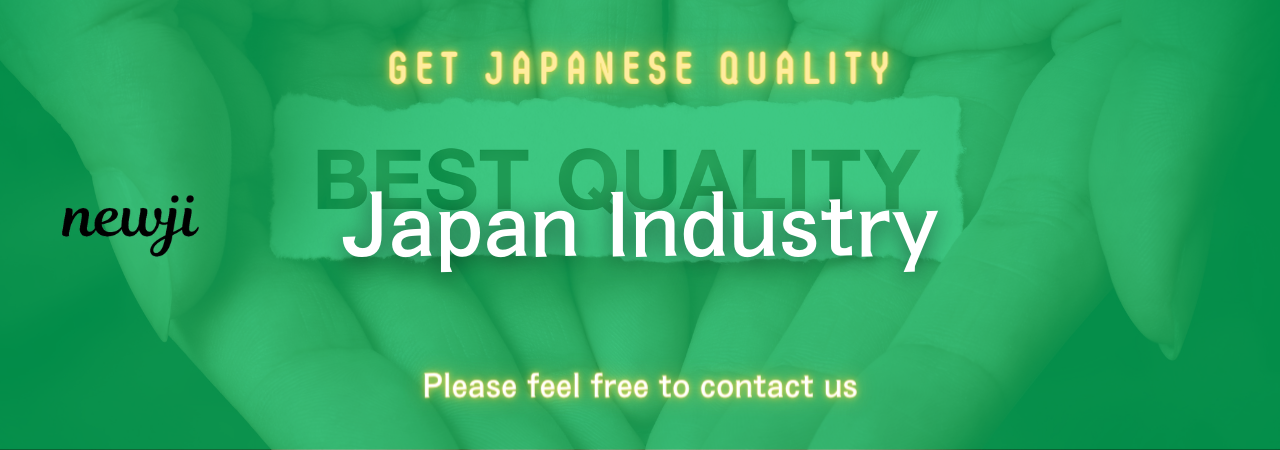
目次
Understanding Smart Contracts
Smart contracts are digital agreements that automatically execute and enforce themselves based on predefined criteria.
They operate on blockchain networks, ensuring transparency and security.
These contracts eliminate the need for intermediaries by enabling trust directly between parties involved in the transaction.
Smart contracts can manage and transfer assets, execute financial agreements, and more, making them a cornerstone of blockchain technology.
Solidity is a programming language used to write smart contracts, particularly on the Ethereum blockchain.
It’s similar to JavaScript, which makes it accessible for developers.
Solidity allows for the creation of complex contracts that can handle intricate transactions and business logic.
By using Solidity, developers can leverage Ethereum’s decentralized infrastructure to deploy smart contracts.
Getting Started with Solidity
Before writing your smart contract, you need to set up your development environment.
Perhaps the most user-friendly way to start is by using Remix, a web-based IDE for Solidity.
Remix allows you to write, test, and deploy smart contracts directly from your browser.
Firstly, visit the Remix website and create a new file with the `.sol` extension, which stands for Solidity.
Begin by specifying the version of Solidity you plan to use.
For instance, you can start with:
“`sol
pragma solidity ^0.8.0;
“`
This line defines that your contract should be compatible with Solidity version 0.8.0, but it will also work with any version that has a higher minor revision, provided it does not break backwards compatibility.
Creating a Basic Smart Contract
Let’s start by creating a simple contract.
A smart contract in Solidity is similar to a class in other programming languages.
Here’s a basic example:
“`sol
pragma solidity ^0.8.0;
contract SimpleStorage {
uint storedData;
function set(uint x) public {
storedData = x;
}
function get() public view returns (uint) {
return storedData;
}
}
“`
This smart contract, named `SimpleStorage`, can store and retrieve a single unsigned integer.
The `set` function allows users to store a number, and the `get` function returns that number.
Key Concepts in Solidity
Solidity contracts are composed of various elements and concepts.
Understanding these is crucial for writing effective smart contracts.
State Variables
State variables are permanently stored in contract storage.
In the example above, `storedData` is a state variable.
Functions
Functions can be defined within contracts to execute tasks.
They can modify the state or read from it.
Functions in Solidity can be assigned visibility levels, including `public`, `private`, `internal`, and `external`.
Modifiers
Modifiers in Solidity allow you to change the behavior of functions.
They can be used to add prerequisite conditions to a function.
For example:
“`sol
modifier onlyOwner() {
require(msg.sender == owner, “Not authorized”);
_;
}
“`
The `onlyOwner` modifier ensures that the function can only be executed by the contract owner.
Deploying Smart Contracts
Once your contract is written, you can compile and deploy it on a blockchain.
If you’re using Remix, you can compile your contract with the `Compile` tab.
After compiling, go to the `Deploy & Run` tab.
Here, select the environment you want to deploy to, such as a test network or the Ethereum mainnet.
You can choose a specific account to deploy from and click the `Deploy` button.
Interacting with Deployed Contracts
After deployment, you can interact with your contract through the Remix interface.
You will see buttons corresponding to the public functions of your contract.
For instance, in the `SimpleStorage` contract, you can click `set` to change the stored data and `get` to retrieve it.
Every interaction with the contract on the blockchain network incurs a gas fee, determined by the complexity of the function and the current network conditions.
Security Considerations
While Solidity is a powerful tool, smart contract developers must focus on security to prevent vulnerabilities.
Testing
Thoroughly test your contracts using unit tests.
Solidity supports libraries like Truffle and Hardhat for testing purposes.
Tests ensure that your contract functions as expected under various scenarios.
Code Audits
Conduct code audits to identify potential security risks.
Third-party audits can provide an additional layer of confidence.
Common Vulnerabilities
Be aware of common vulnerabilities such as Reentrancy, Integer Overflow/Underflow, and Timestamp Dependence.
Using secure libraries like OpenZeppelin’s Solidity Contracts can help mitigate some of these risks.
Conclusion
Implementing smart contracts with Solidity opens up myriad possibilities in decentralized applications.
The simplicity and accessibility of the Solidity language enable developers to create innovative solutions on the blockchain.
Understanding Solidity’s key concepts and maintaining a strong focus on security are vital steps in creating robust smart contracts.
As you embark on your journey to learn and develop with Solidity, remember that the blockchain landscape is constantly evolving.
Stay updated on best practices and advancements to build resilient applications.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)