- お役立ち記事
- Basics and practice of text mining using Python
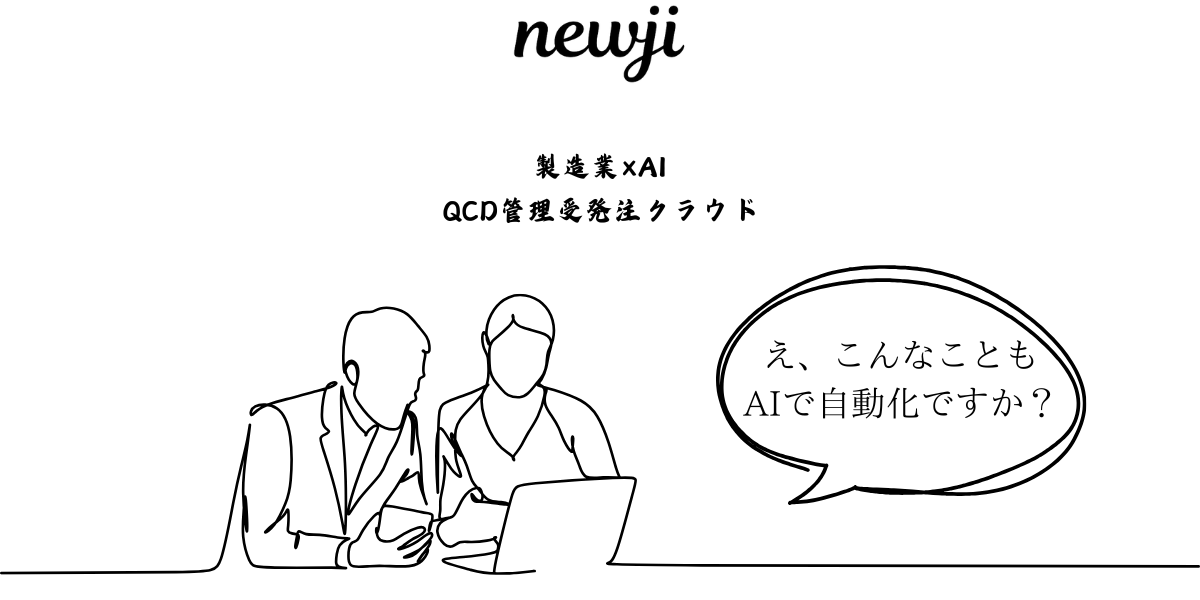
Basics and practice of text mining using Python
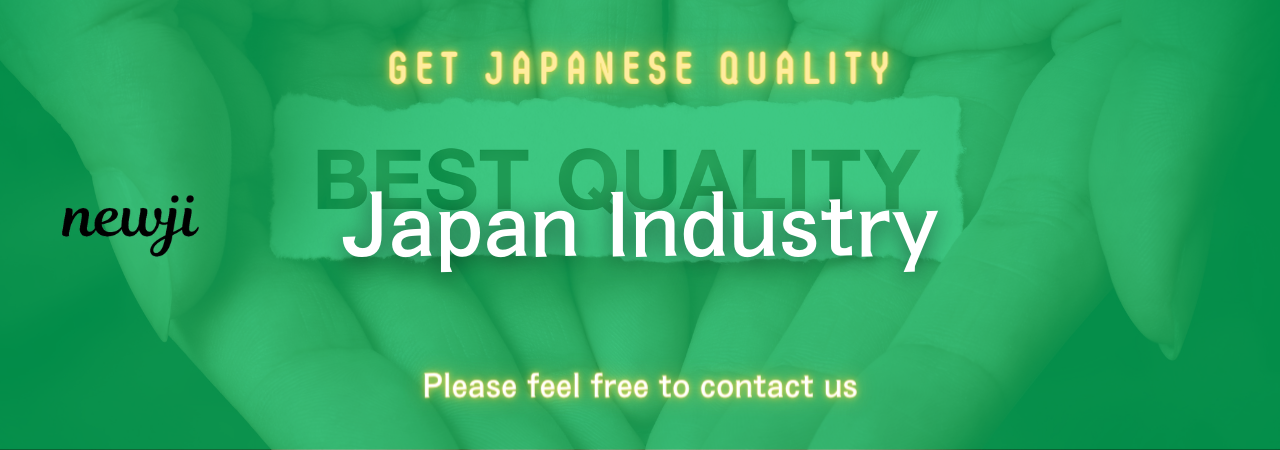
目次
Understanding Text Mining
Text mining is an advanced technological method used to extract valuable information from text data.
In simple terms, it involves analyzing and transforming text into manageable and structured data that computers can interpret.
The ultimate goal of text mining is to uncover hidden insights from text by identifying patterns and trends.
