- お役立ち記事
- Basics of image processing technology using Python and image recognition programming using deep learning
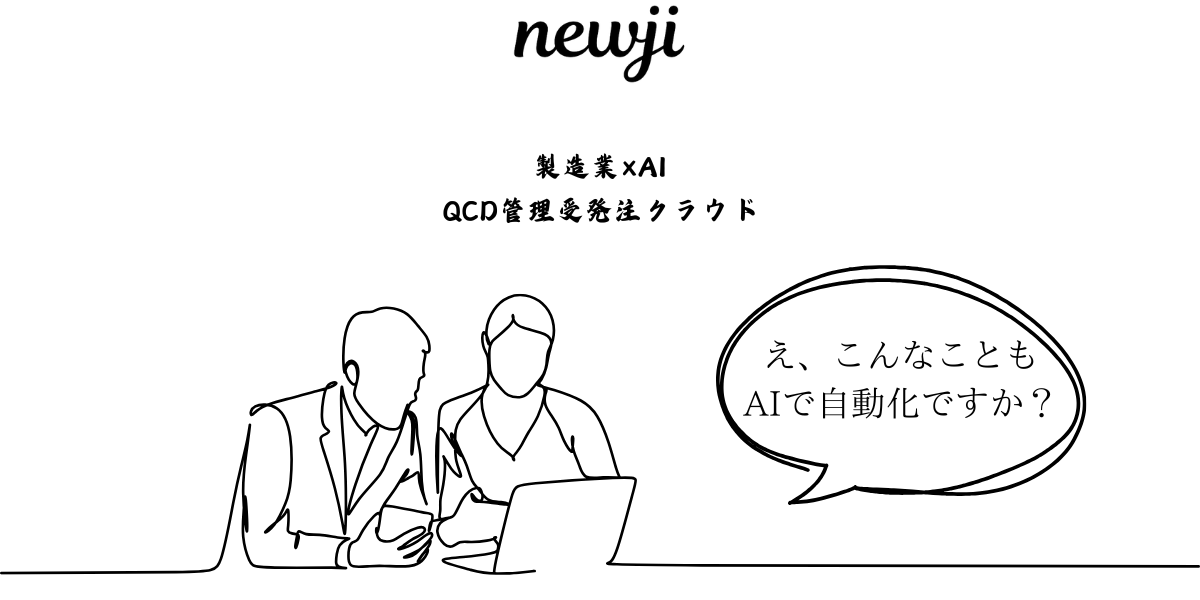
Basics of image processing technology using Python and image recognition programming using deep learning
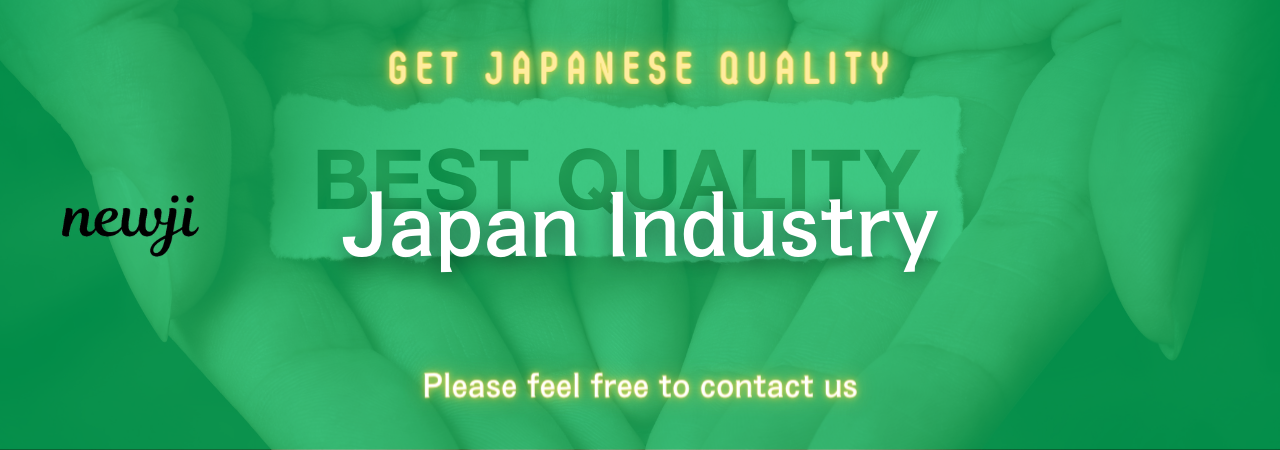
目次
Introduction to Image Processing with Python
Image processing is a method used to perform operations on images to enhance them or extract useful information.
It’s a growing field with numerous applications in various industries, such as medical imaging, computer vision, and robotics.
