- お役立ち記事
- Basics and usage of deep learning using PyTorch and programming practice
月間76,176名の
製造業ご担当者様が閲覧しています*
*2025年3月31日現在のGoogle Analyticsのデータより
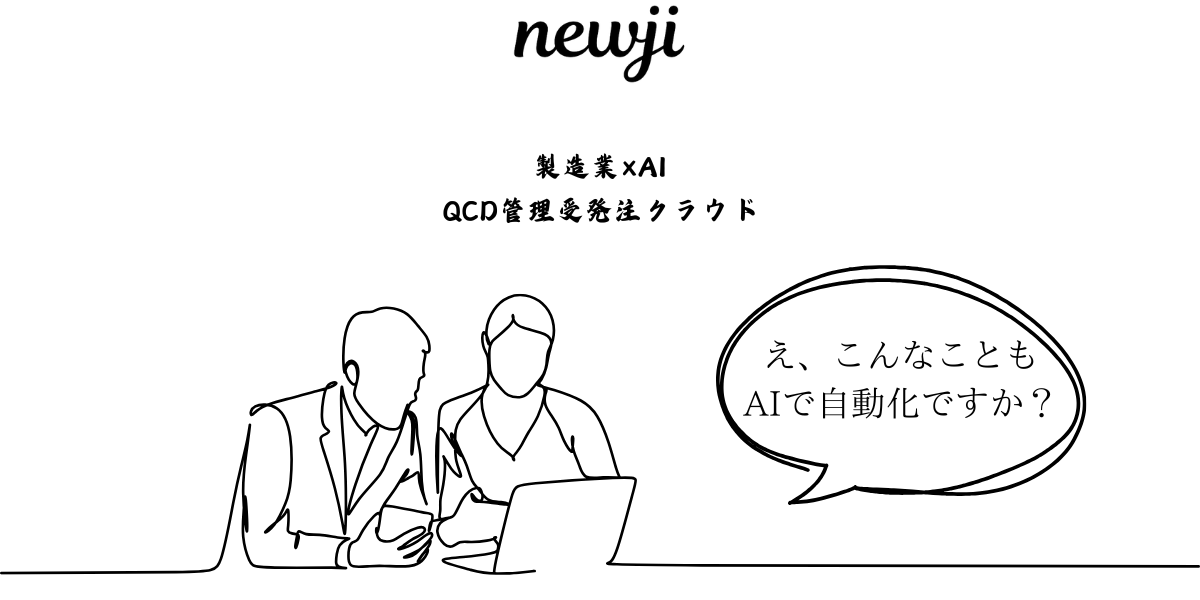
Basics and usage of deep learning using PyTorch and programming practice
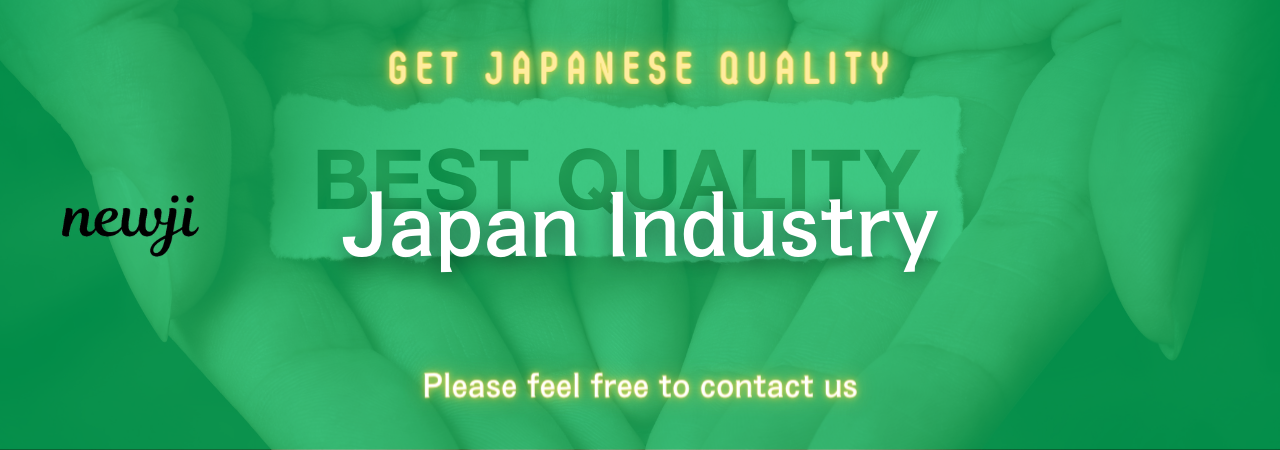
目次
Introduction to Deep Learning
Deep learning is a subfield of artificial intelligence (AI) focused on creating and using neural networks that mimic the human brain’s functionality.
These networks consist of layers that enable computers to learn from large amounts of data.
