- お役立ち記事
- Basics of Python programming and effective use of libraries
月間77,185名の
製造業ご担当者様が閲覧しています*
*2025年2月28日現在のGoogle Analyticsのデータより
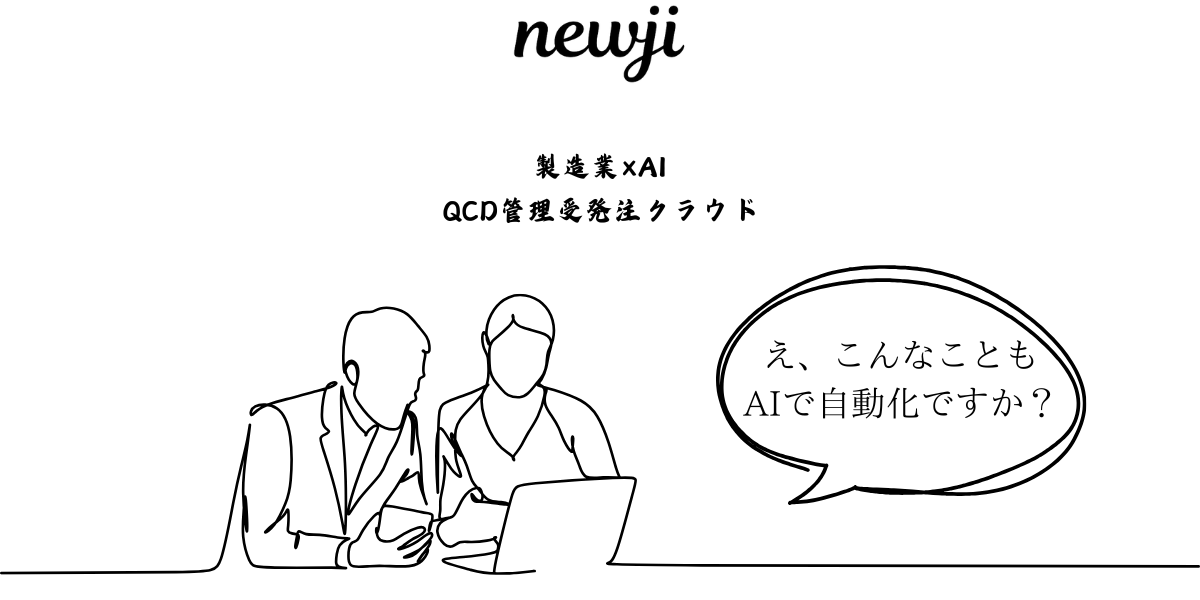
Basics of Python programming and effective use of libraries
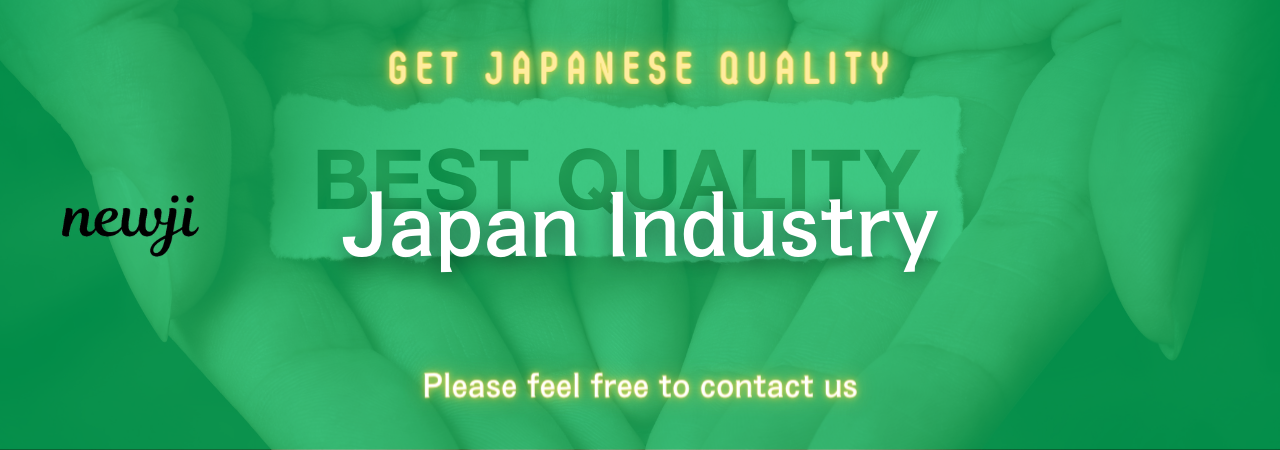
Python has rapidly become one of the most popular programming languages in the world.
