- お役立ち記事
- Basics and practice of Python programming and how to utilize libraries
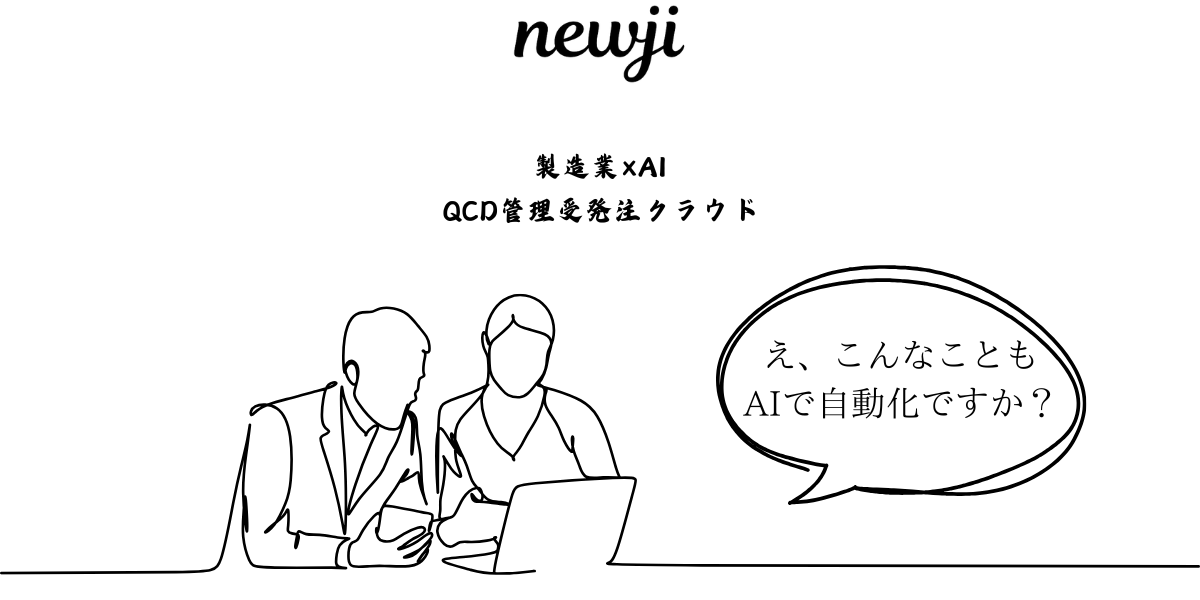
Basics and practice of Python programming and how to utilize libraries
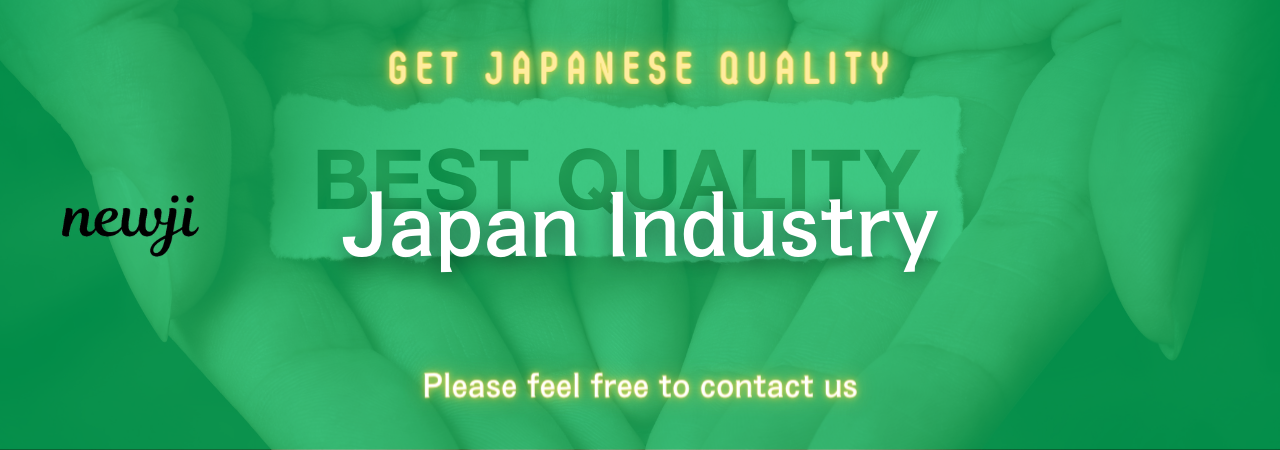
目次
Understanding the Basics of Python Programming
Python is a powerful and versatile programming language that’s easy for beginners to grasp yet robust enough for experienced developers to tackle complex tasks.
