- お役立ち記事
- A practical course on image processing and machine learning modeling using Python
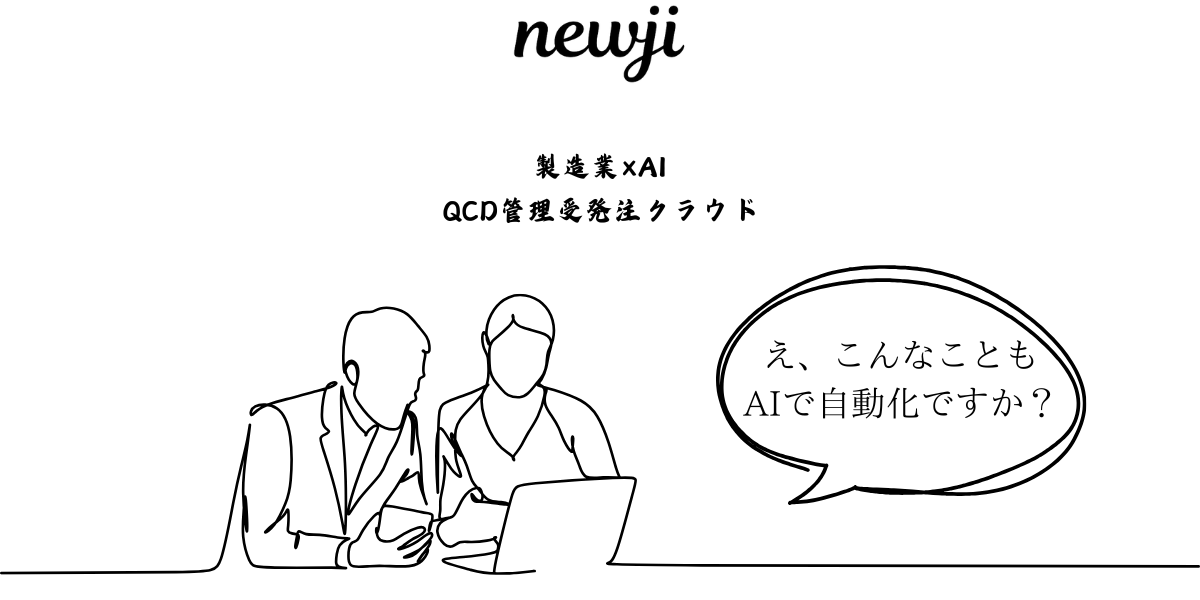
A practical course on image processing and machine learning modeling using Python
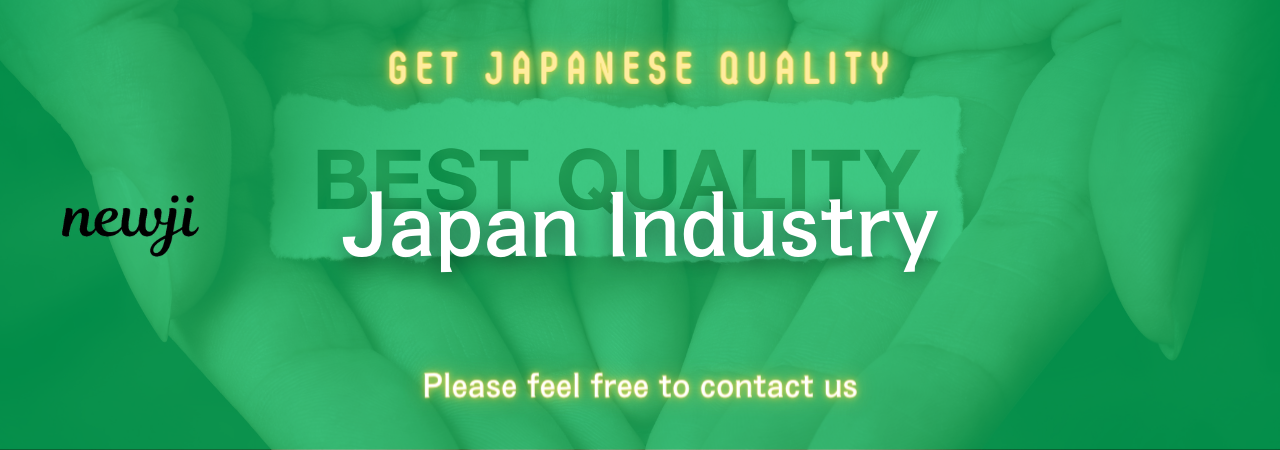
目次
Introduction to Image Processing and Machine Learning
Python has become a leading programming language in the fields of image processing and machine learning.
