- お役立ち記事
- Basics of image processing using OpenCV and application to image analysis
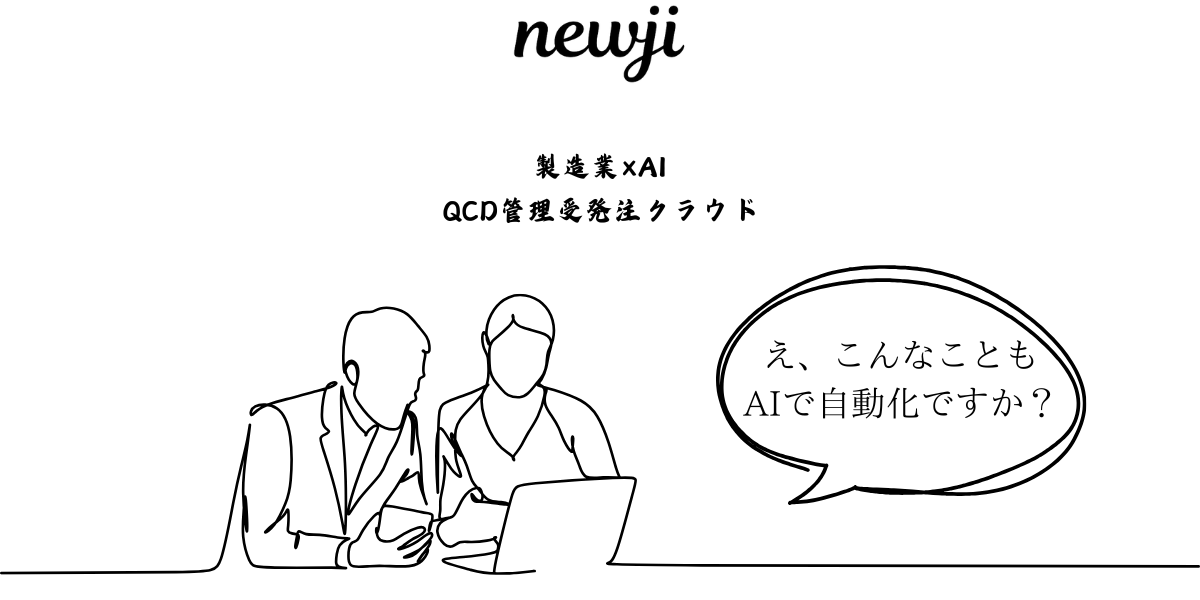
Basics of image processing using OpenCV and application to image analysis
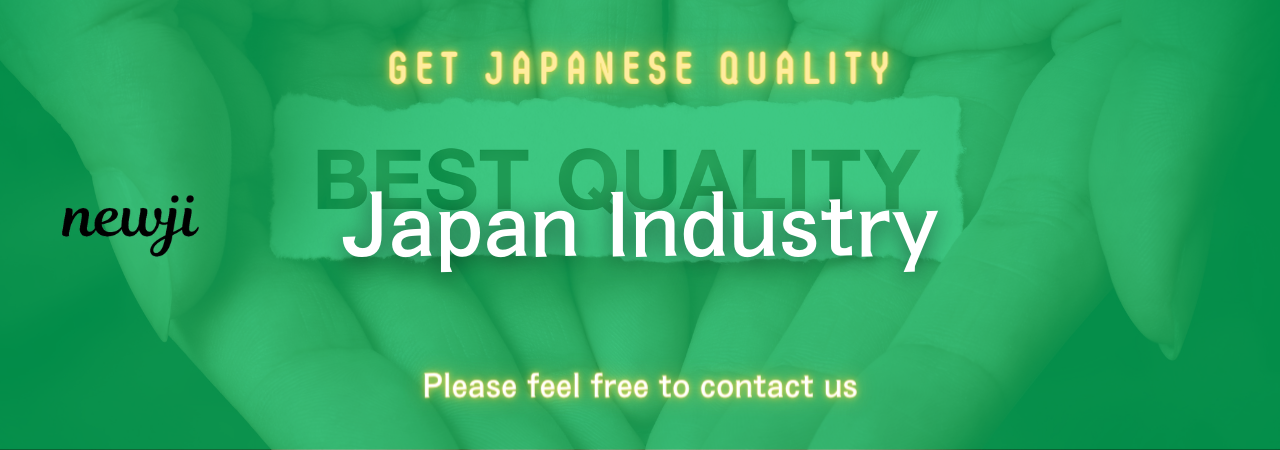
目次
Understanding Image Processing and OpenCV
Image processing is a method used to perform operations on images to enhance them or to extract useful information.
