- お役立ち記事
- Fundamentals of deep learning with Python and PyTorch and applications to data analysis
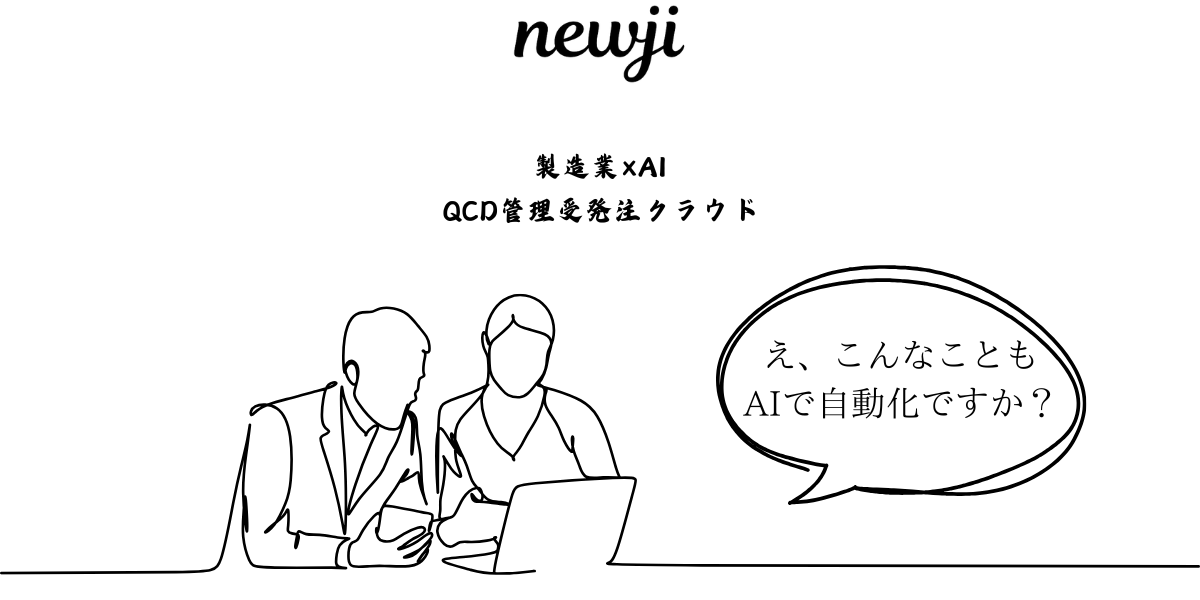
Fundamentals of deep learning with Python and PyTorch and applications to data analysis
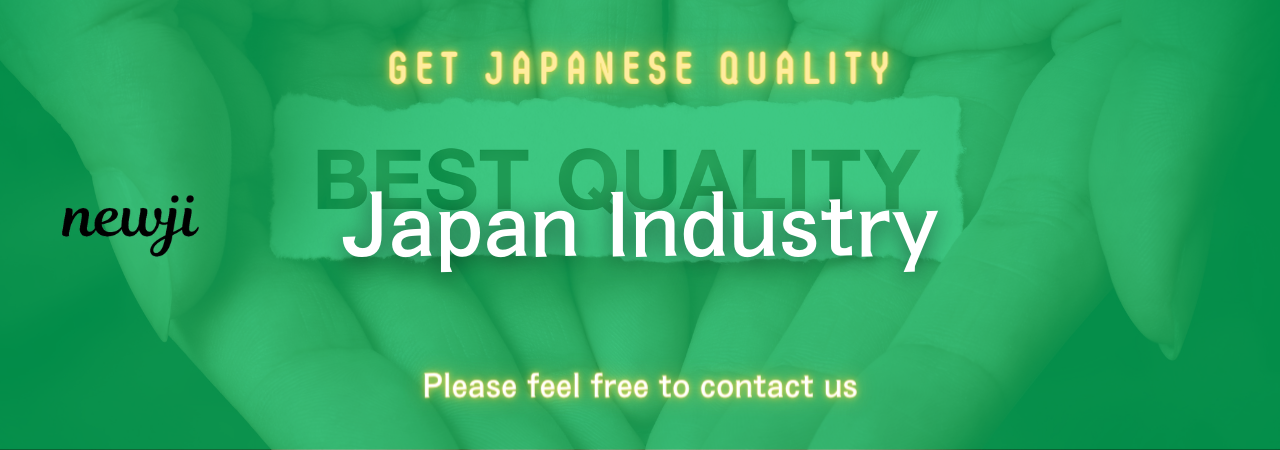
目次
Introduction to Deep Learning
Deep learning is a subset of artificial intelligence (AI) that focuses on algorithms inspired by the structure and function of the brain, known as neural networks.