Whether you are a beginner or an advanced programmer, understanding how to manipulate images and implement machine learning models in Python can be incredibly beneficial.
This practical course aims to introduce you to the key concepts and tools needed to get started with image processing and machine learning using Python.
Why Use Python for Image Processing and Machine Learning?
Python’s popularity can be attributed to its simplicity and the wide range of libraries it offers.
For image processing, libraries like OpenCV and Pillow provide powerful tools for manipulating images effortlessly.
Meanwhile, machine learning frameworks such as TensorFlow, Keras, and scikit-learn simplify the process of building and training models.
The integration of these libraries with Python makes it a versatile language for solving complex problems in image processing and machine learning.
The community support and availability of extensive documentation make Python a preferred choice for both beginners and experts in the field.
Setting Up Your Environment
Before diving into image processing and machine learning, you need to set up a Python environment.
The Anaconda distribution is highly recommended as it includes essential packages and simplifies the installation process of additional libraries.
Once Anaconda is installed, use the following command in the Anaconda Prompt to create a virtual environment:
“`
conda create -n image_ml python=3.9
“`
After creating the environment, activate it with:
“`
conda activate image_ml
“`
You can then install necessary libraries using pip or conda:
“`
pip install opencv-python pillow tensorflow keras scikit-learn
“`
Basic Image Processing with Python
Image processing involves enhancing, transforming, and extracting information from images.
Let’s cover some basic techniques using OpenCV and Pillow.
Loading and Displaying an Image
Begin by loading an image using OpenCV:
“`python
import cv2
# Load an image
image = cv2.imread(‘path_to_image.jpg’)
# Display the image
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Alternatively, you can use Pillow:
“`python
from PIL import Image
# Load an image
image = Image.open(‘path_to_image.jpg’)
# Display the image
image.show()
“`
Basic Image Transformations
Common transformations include resizing, rotating, and cropping.
To resize using OpenCV:
“`python
resized_image = cv2.resize(image, (new_width, new_height))
“`
To rotate:
“`python
(h, w) = image.shape[:2]
center = (w // 2, h // 2)
matrix = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated_image = cv2.warpAffine(image, matrix, (w, h))
“`
With Pillow, you can resize and rotate as follows:
“`python
# Resize
resized_image = image.resize((new_width, new_height))
# Rotate
rotated_image = image.rotate(angle)
“`
Introduction to Machine Learning
Machine learning involves training computers to recognize patterns from data.
In the context of image processing, this often means training models to identify features in images.
Building a Simple Classifier
Let’s build a simple image classifier using scikit-learn.
Imagine you have two categories: cats and dogs.
Start by loading the dataset and dividing it into training and testing sets:
“`python
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_files
# Load dataset
data = load_files(‘path_to_dataset’, shuffle=True)
X = data[‘filenames’]
y = data[‘target’]
# Split into training and testing
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
“`
Next, pre-process the images and convert them into a numerical format suitable for training:
“`python
from skimage.io import imread
from skimage.transform import resize
import numpy as np
def preprocess_images(images):
processed_images = [resize(imread(img), (150, 150)) for img in images]
return np.array(processed_images)
X_train_processed = preprocess_images(X_train)
X_test_processed = preprocess_images(X_test)
“`
Choose a model, such as a simple Neural Network or Support Vector Machine (SVM):
“`python
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
# Initialize the model
model = SVC(kernel=’linear’)
# Fit the model
model.fit(X_train_processed.reshape(X_train_processed.shape[0], -1), y_train)
# Predict and evaluate
y_pred = model.predict(X_test_processed.reshape(X_test_processed.shape[0], -1))
accuracy = accuracy_score(y_test, y_pred)
print(f’Accuracy: {accuracy}’)
“`
Expanding Your Skills
The example above is just the beginning.
Once comfortable with basic processing and model building, explore deep learning with frameworks like TensorFlow and Keras.
They provide more advanced techniques for image classification, object detection, and segmentation.
Working with Neural Networks
Deep learning models such as Convolutional Neural Networks (CNNs) excel at image-related tasks.
These networks mimic the human brain’s structure and detect intricate patterns in image data.
Start by experimenting with pre-trained models available in frameworks like Keras.
Loading and fine-tuning these models often yields impressive results even with limited resources and data.
Conclusion
By following this practical course, you’ve taken the first steps in leveraging Python for image processing and machine learning tasks.
The combination of robust libraries and a supportive community makes Python a compelling choice for working with images and building intelligent models.
As you continue to practice and explore, you’ll find more creative and innovative ways to apply these techniques to real-world problems.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)