- お役立ち記事
- Basics of image processing using Python and machine learning programming practice course
Basics of image processing using Python and machine learning programming practice course
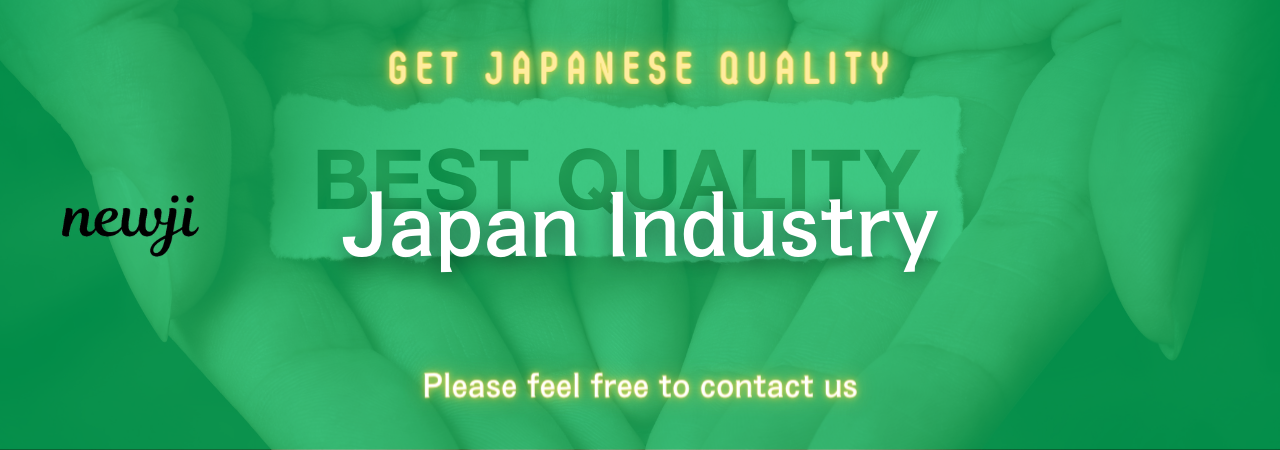
目次
Introduction to Image Processing
Image processing is a field of computer science dedicated to analyzing and manipulating digital images to extract useful information or enhance visual quality.
It involves algorithms and techniques that can perform various tasks, such as object recognition, image compression, and color enhancement.
With the advent of machine learning, image processing has seen significant advancements, enabling more complex and accurate image analysis.
Why Python for Image Processing?
Python is a popular programming language for image processing due to its simplicity and the extensive libraries and frameworks available.
Libraries such as OpenCV, PIL (Pillow), and scikit-image offer a wide range of tools to perform image manipulation tasks efficiently.
Python’s integration with machine learning libraries like TensorFlow and PyTorch makes it an excellent choice for implementing intelligent image processing solutions.
Setting Up Your Python Environment
Before diving into image processing tasks, it is essential to set up a suitable Python environment.
You’ll need to install Python if it’s not already on your system.
Download it from the official Python website and follow the installation instructions.
Once Python is installed, use a package manager like pip to install the necessary libraries for image processing and machine learning.
Installing Essential Libraries
For basic image processing, you will primarily use the following Python libraries:
– **OpenCV**: A comprehensive computer vision library that provides a range of functionalities to manipulate images and videos.
Install it by running `pip install opencv-python`.
– **PIL (Pillow)**: A friendly fork of the original Python Imaging Library (PIL) that provides image manipulation capabilities.
Install it by running `pip install Pillow`.
– **NumPy**: A fundamental library for numerical operations in Python that heavily supports image processing activities.
Install it by running `pip install numpy`.
– **Matplotlib**: A plotting library that can visualize images and various data plots useful in image processing.
Install it by running `pip install matplotlib`.
– **scikit-image**: A collection of algorithms for image processing, building on the capabilities of NumPy and SciPy.
Install it by running `pip install scikit-image`.
Basics of Image Processing
Image processing can be broken down into a series of steps that allow for manipulation and analysis of digital images.
Here, we will cover the basics that you can perform using Python.
Reading and Displaying Images
The first step in image processing is to read an image file and display it.
You can use OpenCV or PIL for this purpose.
Here’s an example using OpenCV to read and display an image:
“`python
import cv2
# Read image
image = cv2.imread(‘path_to_your_image.jpg’)
# Display image
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Similarly, using PIL for the same task:
“`python
from PIL import Image
# Read image
image = Image.open(‘path_to_your_image.jpg’)
# Display image
image.show()
“`
Image Transformation Techniques
Several image transformation techniques are fundamental in image processing:
– **Grayscale Conversion**: Simplifies image processing by reducing the image to a single color channel.
“`python
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
“`
– **Resizing Images**: Useful when working with uniform image dimensions for machine learning models.
“`python
resized_image = cv2.resize(image, (width, height))
“`
– **Image Rotation**: Can adjust the angle of an image for better alignment or augmentation purposes.
“`python
rows, cols = image.shape[:2]
rotation_matrix = cv2.getRotationMatrix2D((cols/2, rows/2), angle, 1)
rotated_image = cv2.warpAffine(image, rotation_matrix, (cols, rows))
“`
Image Filtering and Enhancement
You can enhance image quality or extract significant features using filters and image enhancement techniques:
– **Blurring and Smoothing**: Reduces image noise and details for a clean look or as a pre-processing step.
“`python
blurred_image = cv2.GaussianBlur(image, (5, 5), 0)
“`
– **Edge Detection**: Highlights the boundaries within an image, useful for object detection.
“`python
edges = cv2.Canny(image, threshold1, threshold2)
“`
Introduction to Machine Learning in Image Processing
Machine learning techniques have considerably improved image processing tasks by enabling intelligent image analysis.
Using Pre-trained Models
Pre-trained models, like those available in TensorFlow Hub or PyTorch Hub, can be used for tasks such as image classification:
“`python
import tensorflow as tf
from tensorflow.keras.applications import VGG16
model = VGG16(weights=’imagenet’)
“`
These models have been trained on large datasets and can easily generalize to similar tasks with minimal adjustments.
Building Custom Models
Building your custom model allows for more flexibility and control, especially for specific problem domains.
You can use libraries like TensorFlow or PyTorch to define, train, and optimize neural networks tailored to your needs.
“`python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Conv2D, MaxPooling2D, Flatten
model = Sequential([
Conv2D(32, (3, 3), activation=’relu’, input_shape=(height, width, channels)),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation=’relu’),
Dense(number_of_classes, activation=’softmax’)
])
“`
Training typically involves feeding your model with labeled image data, allowing it to learn patterns and features for successful classification or detection.
Conclusion
Image processing is a crucial aspect of modern computing, enabling various innovative applications, from simple image enhancements to complex artificial intelligence solutions.
Python serves as a powerful tool in this domain, thanks to its rich ecosystem of libraries that support image manipulation and machine learning.
By understanding the basics and practicing these techniques, you can build a strong foundation to tackle more advanced image processing challenges.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)