- お役立ち記事
- Basics and implementation points of deep learning using Python ~Demo included~
Basics and implementation points of deep learning using Python ~Demo included~
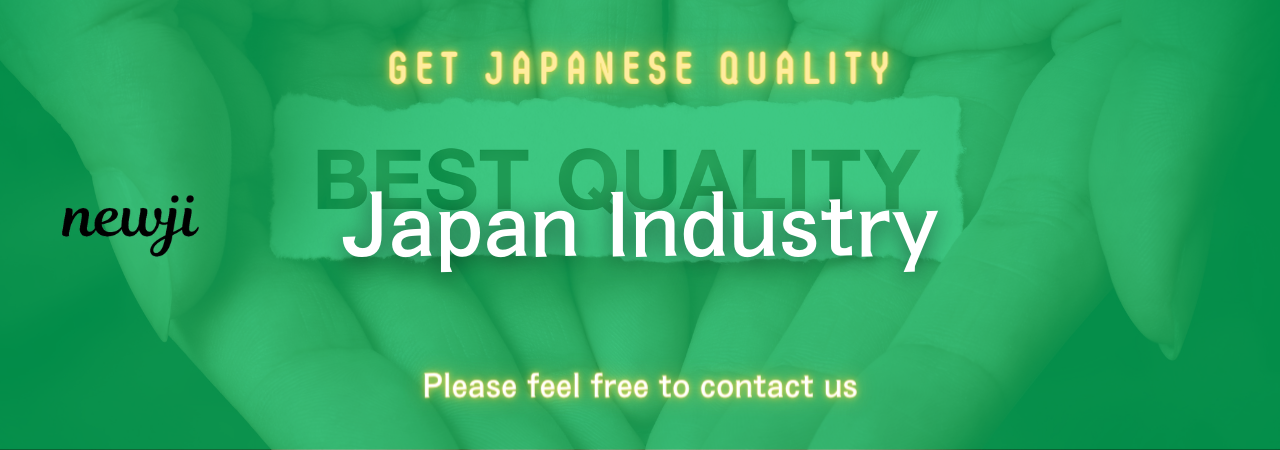
目次
Introduction to Deep Learning
Deep learning has become a significant area of interest in the field of artificial intelligence and machine learning.
This advanced technology allows computers to learn from vast amounts of data and make intelligent decisions based on that information.
At the heart of deep learning are neural networks, which are inspired by the structure and function of the human brain.
Python, a highly versatile programming language, is widely used for implementing deep learning algorithms.
In this article, we will discuss the basics of deep learning, explore its implementation using Python, and provide a demo to illustrate the concepts.
Understanding Deep Learning and Neural Networks
What is Deep Learning?
Deep learning is a subset of machine learning that focuses on using neural networks with many layers (often referred to as deep neural networks) to process data and generate predictions or classifications.
It’s designed to automatically learn hierarchical features from data by transforming it through multiple layers of computation.
Deep learning has achieved remarkable success in various applications, including image and speech recognition, natural language processing, and autonomous systems.
Neural Networks Explained
Neural networks are computational models inspired by the human brain and consist of interconnected nodes, or neurons, which process and transmit information.
A simple neural network consists of an input layer, one or more hidden layers, and an output layer.
Each layer is composed of neurons, and each connection between neurons has an associated weight that determines the influence of the input data on the final output.
The key feature of deep learning is the presence of multiple hidden layers, which allow for the extraction of complex patterns from data.
Getting Started with Python for Deep Learning
Python has become the go-to language for deep learning due to its simplicity and extensive libraries that facilitate the development of neural network architectures.
Installing Necessary Libraries
Before diving into deep learning with Python, you need to install essential libraries that provide the required tools and functions needed to build and train neural networks.
The most popular libraries for deep learning in Python include:
– TensorFlow: A powerful library developed by Google, TensorFlow is used for large-scale machine learning and deep learning tasks.
– Keras: A high-level neural networks API written in Python that runs on top of TensorFlow, making it easy to build and train deep learning models.
– PyTorch: Developed by Facebook’s AI Research lab, PyTorch is a flexible and dynamic library that allows for easy experimentation.
To install these libraries, you can use pip, the package manager for Python, by running the following commands in your terminal or command prompt:
“`
pip install tensorflow
pip install keras
pip install torch
“`
Creating a Simple Neural Network
Once the libraries are installed, you can start building your first neural network.
Here, we’ll create a simple feedforward neural network using Keras to classify a basic dataset.
“`python
import keras
from keras.models import Sequential
from keras.layers import Dense
# Define the Sequential model
model = Sequential()
# Add an input layer and a first hidden layer
model.add(Dense(units=10, activation=’relu’, input_dim=20))
# Add a second hidden layer
model.add(Dense(units=10, activation=’relu’))
# Add an output layer
model.add(Dense(units=1, activation=’sigmoid’))
# Compile the model
model.compile(optimizer=’adam’, loss=’binary_crossentropy’, metrics=[‘accuracy’])
# Print the model summary
model.summary()
“`
In this example, we initialize a `Sequential` model and add multiple `Dense` layers to define the architecture of the neural network.
The `input_dim` parameter specifies the number of input features, and the neurons in each layer use the ReLU activation function, except for the output layer, which uses the sigmoid function suitable for binary classification.
Training and Evaluating the Model
Once the model architecture is defined, the next step is to train the model using a dataset.
The training process involves feeding input data into the network, calculating the error (loss), and updating the weights using an optimization algorithm to minimize the loss.
Preparing the Dataset
For the sake of demonstration, let’s assume we have a simple dataset with binary output labels (e.g., 0 or 1):
“`python
import numpy as np
# Generate a random dataset with 1000 samples and 20 features
X_train = np.random.rand(1000, 20)
y_train = np.random.randint(2, size=1000)
“`
Training the Neural Network
With the dataset ready, you can train the neural network using the `fit` method:
“`python
# Train the model on the training data
model.fit(X_train, y_train, epochs=10, batch_size=32)
“`
In this example, we train the model for 10 epochs with a batch size of 32.
Each epoch represents a complete iteration over the entire dataset.
Evaluating the Model
After training, it’s crucial to evaluate the model’s performance on unseen data to ensure its accuracy and generalization capability:
“`python
# Generate a random test dataset
X_test = np.random.rand(200, 20)
y_test = np.random.randint(2, size=200)
# Evaluate the model on the test data
loss, accuracy = model.evaluate(X_test, y_test)
print(f”Test Loss: {loss}”)
print(f”Test Accuracy: {accuracy}”)
“`
Here, we use the `evaluate` method to calculate the loss and accuracy on a test dataset, providing a measure of how well the model performs on new data.
Conclusion
Deep learning with Python offers endless possibilities for creating intelligent systems capable of learning from complex data.
This article has introduced the basics of deep learning, the role of neural networks, and how to implement them using Python and its powerful libraries such as TensorFlow and Keras.
By following the provided example, you can start building your neural networks and delve deeper into the fascinating world of deep learning.
The journey doesn’t end here; there are many advanced concepts, such as convolutional neural networks and recurrent neural networks, which you can explore to tackle more complex tasks and make significant advancements in artificial intelligence applications.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)