- お役立ち記事
- Anomaly detection method and implementation programming using Python
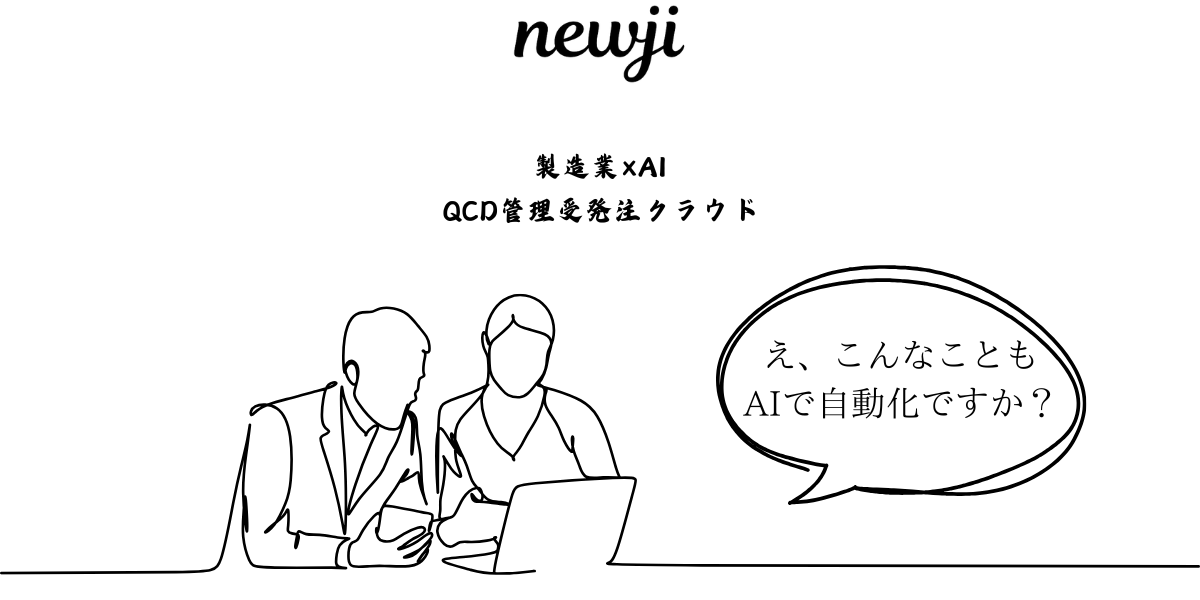
Anomaly detection method and implementation programming using Python
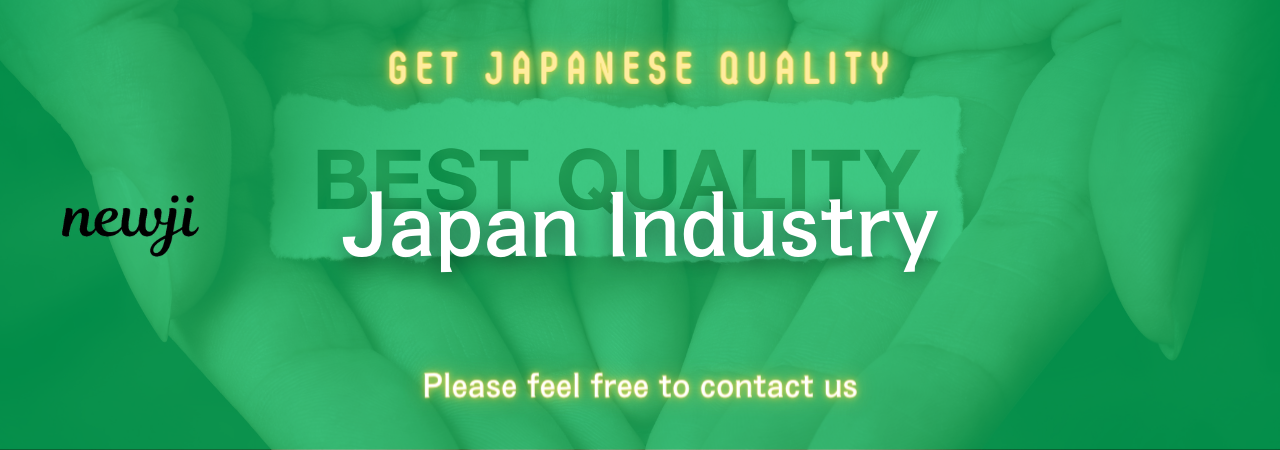
目次
Understanding Anomaly Detection
Anomaly detection is a crucial concept in various fields such as finance, healthcare, and cybersecurity.
It involves identifying patterns in data that deviate from expected behavior.
These unusual patterns could indicate potential issues or significant occurrences that require further investigation.