As the amount of text data available online continues to grow, the significance of text mining has never been more crucial.
Applications of Text Mining
Text mining can be applied to various fields and industries.
For instance, businesses use text mining to analyze customer feedback, reviews, and social media dialogue for market research.
In healthcare, text mining helps in processing clinical notes and patient records to improve medical diagnostics.
Another exciting application is in sentiment analysis, where businesses determine customer sentiment towards their products from large datasets.
Why Use Python for Text Mining?
Python is one of the most preferred programming languages for text mining.
This preference is due to Python’s simplicity, a vast array of libraries, and tools explicitly designed for text processing.
Moreover, Python’s growing community support makes it easier to find resources and seek help for text mining projects.
Popular Python Libraries for Text Mining
Several powerful libraries can be used for text mining in Python.
These include:
1. **Natural Language Toolkit (NLTK):** It is one of the most popular libraries, offering multiple text-processing libraries for classification, tokenization, stemming, tagging, and more.
2. **spaCy:** Known for its speed and efficiency, spaCy is used for large-scale data mining and complex natural language processing tasks.
3. **Pandas:** While primarily a data manipulation tool, Pandas becomes essential when you need to handle structured data alongside your text mining processes.
4. **Scikit-learn:** This library is perfect for text classification tasks and includes tools for model training and evaluation.
5. **Gensim:** Known for its topic modeling capabilities, Gensim handles large text data and creates word embeddings efficiently.
Basics of Text Mining Using Python
To get started with text mining using Python, you’ll need to familiarize yourself with essential preprocessing techniques.
These techniques prepare raw text data for analysis.
Tokenization
Tokenization is the process of breaking down text into smaller units called tokens.
Tokens can be words, phrases, or even sentences depending on the level of analysis required.
This process helps in understanding the structure and meaning of text data.
Example code using NLTK library:
“`python
import nltk
from nltk.tokenize import word_tokenize
text = “Text mining is fascinating!”
tokens = word_tokenize(text)
print(tokens)
“`
The output will be a list of tokens: `[‘Text’, ‘mining’, ‘is’, ‘fascinating’, ‘!’]`.
Removing Stopwords
Stopwords are frequently used words in a language that do not contribute much to the meaning of a sentence.
Words like ‘the’, ‘is’, ‘in’, ‘and’ are common English stopwords.
Removing them can improve the focus of your analysis.
Example code:
“`python
from nltk.corpus import stopwords
stop_words = set(stopwords.words(‘english’))
filtered_text = [word for word in tokens if word.lower() not in stop_words]
print(filtered_text)
“`
The expected output after filtering will be `[‘Text’, ‘mining’, ‘fascinating’, ‘!’]`.
Stemming and Lemmatization
These processes reduce words to their root forms.
Stemming involves trimming the end of words to achieve a common base form.
Lemmatization, meanwhile, uses dictionaries to reduce words to their base form but considers the context.
Example stem and lemma using NLTK:
“`python
from nltk.stem import PorterStemmer
from nltk.stem import WordNetLemmatizer
ps = PorterStemmer()
lemmatizer = WordNetLemmatizer()
word_stem = ps.stem(‘running’)
word_lemma = lemmatizer.lemmatize(‘running’, pos=’v’)
print(word_stem, word_lemma)
“`
Output: `run run`
Part-of-Speech Tagging (POS)
POS tagging assigns a part of speech to each word, such as noun, verb, adjective, etc., facilitating a deeper understanding of the text’s context.
“`python
tagged_text = nltk.pos_tag(tokens)
print(tagged_text)
“`
The output will be a list of tuples with words and their POS, e.g., `(‘Text’, ‘NN’), (‘mining’, ‘VBG’)`.
Advanced Text Mining Techniques in Python
Once you are comfortable with the basics, you can delve into more advanced text mining techniques.
Text Classification
Text classification involves categorizing text into predefined groups.
For example, spam detection is a text classification task.
“`python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.pipeline import make_pipeline
model = make_pipeline(TfidfVectorizer(), MultinomialNB())
model.fit(train_data, train_labels)
predicted_labels = model.predict(test_data)
“`
Sentiment Analysis
Sentiment analysis aims to extract, quantify, and study effective states and subjective information from text.
It is commonly used in social media monitoring.
“`python
from textblob import TextBlob
text = “I love learning about text mining!”
analysis = TextBlob(text)
print(analysis.sentiment)
“`
This will output a sentiment score that shows text’s polarity and subjectivity.
Summary
Text mining with Python is an invaluable skill that allows you to mine insights from large volumes of unstructured text data.
Equipped with Python’s diverse libraries and tools, you can efficiently tokenize text, remove irrelevant data, and utilize advanced techniques like sentiment analysis and classification.
As the digital world expands, so does the need for professionals well-versed in text mining methodologies.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)