These algorithms can learn from vast amounts of data and make decisions or predictions based on that data.
Deep learning has gained significant attention due to its ability to achieve human-like accuracy in tasks such as image and speech recognition.
Understanding Python and PyTorch
Python is a popular programming language known for its readability and versatility.
It is widely used in data science, machine learning, and deep learning applications.
One of the primary reasons for Python’s popularity in these fields is its vast ecosystem of libraries and frameworks, which makes complex computations more manageable and efficient.
PyTorch is an open-source machine learning library developed by Facebook’s AI Research lab.
It provides flexibility and speed, which allows developers to create deep learning models with ease.
Its dynamic computation graph and robust community support make it a preferred choice for researchers and practitioners alike.
Building Blocks of Deep Learning
Deep learning models are built using layers of artificial neurons.
Each layer performs computations on the input data and passes the output to the next layer.
The main components of these models include:
1. **Neurons:** The fundamental units that process inputs and produce outputs. Each neuron performs a weighted sum of its inputs, applies an activation function, and outputs a signal.
2. **Layers:** Organized collections of neurons. Deep learning models consist of multiple layers, including input, hidden, and output layers.
3. **Activation functions:** Functions applied to neurons to introduce non-linearity. Popular activation functions include ReLU, Sigmoid, and Tanh.
4. **Loss functions:** Calculate the error between the predicted and actual outputs. The aim is to minimize this error during training.
5. **Optimization algorithms:** Methods used to update model parameters to minimize the loss function. The most commonly used algorithm is Stochastic Gradient Descent (SGD) and its variants, like Adam and RMSProp.
Implementing Deep Learning with PyTorch
Let’s dive into how deep learning can be implemented using PyTorch, focusing on a simple data analysis task.
Step 1: Setting Up the Environment
Before we start coding, ensure you have the necessary software installed on your system.
You’ll need Python, PyTorch, and some additional libraries like NumPy, which are used for numerical operations.
Step 2: Preparing the Data
Data is the lifeblood of any deep learning model.
Begin by collecting and preprocessing the data needed for your analysis.
Transform the data into tensors, which are the fundamental data structure in PyTorch, similar to arrays in NumPy.
Step 3: Defining the Model
Define a neural network model using PyTorch’s modules.
You’ll create a class that inherits from `torch.nn.Module`, which encapsulates all the layers of your network.
“`python
import torch
import torch.nn as nn
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(in_features=4, out_features=3)
self.fc2 = nn.Linear(in_features=3, out_features=2)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.softmax(self.fc2(x), dim=1)
return x
“`
In this simple example, `SimpleNet` consists of two fully connected layers with an activation function in between.
Step 4: Defining the Loss and Optimizer
Choose a loss function and an optimizer for training your model.
For a classification task, you might use cross-entropy loss coupled with an optimization algorithm like Adam.
“`python
loss_fn = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(SimpleNet.parameters(), lr=0.001)
“`
Step 5: Training the Model
Train your model by feeding it with data, computing the loss, and updating the weights using backpropagation.
“`python
for epoch in range(num_epochs):
for inputs, labels in data_loader:
# Forward pass
outputs = SimpleNet(inputs)
loss = loss_fn(outputs, labels)
# Backward pass and optimization
optimizer.zero_grad()
loss.backward()
optimizer.step()
“`
The model updates its weight parameters using the optimizer, reducing the loss over time.
Applications to Data Analysis
Deep learning’s abilities extend to various domains in data analysis.
Some common applications include:
1. **Image Recognition:** Deep learning models can interpret and label objects within images with high accuracy.
2. **Natural Language Processing (NLP):** Models can understand and generate human-like text, enabling chatbots and other language-based applications.
3. **Forecasting and Predictive Analytics:** Deep learning detects patterns and predicts future trends in data, such as stock prices or weather forecasts.
4. **Anomaly Detection:** Identifies unusual patterns or outliers in data, used in fraud detection or monitoring manufacturing processes.
Conclusion
Deep learning, when combined with tools like Python and PyTorch, offers powerful capabilities for data analysis and beyond.
By understanding the fundamental concepts and following structured implementation steps, you can unlock insights from various forms of data.
The applications of deep learning continue to expand, making it an exciting field to explore and innovate within.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)