- お役立ち記事
- Basics of image processing using OpenCV and application to image analysis
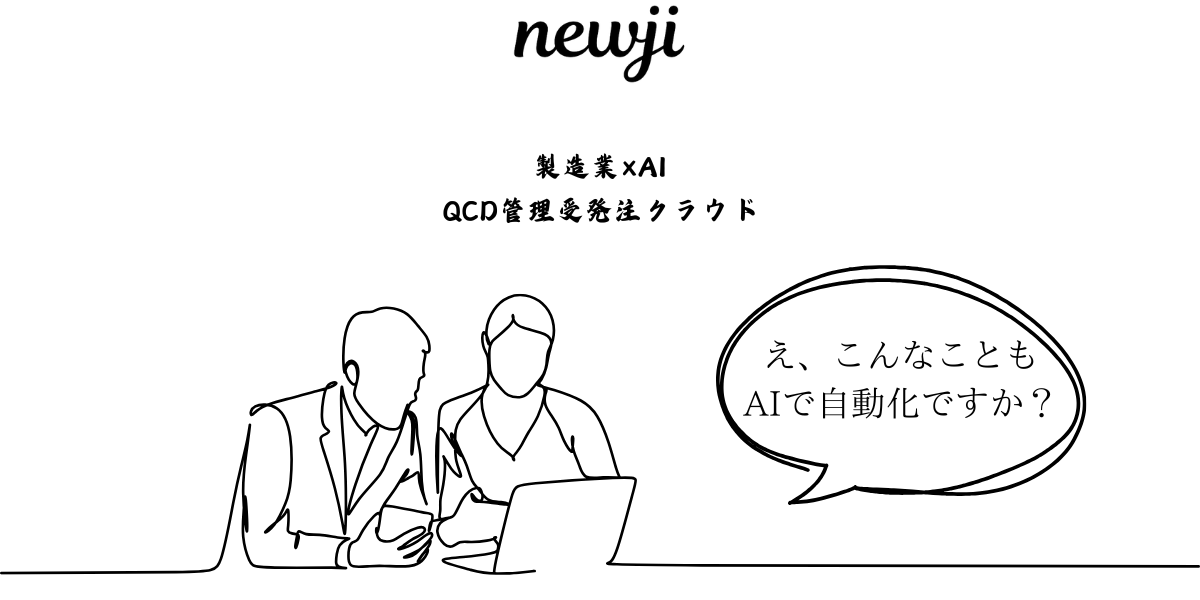
Basics of image processing using OpenCV and application to image analysis
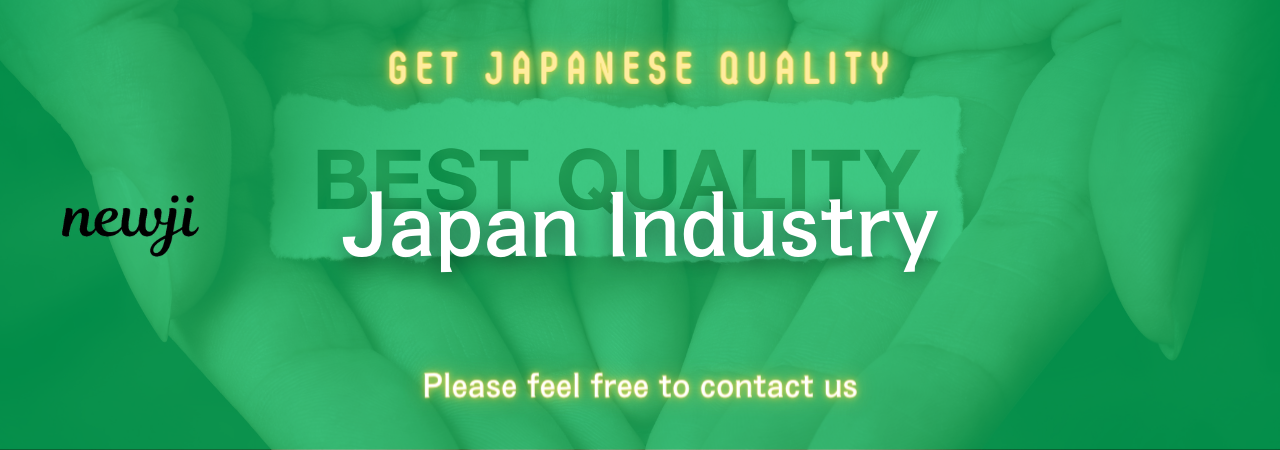
目次
Introduction to Image Processing with OpenCV
Image processing is a technique used to perform various operations on digital images to enhance or extract useful information.
