- お役立ち記事
- Basics and practice of classification, regression, and time series processing using Python and TF2.0 (Keras)
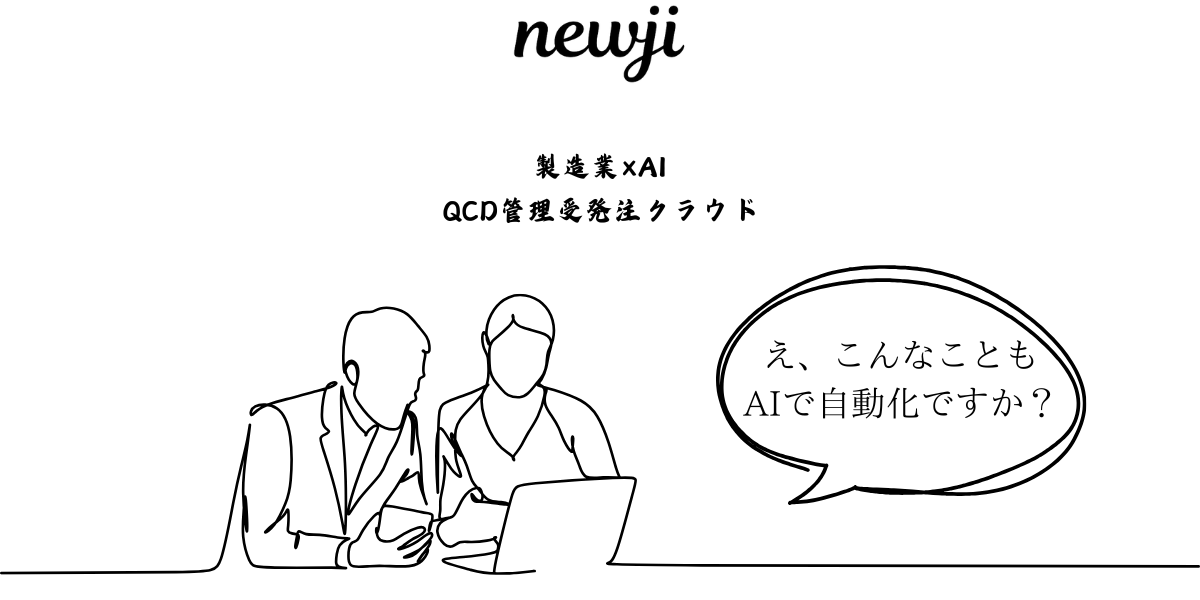
Basics and practice of classification, regression, and time series processing using Python and TF2.0 (Keras)
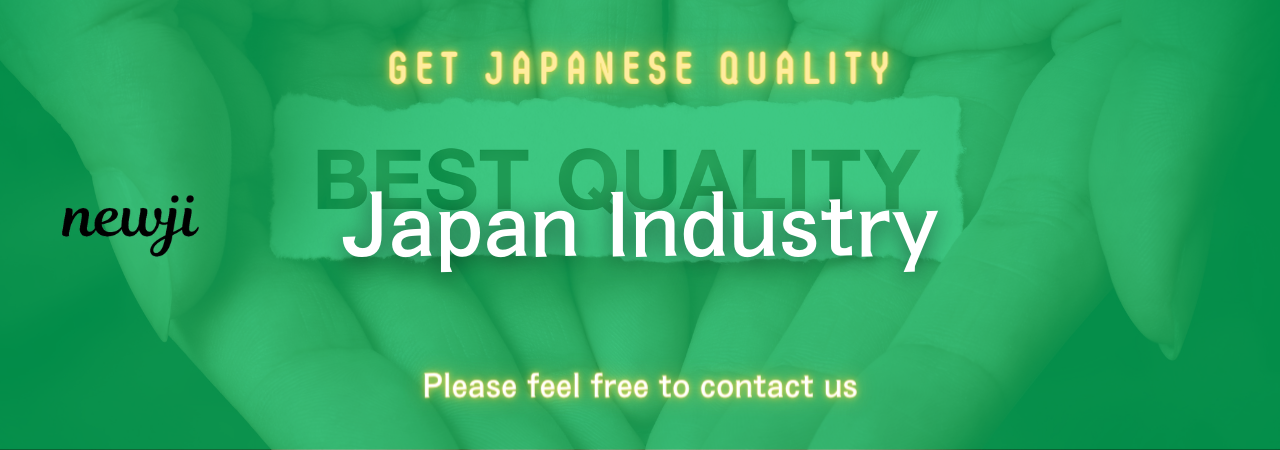
目次
Introduction to Machine Learning with Python and TF2.0
Python and TensorFlow 2.0 (TF2.0) are powerful tools used extensively in machine learning projects.
