- お役立ち記事
- Basics and practice of image processing using OpenCV and Python
月間73,982名の
製造業ご担当者様が閲覧しています*
*2025年1月31日現在のGoogle Analyticsのデータより
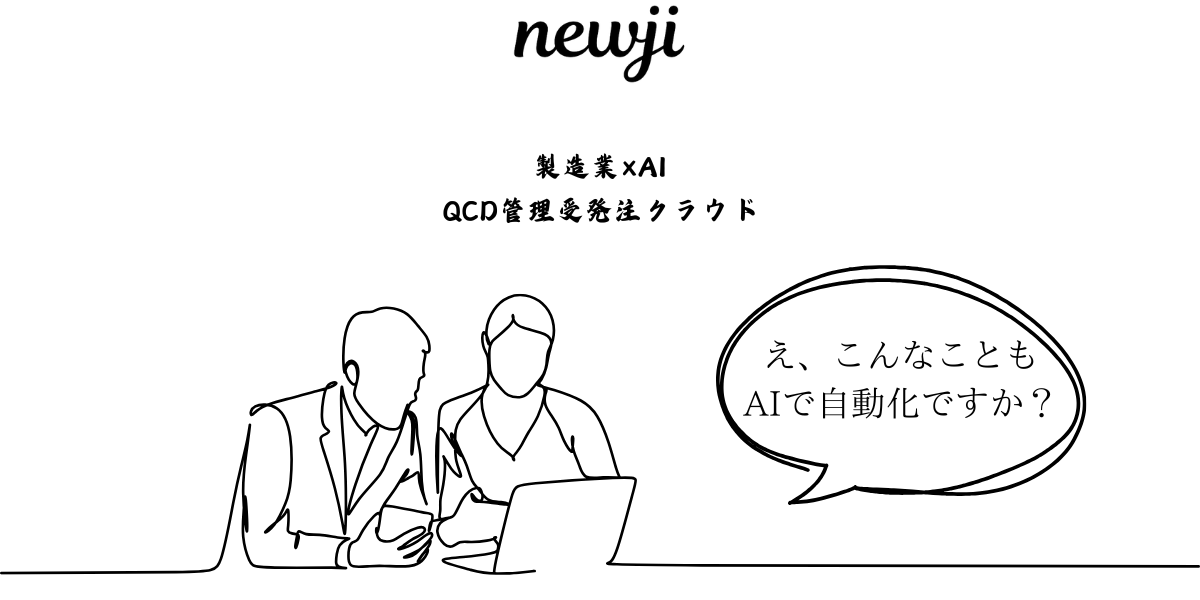
Basics and practice of image processing using OpenCV and Python
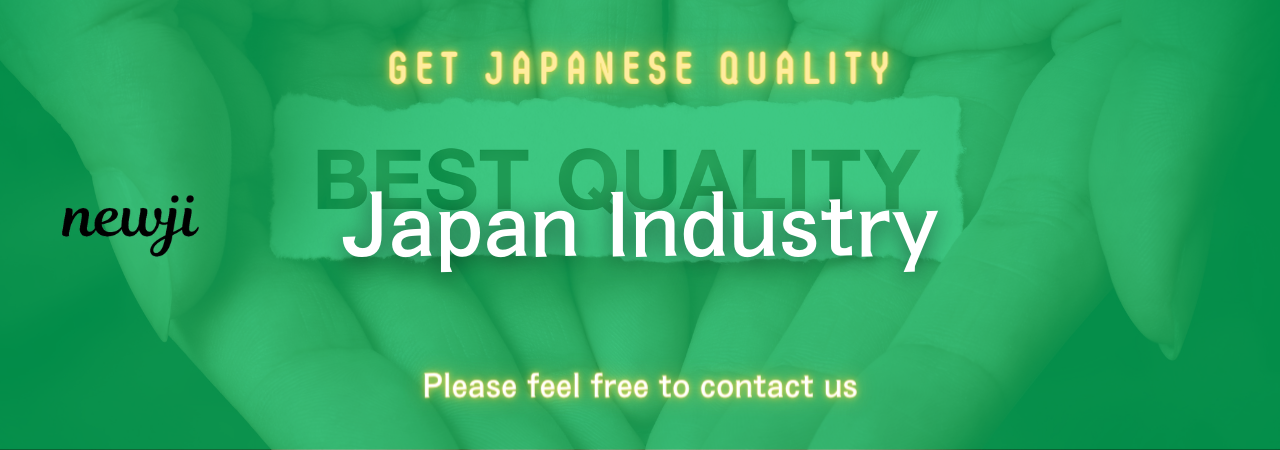
目次
Introduction to Image Processing
Image processing is an exciting field that involves the manipulation and analysis of images to extract meaningful information or improve their quality.