One of the most popular libraries for image processing is OpenCV, which stands for Open Source Computer Vision Library.
OpenCV is a powerful tool that is used in real-time computer vision applications such as facial recognition, object detection, and augmented reality.
In this article, we will explore the basics of image processing using OpenCV and its application to image analysis.
Understanding OpenCV
OpenCV is a library of programming functions mainly aimed at real-time computer vision.
Originally developed by Intel, it is now supported by Willow Garage and Itseez.
The library is open source and available for multiple programming languages, including Python, C++, and Java.
OpenCV provides a wide range of functionalities, including image processing, video capture, and machine learning.
Installation of OpenCV
Before diving into image processing, you’ll need to install OpenCV on your system.
If you’re using Python, you can easily install it using pip by executing the following command:
“`
pip install opencv-python
“`
Once installed, you can begin utilizing OpenCV’s vast array of functions to manipulate images.
Basics of Image Processing
Image processing involves manipulating and analyzing digital images to improve their quality or extract useful information.
Here are some basic operations you can perform with OpenCV:
Reading and Displaying Images
The first step in image processing is loading an image and displaying it.
With OpenCV, you can easily read an image from your filesystem using the `cv2.imread()` function and display it using `cv2.imshow()`.
“`python
import cv2
# Load the image
image = cv2.imread(‘example.jpg’)
# Display the image
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Image Resizing
Resizing is an essential operation that allows you to adjust the dimensions of your image.
This is particularly useful when you need to fit an image to a specific size or reduce its load time.
“`python
# Resize the image to half its original size
resized_image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
cv2.imshow(‘Resized Image’, resized_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Converting to Grayscale
In many image processing tasks, you may need to convert a colored image into a grayscale image.
This reduces the complexity of the image and speeds up the processing time.
“`python
# Convert image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow(‘Grayscale Image’, gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Blurring an Image
Blurring or smoothing an image reduces noise and detail.
It’s useful for highlighting significant regions of an image or preparing it for further processing.
“`python
# Apply a Gaussian blur
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
cv2.imshow(‘Blurred Image’, blurred_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Application to Image Analysis
Once you’ve mastered basic image processing operations, you can move on to more complex tasks like image analysis.
OpenCV provides numerous tools for identifying and analyzing specific features in an image.
Edge Detection
Edge detection is an image processing technique used to identify the boundaries within images.
The Canny edge detector is a popular algorithm provided by OpenCV for this purpose.
“`python
# Detect edges using the Canny algorithm
edges = cv2.Canny(image, 100, 200)
cv2.imshow(‘Edges’, edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Contour Detection
Contours are curves joining all the continuous points along a boundary with the same color or intensity.
OpenCV’s `findContours()` function can be used to detect contours in an image, which is useful for shape analysis and object detection.
“`python
# Find contours
contours, hierarchy = cv2.findContours(gray_image, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# Draw the contours
contour_image = cv2.drawContours(image.copy(), contours, -1, (0, 255, 0), 3)
cv2.imshow(‘Contours’, contour_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Histogram Analysis
A histogram represents the distribution of pixel intensities in an image.
By analyzing histograms, you can gain insights into the intensity distribution, which aids in image enhancement and segmentation.
“`python
# Calculate and plot the histogram
histogram = cv2.calcHist([gray_image], [0], None, [256], [0, 256])
import matplotlib.pyplot as plt
plt.plot(histogram)
plt.title(‘Histogram’)
plt.xlabel(‘Pixel Intensity’)
plt.ylabel(‘Frequency’)
plt.show()
“`
Conclusion
OpenCV is a versatile library that provides powerful tools for image processing and analysis.
By understanding the basics of image manipulation and analysis using OpenCV, you can create sophisticated computer vision applications.
This guide has introduced you to fundamental concepts and functions in OpenCV, which can be further explored for more advanced image processing tasks.
With practice and experimentation, you can harness the power of OpenCV to enhance your image processing projects and applications.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)