- お役立ち記事
- Basics and practical applications of sparse modeling using Python
Basics and practical applications of sparse modeling using Python
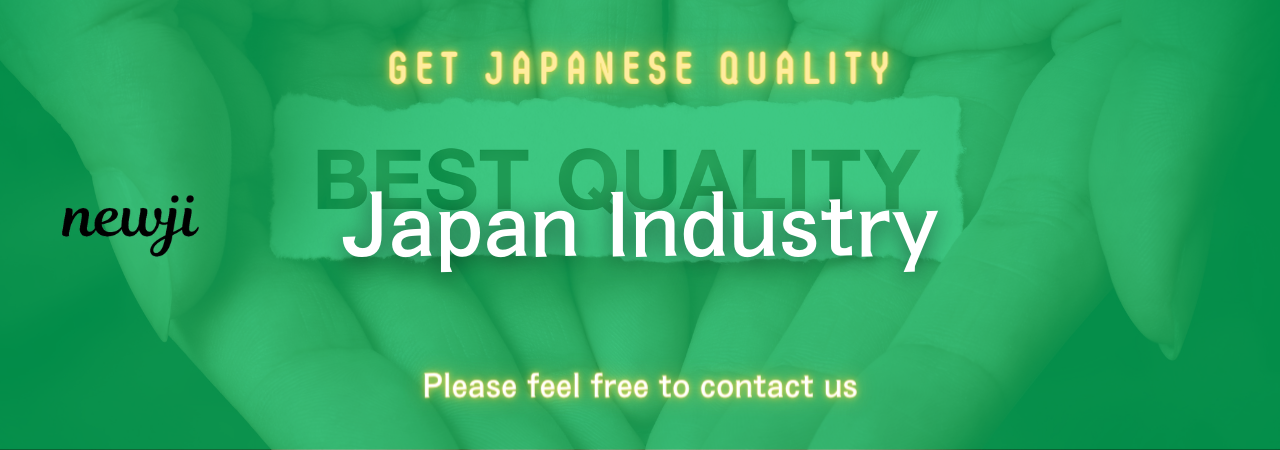
目次
Introduction to Sparse Modeling
Sparse modeling is a technique used in data science and machine learning to handle high-dimensional data more efficiently by assuming that the underlying data structure is sparse.
This means that most of the values in the dataset are zero or irrelevant, allowing for a more efficient processing of the non-zero elements.
Python, with its rich ecosystem of libraries, provides an ideal platform for implementing sparse modeling techniques.
In this article, we will delve into the basics of sparse modeling and explore how you can practically apply these techniques using Python.
Understanding Sparsity
Sparse data refers to data in which the majority of the elements are zero or have minimal impact on the system being modeled.
An excellent example of this is text data processed in the form of a bag-of-words representation, where most of the elements in the vector are zeros for large vocabularies.
Sparse modeling aims to focus on the important, non-zero features, allowing for more efficient computations and simpler models.
The concept of sparsity is leveraged in various fields, such as image processing, natural language processing, and signal processing, to name a few.
It is particularly useful when dealing with large datasets and complex models, helping to reduce overfitting and improve interpretability.
The Basics of Sparse Modeling
Sparse modeling involves techniques that lead to sparse solutions.
Some of the common methods include L1 regularization, compressed sensing, and matrix factorization techniques.
Let’s take a closer look at these methods.
L1 Regularization
L1 regularization, also known as Lasso regularization, is a technique commonly used in linear regression models to promote sparsity.
By adding a penalty term to the loss function proportional to the absolute value of the coefficients, L1 regularization results in some coefficients being exactly zero, effectively selecting a subset of features.
The L1 regularization term can be expressed as:
\( L1(w) = \lambda \sum |w_i| \)
where \( w \) is the weight vector and \( \lambda \) is the regularization strength.
Compressed Sensing
Compressed sensing is a method that reconstructs a signal or image from a small number of measurements, assuming that the signal is sparse in some basis.
The key idea is that, if the data is sparse, fewer samples are needed to accurately reconstruct it, compared to traditional methods.
Python offers various libraries like `scipy` and `sklearn` that facilitate implementations of compressed sensing algorithms, empowering users with tools for effective signal and image compression.
Matrix Factorization
Matrix factorization techniques such as Singular Value Decomposition (SVD) and Principal Component Analysis (PCA) can also be leveraged for sparse modeling.
These methods decompose a matrix into smaller, more manageable parts, revealing the underlying sparse structure.
Python’s numerical libraries, such as `numpy` and `scipy`, provide efficient implementations of these matrix factorization techniques, allowing for seamless integration into data processing workflows.
Implementing Sparse Modeling in Python
Python’s ecosystem includes several libraries and functions to implement sparse modeling efficiently.
In this section, we’ll explore practical implementation using Python, focusing on using libraries like `scikit-learn`, `scipy`, and `numpy`.
Using Scikit-learn for Sparse Modeling
`scikit-learn` is a popular machine learning library in Python that provides extensive support for modeling with sparse data.
For instance, one can perform L1 regularization using the `Lasso` class from `scikit-learn`.
“`python
from sklearn.linear_model import Lasso
from sklearn.datasets import make_regression
# Generate a sample dataset
X, y = make_regression(n_samples=100, n_features=20, noise=0.1, random_state=42)
# Create a Lasso regression model with L1 regularization
lasso = Lasso(alpha=0.1)
# Fit the model on data
lasso.fit(X, y)
# Output the sparse coefficients
print(“Sparse Coefficients:”, lasso.coef_)
“`
The snippet above generates a regression dataset and fits a Lasso model, resulting in a sparse representation of the coefficients.
Working with Scipy for Sparse Matrices
`scipy` is a fundamental library for scientific computing in Python and provides robust support for sparse matrices.
This is particularly useful in handling large datasets with a significant number of zero values, optimizing both storage and computation time.
“`python
from scipy.sparse import csr_matrix
# Create a dense matrix
dense_matrix = [[0, 0, 3], [4, 0, 0], [0, 5, 0]]
# Convert the dense matrix to a sparse matrix (CSR format)
sparse_matrix = csr_matrix(dense_matrix)
print(“Sparse Matrix Data:”, sparse_matrix.data)
print(“Indices of non-zero elements:”, sparse_matrix.indices)
“`
This code demonstrates the conversion of a dense matrix into a sparse matrix using the Compressed Sparse Row (CSR) format.
Applications of Sparse Modeling
Sparse modeling has numerous applications across different domains:
Image Compression
In image processing, sparse modeling techniques can efficiently compress and reconstruct images.
By focusing on non-zero coefficients, these techniques significantly reduce image data size without compromising on quality.
Natural Language Processing
Sparse modeling is crucial in natural language processing tasks like text classification and sentiment analysis, where text data is converted into high-dimensional feature vectors.
Signal Processing
In signal processing, techniques like compressed sensing leverage sparsity to reconstruct signals accurately from minimal sampling, greatly benefiting fields like wireless communication and medical imaging.
Conclusion
Sparse modeling is a powerful technique in data science and machine learning, enabling efficient handling of high-dimensional data.
With Python’s robust libraries, implementing sparse modeling techniques becomes accessible and effective.
Understanding the basics of sparsity, utilizing tools like scikit-learn and scipy, and recognizing the wide range of applications can enhance your data processing workflow and lead to more accurate and interpretable models.
Whether you’re working with images, text, or signals, embracing sparse modeling techniques can significantly improve outcomes in various data-rich environments.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)