Understanding the basics of classification, regression, and time series analysis using these tools can open many doors in the realm of artificial intelligence (AI).
In this guide, we will explore these key areas and provide insights into their practice with Python and TF2.0, leveraging Keras, which simplifies the process of building and training deep learning models.
Understanding Classification
Classification is a fundamental machine learning task where the goal is to assign a label or category to input data.
This process can be applied to a variety of real-world problems such as spam detection, image recognition, and sentiment analysis.
Getting Started with Classification
To begin with classification using Python, you need to load and preprocess your dataset.
Common datasets for learning classification include MNIST for digit recognition or CIFAR-10 for image classification.
Using libraries such as NumPy, Pandas, and Scikit-learn, you can clean and prepare your data for analysis.
Building a Classification Model with Keras
Once your data is ready, you can build a neural network model using Keras, a high-level API for TF2.0.
Start by defining a sequential model and adding layers.
Here’s a quick example:
“`python
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(128, activation=’relu’, input_shape=(input_shape,)),
Dense(64, activation=’relu’),
Dense(num_classes, activation=’softmax’)
])
“`
In this example, a simple feedforward neural network is created with one input layer, two hidden layers, and an output layer with a softmax activation function for classification.
Training and Evaluating the Model
Compile your model using an appropriate optimizer and loss function.
For a classification problem, you might use Adam optimizer and categorical crossentropy as the loss function.
Then, fit the model to your data:
“`python
model.compile(optimizer=’adam’, loss=’categorical_crossentropy’, metrics=[‘accuracy’])
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
“`
Evaluate the model using test data to measure its performance:
“`python
model.evaluate(x_test, y_test)
“`
Exploring Regression with Python and TF2.0
Regression analysis is another core task in machine learning where the goal is to predict continuous values.
Applications include price forecasting, stock prediction, and risk estimation.
Preparing Data for Regression
Like classification, regression requires data preprocessing.
This involves handling missing values, normalizing features, and splitting data into training and testing sets.
Creating a Regression Model
In Keras, a regression model can be built similarly to a classification model.
However, the output layer will have a linear activation function to handle continuous variables.
“`python
model = Sequential([
Dense(128, activation=’relu’, input_shape=(input_shape,)),
Dense(64, activation=’relu’),
Dense(1, activation=’linear’)
])
“`
This structure is suitable for predicting a single-continuous feature.
Training the Regression Model
Compile the model using a regression-specific loss function like mean squared error (MSE) and a suitable optimizer.
Train the model to fit your data:
“`python
model.compile(optimizer=’adam’, loss=’mean_squared_error’, metrics=[‘mean_absolute_error’])
model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_val, y_val))
“`
The lower the mean absolute error (MAE), the better the model’s predictive performance.
Introduction to Time Series Processing
Time series analysis involves working with data that is ordered over time.
This is crucial for sectors like finance, meteorology, and IoT where trends and patterns over time are significant.
Preparing Time Series Data
Time series data needs special attention in terms of preprocessing.
It often includes steps such as resampling, detrending, scaling, and splitting.
Pandas provides excellent support for time series data manipulation.
Building a Time Series Model
Recurrent Neural Networks (RNNs) and Long Short-Term Memory networks (LSTMs) are popular for time series prediction due to their ability to capture temporal features.
“`python
from tensorflow.keras.layers import LSTM
model = Sequential([
LSTM(50, activation=’relu’, input_shape=(n_timesteps, n_features)),
Dense(1)
])
“`
The LSTM layer helps retain knowledge from sequential data, making it effective for time-dependent predictions.
Training a Time Series Model
The model training process is similar to regression but may require more epochs due to the complexity of time dependencies.
“`python
model.compile(optimizer=’adam’, loss=’mean_squared_error’)
model.fit(x_train, y_train, epochs=50, batch_size=32, validation_data=(x_val, y_val))
“`
Evaluating and tuning time series models might involve more extensive hyperparameter adjustments to achieve the desired predictive accuracy.
Conclusion
By harnessing the power of Python and TensorFlow 2.0, you can effectively tackle problems involving classification, regression, and time series analysis.
Keras simplifies the model-building process, allowing you to focus on problem-solving.
Understanding these basic concepts equips you with essential tools for a career in AI and machine learning.
By practicing and experimenting, you will deepen your expertise in developing sophisticated models that address real-world challenges.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)