It’s widely used in web development, data science, artificial intelligence, machine learning, automation, and more.
But before diving into how Python is used and its libraries, it’s important to understand the basics of Python programming.
Python is known for its readability and simplicity, writing code in a way that’s clear and understandable.
Its syntax is designed to be intuitive, which means it looks a lot like regular English.
This makes it an excellent choice for both new programmers and seasoned developers.
One of the core concepts in Python is understanding data types and variables.
Python supports several data types, including integers (whole numbers), floats (decimal points), strings (text), and booleans (True or False values).
Variables are used to store these data types, making it easy to manipulate and utilize them within your programs.
For example, you can create a variable in Python with just a simple statement:
“`python
age = 10
name = “Alice”
is_student = True
“`
Here, `age` is an integer, `name` is a string, and `is_student` is a boolean.
Getting Started with Python Programming
The first step to practicing Python is installing it on your computer system.
Python and its library can be installed from the official website at python.org.
After installation, you can start coding using an Integrated Development Environment (IDE) like PyCharm, Visual Studio Code, or even a simple text editor followed by running your scripts via the command line or terminal.
When programming in Python, the most basic tool is the print statement, which allows the output of text or variables to the screen.
Here is a simple example:
“`python
print(“Hello, World!”)
“`
Running this line of code will display “Hello, World!” on your screen.
Control Flow: Conditionals and Loops
The flow of a Python program is controlled by conditionals and loops.
Conditionals use `if`, `else`, and `elif` statements to execute different pieces of code based on conditions.
For instance:
“`python
age = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("You just became an adult!")
else:
print("You are an adult.")
```
Python loops, such as `for` and `while` loops, help repeat actions a certain number of times or until a condition is met.
Here's an example using a `for` loop:
```python
for i in range(5):
print("This is loop iteration", i)
```
Utilizing Libraries in Python Programming
One of Python’s greatest strengths is its extensive library ecosystem, where developers can find modules or packages to speed up development tasks.
These libraries can be easily installed and used to extend Python’s capabilities.
Standard Library
The Python Standard Library is included with every Python installation, providing useful modules for various tasks like reading and writing files, managing dates and times, mathematics, and more.
For instance, you can use the `math` module to perform mathematical operations:
“`python
import math
print(math.sqrt(16)) # Output will be 4.0
“`
Popular Third-Party Libraries
Python’s popularity has led to the development of countless third-party libraries, with some being particularly notable:
– **NumPy**: A library for numerical computing that includes comprehensive mathematical functions and supports large multi-dimensional arrays and matrices.
– **Pandas**: Provides easy-to-use data structures and data analysis tools for data manipulation and analysis.
– **Matplotlib**: A plotting library to create static, interactive, and animated visualizations in Python.
– **Requests**: A library for simplifying HTTP requests, allowing developers to easily connect to web resources.
– **TensorFlow**: An open-source library for machine learning and artificial intelligence tasks.
To use these libraries, they need to be installed using Python’s package manager, pip.
For example:
“`shell
pip install numpy
“`
Practical Python Applications
Python’s flexibility allows it to be applied to various practical applications that are both useful and fun.
Here are some areas where Python makes a significant impact:
Web Development
Python frameworks like Django and Flask enable developers to build dynamic websites efficiently.
These frameworks handle many of the intricacies involved in web development, such as database interactions, and offer tools for crafting robust web applications.
Data Analysis and Visualization
Data analysts leverage Python’s potential in handling data to make sense of large datasets.
With libraries like Pandas for data manipulation and Matplotlib or Seaborn for visualization, Python helps transform raw data into insightful graphs and charts.
You can create a simple line graph using Matplotlib:
“`python
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.ylabel(‘Some Numbers’)
plt.show()
“`
Automation and Scripting
Python scripts can automate repetitive tasks, saving time and minimizing errors.
This includes everything from organizing files to managing spreadsheets, pulling data from websites, and more.
Python’s simplicity and rich ecosystem of libraries make it a prime choice for beginners and experts in the tech field.
By understanding its basic syntax and leveraging its powerful libraries, you can harness Python’s full potential to create impactful applications and solutions.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)