In recent years, deep learning has become essential in various fields, including image recognition, natural language processing, and autonomous vehicles.
What is PyTorch?
PyTorch is an open-source machine learning library developed by Facebook’s AI Research lab.
It has gained popularity for its flexibility and ease of use, especially among researchers and developers.
PyTorch provides a seamless and intuitive interface for building deep learning models, making it ideal for both beginners and experienced practitioners.
Why Use PyTorch?
PyTorch’s ease of use stems from its dynamic computational graph, which allows developers to change the network behavior on the fly.
This characteristic is particularly useful for complex models like those used in research settings, where experiments often require frequent adjustments.
Moreover, with PyTorch, users benefit from a comprehensive set of utilities for deep learning tasks, as well as extensive support and resources from a large and active community.
Flexibility and Customization
PyTorch’s dynamic computational graph system provides unparalleled flexibility when building and modifying models.
This feature empowers users to write code that feels like regular Python programming, facilitating readability and customization.
Extensive Support and Resources
The PyTorch community continuously contributes to its development by providing numerous tutorials, guides, and forums dedicated to problem-solving and learning new techniques.
This makes PyTorch a suitable choice for beginners seeking support during their learning journey.
Setting Up PyTorch
Before diving into programming with PyTorch, you need to install it on your computer.
The installation process is straightforward, but it varies according to your machine’s configuration.
Installing PyTorch
First, you’ll need to verify whether you have Python installed on your system.
If not, download and install it from the official Python website.
After installing Python, you can install PyTorch using a package manager like pip or conda.
Run the following command in your terminal to install PyTorch using pip:
“`
pip install torch torchvision torchaudio
“`
Alternatively, use conda to install PyTorch by executing the following command:
“`
conda install pytorch torchvision torchaudio -c pytorch
“`
Now that PyTorch is installed, you’re ready to explore its functionality and start building deep learning models.
Understanding Neural Networks
Neural networks, the building blocks of deep learning, consist of interconnected layers of nodes, or “neurons.”
Each layer transforms the input data in various ways, allowing the network to learn and make predictions.
Layers and Activation Functions
In a neural network, each layer processes the input from the previous one.
The first layer, known as the input layer, receives the raw data.
Subsequent layers, called hidden layers, apply distinct mathematical operations, including activation functions like ReLU or Sigmoid, to learn complex patterns.
The final layer, called the output layer, returns the network’s predicted output.
Training Neural Networks
Training a neural network involves adjusting the weights and biases of each node to minimize the difference between predicted and actual values.
This process is usually accomplished through optimization techniques like gradient descent.
During training, the network employs a loss function to evaluate its performance.
The loss function measures the discrepancy between predicted and actual values, instructing the network on how to update its parameters for better accuracy.
Building a Simple Neural Network with PyTorch
To illustrate PyTorch’s capabilities, let’s construct a simple neural network for image classification.
This example will focus on classifying handwritten digits from the popular MNIST dataset.
Loading the Dataset
First, import necessary libraries and load the dataset using PyTorch’s built-in utilities:
“`python
import torch
import torchvision
import torchvision.transforms as transforms
# Transformations to normalize the data
transform = transforms.Compose([transforms.ToTensor(),
transforms.Normalize((0.5,), (0.5,))])
# Load the training and test datasets
trainset = torchvision.datasets.MNIST(root=’./data’, train=True,
download=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=4,
shuffle=True)
testset = torchvision.datasets.MNIST(root=’./data’, train=False,
download=True, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=4,
shuffle=False)
“`
Defining the Neural Network Architecture
Next, define a simple neural network model using PyTorch’s `torch.nn` module:
“`python
import torch.nn as nn
import torch.nn.functional as F
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.layer1 = nn.Linear(28*28, 128)
self.layer2 = nn.Linear(128, 64)
self.layer3 = nn.Linear(64, 10)
def forward(self, x):
x = x.view(-1, 28*28)
x = F.relu(self.layer1(x))
x = F.relu(self.layer2(x))
x = self.layer3(x)
return x
“`
Training the Neural Network
Instantiate the network, define the loss function, and choose an optimizer:
“`python
net = SimpleNet()
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(net.parameters(), lr=0.01, momentum=0.9)
“`
Now, train the network:
“`python
for epoch in range(5):
running_loss = 0.0
for i, data in enumerate(trainloader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 1000 == 999:
print(f”[{epoch + 1}, {i + 1}] loss: {running_loss / 1000:.3f}”)
running_loss = 0.0
print(“Finished Training”)
“`
Evaluating the Model
After training, evaluate your model with the test dataset to measure its accuracy:
“`python
correct = 0
total = 0
with torch.no_grad():
for data in testloader:
images, labels = data
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f”Accuracy: {100 * correct / total}%”)
“`
This concludes our introduction to using PyTorch for building and training a simple neural network.
As you become more familiar with PyTorch, you’ll discover its advanced features, which will enable you to tackle increasingly complex deep learning problems.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)