With advancements in technology, image processing has become an essential part of various applications, including medical imaging, computer vision, and photography.
In this article, we’ll delve into the basics of image processing using OpenCV, a popular library, and Python, a versatile programming language.
What is OpenCV?
OpenCV, or Open Source Computer Vision Library, is an open-source computer vision and machine learning software library.
It is designed to provide a common infrastructure for computer vision applications and to accelerate the use of machine perception in commercial products.
OpenCV has a comprehensive set of tools and functions for image processing, making it a go-to library for developers and researchers alike.
Setting Up OpenCV and Python
Before diving into image processing tasks, you need to set up OpenCV and Python on your computer.
Here are simple steps to get you started:
1. **Install Python**: Ensure you have Python installed on your machine.
You can download it from the official Python website.
2. **Install OpenCV**: Use pip, a package manager for Python, to install OpenCV.
Open your command prompt or terminal and type:
“`
pip install opencv-python
pip install opencv-python-headless
“`
3. **Verify Installation**: Check if OpenCV is installed correctly by opening a Python terminal and typing:
“`
import cv2
print(cv2.__version__)
“`
If everything is set up correctly, you’ll see the version number of the installed OpenCV.
Basic Image Operations
Let’s explore some basic operations you can perform on images using OpenCV and Python.
Loading and Displaying Images
To start with image processing, you need to load an image into your program.
You can use the `imread` function from OpenCV:
“`python
import cv2
# Load an image
image = cv2.imread(‘path_to_image.jpg’)
# Display the image
cv2.imshow(‘Loaded Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Here, `cv2.imread` loads the image from the specified path, and `cv2.imshow` displays it in a window.
Resizing Images
Often, you may need to resize images to different dimensions.
You can use the `resize` function for this:
“`python
# Resize the image
resized_image = cv2.resize(image, (width, height))
“`
Replace `width` and `height` with the desired dimensions.
Converting to Grayscale
Converting an image to grayscale is a common preprocessing step in image processing.
Use the `cvtColor` function to achieve this:
“`python
# Convert to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
“`
Grayscale images simplify complex algorithms by reducing the color information.
Image Transformation Techniques
Rotating Images
Rotating images can be done using the `getRotationMatrix2D` and `warpAffine` functions:
“`python
# Get rotation matrix
angle = 45 # Angle in degrees
center = (image.shape[1] // 2, image.shape[0] // 2)
rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0)
# Rotate the image
rotated_image = cv2.warpAffine(image, rotation_matrix, (image.shape[1], image.shape[0]))
“`
Image Blurring
Blurring an image can be helpful for reducing noise or creating a smooth effect.
OpenCV allows various blurring techniques; one common method is Gaussian Blur:
“`python
# Apply Gaussian Blur
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
“`
The `(5, 5)` parameter defines the kernel size, and altering it can change the blurring effect.
Edge Detection
Edge detection helps identify the boundaries within images.
Canny Edge Detection is a popular technique used in OpenCV:
“`python
# Perform Canny Edge Detection
edges = cv2.Canny(gray_image, 100, 200)
“`
The first parameter is the grayscale image, and the numbers `100` and `200` define the thresholds for detecting edges.
Conclusion
Image processing is a fascinating field with endless possibilities.
Using OpenCV and Python, you have powerful tools at your disposal to perform numerous operations on images.
From basic manipulations like resizing and converting to more advanced techniques like edge detection, the combination of OpenCV and Python makes it easier than ever to work with images.
Whether you’re a beginner or an experienced developer, exploring image processing with OpenCV can open doors to exciting projects and innovative solutions.
So, grab your computer, install OpenCV, and start experimenting with images today!
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)