- お役立ち記事
- Basics and implementation points of deep learning systems using Python
Basics and implementation points of deep learning systems using Python
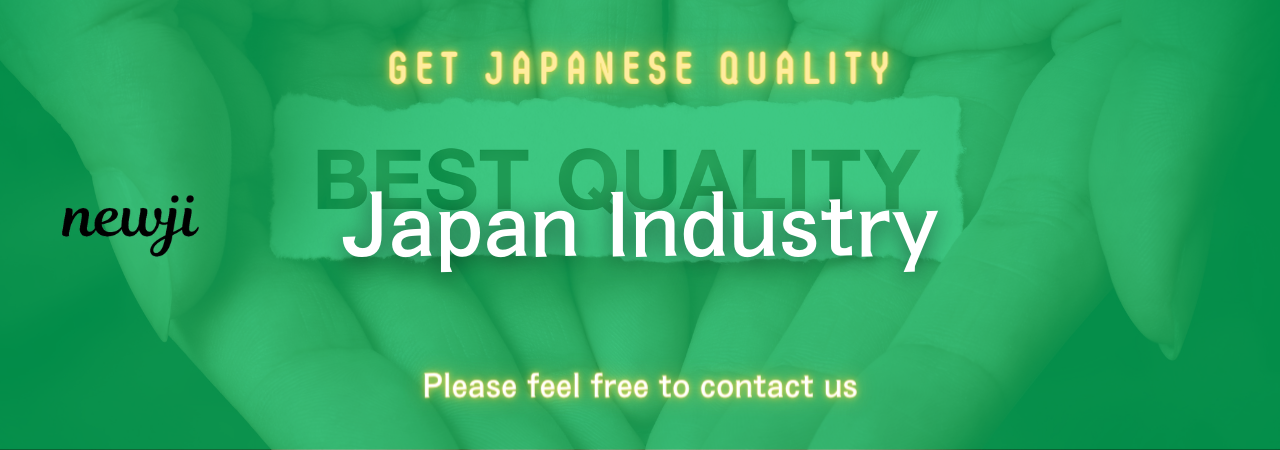
目次
Understanding Deep Learning
Deep learning is a subset of machine learning, which itself is a branch of artificial intelligence.
It uses neural networks with many layers—hence the term “deep”—to model and understand complex patterns in data.
These models are designed to mimic the human brain’s way of processing information, enabling machines to recognize speech, identify images, and even generate coherent text.
Python, with its vast ecosystem and libraries, has become a popular language for implementing deep learning systems.
In understanding how deep learning works, it’s essential to grasp the basics: neural networks, layers, and the training process.
What are Neural Networks?
Neural networks are the core architecture behind deep learning.
They consist of layers of nodes, also called neurons, which are interconnected.
Each node processes input data and passes it on to the next layer.
There are typically three types of layers in a neural network: input, hidden, and output layers.
The input layer receives the raw data, hidden layers transform and interpret this data, and the output layer delivers a prediction or result.
Neural networks adjust their internal parameters, known as weights, based on the error of their predictions through a process called training.
This adjustment is done using optimization techniques such as gradient descent, which aims to minimize the prediction error.
Layers in Deep Learning
In deep learning, the depth refers to the number of hidden layers in the network.
A simple neural network might have only one such layer, while deep neural networks can have dozens or even hundreds.
These multiple layers allow the network to learn abstract representations of data, which is crucial for handling complex tasks.
Layers can be of different types, serving different purposes.
Convolutional layers, for example, are used primarily in image processing to detect patterns like edges and textures.
Recurrent layers, on the other hand, are designed for sequence prediction tasks, such as language modeling or time series forecasting.
The Training Process
Training a deep learning model involves several steps.
First, data is fed into the network, and the model makes predictions.
The error or loss of these predictions is calculated using a loss function.
Then, backpropagation is used to adjust the network’s weights to reduce this loss.
The process involves iteratively fine-tuning the weights through multiple epochs, which are complete passes through the entire training dataset.
During each pass, the optimizer updates the weights designed to minimize the loss function.
To prevent overfitting, which occurs when the model learns the training data too well but fails to generalize to new data, techniques such as dropout, batch normalization, and regularization are employed.
Setting Up a Deep Learning Environment in Python
Python is the go-to language for deep learning due to its readability and the wealth of libraries available.
Setting up the environment properly is essential to get started with deep learning projects.
Required Libraries
Several key libraries are indispensable when building deep learning systems in Python:
1. **TensorFlow** and **Keras**: TensorFlow is a comprehensive, open-source library for numerical computation that makes building deep learning models straightforward.
Keras, which operates as a high-level API within TensorFlow, simplifies the process of building neural networks.
2. **PyTorch**: An alternative to TensorFlow, PyTorch is another powerful library for deep learning.
It’s favored for its dynamic computation graph, which allows for more flexible model building.
3. **NumPy**: This library is essential for numerical operations, providing support for arrays and matrices, which are frequently used in deep learning.
4. **Pandas**: A data manipulation library that is useful for handling datasets in preparation for training models.
5. **Scikit-learn**: This provides simple and efficient tools for data mining and data analysis, which are often used in conjunction with deep learning.
Building a Simple Deep Learning Model
Here, we’ll walk through the basic steps to build a simple deep learning model using Keras.
First, import the necessary libraries:
“`python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
“`
Next, prepare some sample data:
“`python
import numpy as np
# Sample data
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]]) # XOR problem
“`
Define the model architecture:
“`python
model = Sequential([
Dense(8, activation=’relu’, input_shape=(2,)),
Dense(1, activation=’sigmoid’)
])
“`
Compile the model:
“`python
model.compile(optimizer=’adam’, loss=’binary_crossentropy’, metrics=[‘accuracy’])
“`
Train the model:
“`python
model.fit(X, y, epochs=1000, verbose=0)
“`
And finally, evaluate the model:
“`python
loss, accuracy = model.evaluate(X, y)
print(f’Accuracy: {accuracy*100:.2f}%’)
“`
Points to Keep in Mind
– **Data Quality**: Ensure your data is clean and well-prepared, as poor data quality can doom the model’s performance from the start.
– **Model Complexity**: Adjust model complexity according to the dataset.
Too simple might underfit, while too complex might overfit.
– **Experimentation**: Experiment with different architectures, activations, and optimization techniques to see what works best for your specific problem.
– **Continuous Learning**: The field of deep learning is always evolving.
Stay updated with the latest research and practices.
By applying these principles and guidelines, building a deep learning system in Python can become a manageable task, even for those relatively new to the field.
This understanding builds a foundation to tackle more complex problems and explore the vast potential of deep learning technologies.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)