- お役立ち記事
- Anomaly detection method and implementation programming using Python
Anomaly detection method and implementation programming using Python
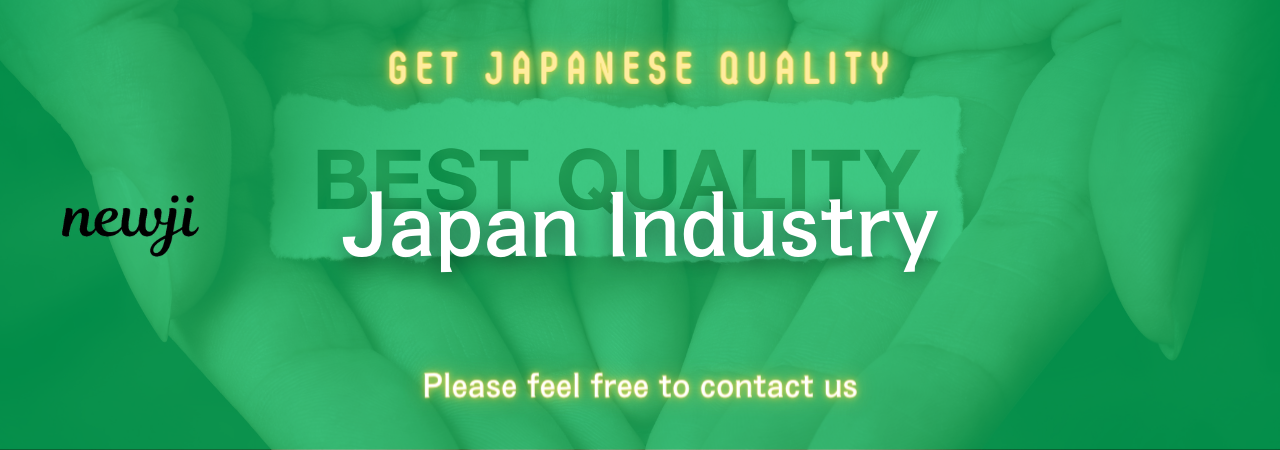
目次
Understanding Anomaly Detection
Anomaly detection is a crucial technique used to identify unusual patterns that do not conform to expected behavior, particularly in data analysis.
Though the term might sound complex, think of anomaly detection as a way to find oddities or surprises in a dataset.
These anomalies could indicate critical issues such as fraud, network intrusions, or faulty systems, making this technique invaluable across various fields.
In general, anomaly detection involves the identification of rare items, events, or observations which raise suspicions by differing significantly from the majority of the data.
These outliers can manifest due to variability in data or may indicate something noteworthy.
Why Use Python for Anomaly Detection?
Python is a versatile, high-level programming language known for its ease of use and readability.
It is a popular tool for data analysis and machine learning, thanks to its rich ecosystem of libraries and frameworks.
Python provides powerful tools for anomaly detection, such as Scikit-learn, TensorFlow, and PyOD, which are specifically designed for machine learning and statistical analysis.
Python’s extensive community support and comprehensive documentation make it an ideal choice for both beginners and experienced programmers attempting anomaly detection projects.
The rich variety of pre-built algorithms and tools simplify the implementation and enable a more efficient workflow.
Common Anomaly Detection Techniques
There are several anomaly detection techniques that you can use in Python, each suitable for different types of data and requirements.
Statistical Methods
Statistical methods are the simplest approach to anomaly detection.
These methods rely on assumptions about the distribution of data.
For example, a common statistical method is to assume that data follows a normal distribution.
Anomalies then can be identified as those points that lie outside a certain deviation from the mean.
These methods are easy to implement but may not be effective for complex datasets that don’t follow a clear distribution pattern.
Machine Learning Methods
Machine learning methods apply trained models to detect anomalies.
Supervised learning involves training models with labelled datasets including both normal and anomalous data points.
Unsupervised learning, on the other hand, involves clustering and clustering-based methods such as k-means and DBSCAN, which help in identifying clusters and outliers with no prior labels.
An unsupervised approach like Isolation Forest, which works by isolating anomalies more easily than normal observations, represents another powerful tool.
Deep Learning Methods
Deep learning methods are widely popularized due to their capability to handle large volumes of data with complex patterns.
Autoencoders and Generative Adversarial Networks (GANs) are examples of neural network architectures used in anomaly detection.
They are powerful but require significant computational resources and a large amount of data for training.
Implementing Anomaly Detection in Python
Here, we’ll walk through a very basic implementation of anomaly detection using Python with Scikit-learn, a powerful machine-learning library.
Installation and Setup
Firstly, ensure you have Python and pip installed on your system.
You can install Scikit-learn using pip:
“`
pip install scikit-learn
“`
Loading Your Data
You’ll need a dataset to work with.
For demonstration, you can use the Iris dataset, a commonly used dataset available in Scikit-learn.
“`python
from sklearn.datasets import load_iris
data = load_iris()
X = data.data
“`
Isolation Forest Example
Anomaly detection with Isolation Forest can be implemented in just a few steps using Scikit-learn.
“`python
from sklearn.ensemble import IsolationForest
import numpy as np
# Set random seed for reproducibility
np.random.seed(42)
# Create the Isolation Forest model
model = IsolationForest(n_estimators=100, contamination=0.1)
# Fit the model
model.fit(X)
# Predict anomalies
anomalies = model.predict(X)
# Identify the anomalies
anomaly_points = np.where(anomalies == -1)
print(“Anomalies detected at data points:”, anomaly_points)
“`
Evaluating Model Performance
It’s essential to test and evaluate the anomaly detection model effectively.
Model evaluation will depend on the data and method used.
Precision, recall, and the F1-score are effective metrics when evaluating performance on labelled datasets.
“`python
from sklearn.metrics import classification_report
# Dummy true labels, the real dataset should have actual labels
true_labels = np.concatenate((np.ones(140), -1 * np.ones(10)))
print(classification_report(true_labels, anomalies))
“`
Conclusion
Anomaly detection is a powerful technique essential for ensuring data integrity and security across diverse fields.
Python’s extensive libraries and easy-to-use syntax simplify implementing various anomaly detection methods.
Whether through statistical methods, machine learning, or deep learning, Python allows you to harness anomaly detection effectively, providing meaningful insights into your data.
By leveraging Python for anomaly detection, you can efficiently identify outliers, making informed decisions and safeguarding the systems you are managing.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)