For instance, in banking, anomaly detection could help spot fraudulent transactions.
In medical settings, it might highlight abnormal test results.
Understanding and implementing anomaly detection can significantly enhance decision-making processes and improve overall operational efficiency.
Why Use Python for Anomaly Detection?
Python is a powerful programming language widely used for data analysis and machine learning tasks, making it an excellent choice for anomaly detection.
Its rich library ecosystem, including NumPy, Pandas, Scikit-learn, and TensorFlow, provides robust tools for processing data and building efficient models.
Python’s simple syntax and readability further facilitate the implementation of complex algorithms.
Through its vast resources, Python allows both beginners and experienced developers to construct sophisticated anomaly detection systems with relative ease.
Types of Anomaly Detection Techniques
Anomaly detection techniques can be broadly categorized into three types: statistical, machine learning, and deep learning-based methods.
Statistical Methods
Statistical methods are among the most straightforward approaches to anomaly detection.
They rely on the assumption that data follows a certain distribution, such as Gaussian or normal distribution.
Outliers are identified based on a specific threshold for statistical measures like mean or variance.
These methods are quick to implement and computationally efficient but may not work well with complex or high-dimensional datasets.
Machine Learning Methods
Machine learning methods offer more flexibility and accuracy compared to statistical approaches.
These techniques include clustering, classification, and ensemble methods.
Clustering algorithms like k-means can group data points and identify anomalies as those that do not fit well in any cluster.
Classification methods involve training a model to distinguish between normal and anomalous instances.
Ensemble methods combine multiple models to enhance prediction accuracy, making them highly effective for complex anomaly detection tasks.
Deep Learning Methods
Deep learning methods use neural networks to model complex patterns in data.
They are particularly effective for large datasets with intricate structures.
Autoencoders, for example, are neural networks trained to reconstruct input data, and deviations in reconstruction error can indicate anomalies.
While deep learning methods require significant computational resources and expertise, they offer superior performance in identifying anomalies in complex datasets.
Implementing Anomaly Detection in Python
Let’s explore how to implement a basic anomaly detection system using Python.
1. Setting Up the Environment
To get started, you’ll need to install a few Python libraries.
Ensure you have Python and pip (Python package installer) setup.
You can use a virtual environment to manage dependencies:
“`python
pip install numpy pandas scikit-learn matplotlib
“`
2. Loading and Preparing Data
Begin by loading your dataset using Pandas:
“`python
import pandas as pd
data = pd.read_csv(‘your_dataset.csv’)
“`
Inspect the data to understand its structure and identify any necessary preprocessing:
“`python
print(data.head())
print(data.info())
“`
3. Example: Using Z-Score for Anomaly Detection
The Z-score method is a simple statistical technique for anomaly detection.
A Z-score indicates how many standard deviations an element is from the mean:
“`python
import numpy as np
mean = data[‘your_column’].mean()
std = data[‘your_column’].std()
threshold = 3
data[‘z_score’] = (data[‘your_column’] – mean) / std
anomalies = data[data[‘z_score’] > threshold]
“`
This method identifies data points that deviate significantly from the mean.
4. Example: Using Isolation Forest in Scikit-learn
Isolation Forest is an effective ensemble method provided by Scikit-learn for anomaly detection:
“`python
from sklearn.ensemble import IsolationForest
model = IsolationForest(contamination=0.05)
model.fit(data[[‘your_column’]])
data[‘anomaly’] = model.predict(data[[‘your_column’]])
anomalies = data[data[‘anomaly’] == -1]
“`
This approach involves training an ensemble of isolation trees to isolate anomalies efficiently.
Visualizing Anomalies
Visualization can enhance the interpretation of anomaly detection results:
“`python
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 6))
plt.plot(data[‘your_column’], label=’Data’)
plt.scatter(anomalies.index, anomalies[‘your_column’], color=’red’, label=’Anomalies’)
plt.title(‘Anomaly Detection’)
plt.xlabel(‘Index’)
plt.ylabel(‘Value’)
plt.legend()
plt.show()
“`
Visualizing anomalies helps in understanding the data distribution and the detected outliers, providing valuable insights for further analysis.
Challenges and Considerations
Implementing anomaly detection comes with its own set of challenges.
Choosing an appropriate method depends on your dataset’s nature and complexity.
It’s crucial to handle data preprocessing carefully, like dealing with missing values and scaling features.
Moreover, setting the correct threshold for anomaly identification is key, as it varies based on the method and the specific application context.
Regularly evaluating your model’s performance and adjusting parameters or methods as necessary ensures accurate and reliable anomaly detection results.
Harnessing the power of Python and its comprehensive libraries, one can effectively implement and refine anomaly detection systems, aiding in uncovering valuable insights concealed within data.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)