It’s a type of signal processing where the input is an image, and the output might be either an image or a set of characteristics related to the image.
One of the most popular libraries for image processing is OpenCV, which stands for Open Source Computer Vision Library.
Created initially by Intel, OpenCV is a powerful tool that offers a variety of functions for both image and video analysis.
What is OpenCV?
OpenCV is an open-source library specifically designed for computational efficiency and includes a wide range of algorithms for image processing and analysis.
It’s extensively used in real-time applications, such as facial recognition systems, motion tracking, and even robotics.
OpenCV supports a range of programming languages including Python, C++, and Java, making it accessible to many developers.
Through OpenCV, users can perform complex operations such as edge detection, image transformation, filtering, and more, with a few lines of code.
Setting Up OpenCV
Before diving into image processing operations, it’s important to set up OpenCV in your working environment.
For Python users, the easiest way is to use pip, a package installer for Python:
“`bash
pip install opencv-python
pip install opencv-python-headless
“`
For C++ users, OpenCV can be compiled from the source or installed through package managers like apt for Ubuntu users.
Once OpenCV is installed, it is very easy to start using it by importing it with the alias typically used in Python:
“`python
import cv2
“`
Basic Image Operations
Once OpenCV is installed and imported, you can start performing basic image operations.
For beginners, understanding how to read, display, and write images is crucial.
Reading and Displaying Images
To read and display an image in Python using OpenCV, you use the `cv2.imread()` and `cv2.imshow()` functions:
“`python
# Load an image
image = cv2.imread(‘path_to_image.jpg’)
# Display the image
cv2.imshow(‘Image’, image)
# Wait until a key is pressed
cv2.waitKey(0)
# Destroy all windows
cv2.destroyAllWindows()
“`
The `cv2.imread()` function reads the image from the specified path, and `cv2.imshow()` displays it in a window.
The window will remain open until a key is pressed, due to `cv2.waitKey(0)`.
Writing Images
To save an image, you use the `cv2.imwrite()` function.
This function writes the image to disk in the designated format:
“`python
# Save the image as ‘output.jpg’
cv2.imwrite(‘output.jpg’, image)
“`
Basic Image Processing Techniques
Now, let’s explore some fundamental image processing techniques you can perform with OpenCV.
Converting Color Spaces
An essential technique is color space conversion.
For example, you might want to convert an image from BGR (Blue, Green, Red) to grayscale or from BGR to HSV (Hue, Saturation, Value):
“`python
# Convert to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Convert to HSV
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
“`
Image Smoothing
Image smoothing, often known as blurring, is an important preprocessing step to reduce noise.
OpenCV offers several methods like Gaussian Blur, Median Blur, and more:
“`python
# Apply Gaussian Blur
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
“`
Gaussian Blur is widely used because it effectively smooths the image by averaging the pixel values in the kernel area.
Edge Detection
Another crucial operation is edge detection, which is commonly used to identify the boundaries within images.
The Canny Edge Detector is one of the most popular methods:
“`python
# Detect edges
edges = cv2.Canny(image, 100, 200)
“`
This function detects edges by looking for areas with strong intensity gradients.
Application of Image Processing
Understanding these basic operations is vital for more complex applications, such as image analysis.
Image analysis often involves examining images to extract meaningful information, like detecting objects or recognizing patterns.
Object Detection
In object detection tasks, image processing helps isolate objects of interest from the background.
By applying techniques like color space conversion, thresholding, and contour detection, you can identify objects efficiently.
For example, converting an image to grayscale, applying thresholding, and then finding contours can help detect specific shapes.
Image Segmentation
Image segmentation partitions an image into multiple segments or regions.
It simplifies the representation of an image to make it more meaningful and easier to analyze.
OpenCV supports several methods for image segmentation, such as Watershed, GrabCut, and K-means clustering.
Conclusion
OpenCV is a robust and versatile tool that is fundamental in the field of image processing and analysis.
From basic operations like reading and displaying images to complex tasks like edge detection and object recognition, OpenCV offers capabilities that cater to both beginners and advanced users.
Understanding these basics is the first step toward mastering image analysis, leading to the creation of intelligent systems that can interpret and respond to visual data.
Experimenting with various functions and techniques in OpenCV will help unlock the potential it offers for innovative applications across many domains.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)