Python, as a versatile programming language, offers powerful libraries for image processing.
These libraries make it easier for developers and data scientists to apply complex algorithms with minimal coding effort.
Why Use Python for Image Processing?
Python is popular for image processing due to its simplicity and the vast range of libraries it offers.
These libraries, like OpenCV, PIL, and Scikit-Image, provide a wide variety of functions for image manipulation.
Python’s easy-to-read syntax enables developers to focus on algorithms and problem-solving.
Additionally, Python’s strong community support means there’s a wealth of resources available for learners at all skill levels.
Getting Started with Python Libraries
To begin processing images with Python, you must first install some essential libraries.
Here’s a brief overview of some common Python libraries used in image processing:
OpenCV
Open Source Computer Vision Library (OpenCV) is a widely-used open-source library for machine learning and computer vision tasks.
It allows users to read, write, and process images and videos.
With OpenCV, you can perform operations like image filtering, edge detection, and face recognition.
Install OpenCV using pip with the following command:
“`
pip install opencv-python
“`
PIL/Pillow
The Python Imaging Library (PIL) is an older library for image processing tasks.
Pillow is its modernized fork that is more actively maintained.
Pillow supports various image file formats and provides tools for opening, manipulating, and saving images.
Use the command below to install Pillow:
“`
pip install pillow
“`
Scikit-Image
Scikit-Image is a library built on top of SciPy, specializing in image processing.
It offers functions for image segmentation, transformation, and feature extraction.
Scikit-Image is an excellent choice for beginners due to its extensive documentation and user-friendly examples.
You can install Scikit-Image using:
“`
pip install scikit-image
“`
Basic Image Processing Techniques
Once you’ve installed the necessary libraries, it’s time to dive into some basic image processing techniques.
These techniques form the foundation for more advanced applications in computer vision and deep learning.
Reading and Displaying Images
The first step in any image processing task is loading an image into your Python program.
Here’s a simple example using OpenCV:
“`python
import cv2
# Load an image from file
image = cv2.imread(‘path_to_image.jpg’)
# Display the image in a window
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
In this example, OpenCV’s `imread` function loads an image, and `imshow` displays it in a window.
Image Resizing
Resizing images is often necessary to speed up processing time and reduce memory usage.
Here’s how you can resize an image using OpenCV:
“`python
import cv2
# Load an image
image = cv2.imread(‘path_to_image.jpg’)
# Resize the image
resized_image = cv2.resize(image, (width, height))
# Display the resized image
cv2.imshow(‘Resized Image’, resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
You can specify the desired width and height as parameters in the `resize` function.
Changing Image Color Spaces
Transforming an image’s color space can highlight specific features or components.
For instance, you can convert an image from the standard BGR to grayscale using OpenCV:
“`python
import cv2
# Load an image
image = cv2.imread(‘path_to_image.jpg’)
# Convert to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Display the grayscale image
cv2.imshow(‘Grayscale Image’, gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Grayscale images are computationally less expensive and are widely used in computer vision applications.
Diving into Deep Learning for Image Recognition
As we delve deeper into image processing, we encounter image recognition, a crucial aspect of computer vision.
Deep learning, especially with convolutional neural networks (CNNs), has revolutionized the way we approach image recognition tasks.
Introduction to Deep Learning with TensorFlow and Keras
TensorFlow, an open-source library by Google, is among the most popular platforms for building deep learning models.
Keras, a high-level API for TensorFlow, simplifies the process of building and training deep learning models.
You can install them via pip:
“`
pip install tensorflow
“`
Building a Simple Image Recognition Model
Let’s create a basic image recognition model using Keras with the MNIST dataset, a widely-used benchmark for image processing tasks:
“`python
import tensorflow as tf
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.models import Sequential
# Load the MNIST dataset
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Preprocess the data
x_train, x_test = x_train / 255.0, x_test / 255.0
# Build a simple model
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation=’relu’),
Dense(10, activation=’softmax’)
])
# Compile the model
model.compile(optimizer=’adam’,
loss=’sparse_categorical_crossentropy’,
metrics=[‘accuracy’])
# Train the model
model.fit(x_train, y_train, epochs=5)
# Evaluate the model
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f”Test accuracy: {test_acc}”)
“`
In this example, the model is trained to recognize handwritten digits from the MNIST dataset.
This demonstration showcases how easy it is to implement image recognition models using high-level APIs provided by Keras.
Conclusion
Python’s flexibility and powerful libraries make it an excellent choice for image processing and image recognition tasks.
By leveraging these tools together with deep learning frameworks, you can build and deploy state-of-the-art models for various applications.
As you continue exploring the realm of image processing and recognition, you’ll encounter more advanced techniques and inspiring possibilities to apply them in solving real-world problems.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)