Its simplicity and readability make it a favorite among beginners, while its versatility and robust ecosystem of libraries make it indispensable for seasoned developers.
In this article, we’ll explore the basics of Python programming and delve into the effective use of libraries that enhance its functionality.
目次
Getting Started with Python
Python is an interpreted, high-level, general-purpose programming language.
Its easy-to-read syntax means that new programmers can quickly get started and develop functional scripts or programs.
Setting Up Python
To begin programming in Python, you need to have Python installed on your system.
You can download the latest version from Python’s official website.
Installation is straightforward, with options for Windows, macOS, and Linux.
Once installed, you can use the Python interactive shell or any of a variety of integrated development environments (IDEs) like PyCharm, Visual Studio Code, or Jupyter Notebook to write your programs.
Basic Syntax
Python’s syntax is straightforward and often described as ‘executable pseudocode.’
Here are some basic concepts:
– **Variables and Data Types**: Python uses dynamic typing, meaning you don’t need to declare a variable type.
Common data types include integers, floats, strings, and booleans.
– **Loops and Conditionals**: Python uses straightforward syntax for loops (for, while) and conditionals (if, elif, else).
– **Functions**: Define functions using the `def` keyword, which can take parameters and return results using the `return` statement.
– **Indentation**: Python uses indentation to define block structure instead of braces, making clean and readable code.
For example, a simple Python code snippet to print “Hello, World!” would look like:
“`python
def greet():
print(“Hello, World!”)
greet()
“`
Diving Deeper with Python Libraries
One of Python’s key advantages is its extensive library ecosystem.
These libraries can significantly accelerate development by providing pre-written code for a wide range of tasks.
Here are some of the most commonly used libraries across different domains.
NumPy and Pandas for Data Manipulation
NumPy is useful for numerical computations, making it a staple for scientists and engineers.
It provides support for multidimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Pandas, built on top of NumPy, is a powerful library for data manipulation and analysis.
It provides data structures like Series and DataFrames, which simplify data handling and enable complex data operations.
“`python
import numpy as np
import pandas as pd
# Creating a NumPy array
array = np.array([1, 2, 3, 4, 5])
# Creating a Pandas DataFrame
data = {‘Name’: [‘John’, ‘Alice’, ‘Bob’], ‘Age’: [25, 30, 22]}
df = pd.DataFrame(data)
print(array)
print(df)
“`
Matplotlib and Seaborn for Data Visualization
Visualizing data is crucial for understanding patterns and trends.
Matplotlib is a plotting library that enables the creation of static, interactive, and animated visualizations in Python.
For more advanced statistical plotting, Seaborn is built on top of Matplotlib and provides a high-level interface for drawing attractive and informative graphics.
“`python
import matplotlib.pyplot as plt
import seaborn as sns
# Plotting with Matplotlib
x = [1, 2, 3, 4, 5]
y = [5, 7, 8, 5, 2]
plt.plot(x, y)
plt.show()
# Plotting with Seaborn
tips = sns.load_dataset(“tips”)
sns.scatterplot(data=tips, x=”total_bill”, y=”tip”)
plt.show()
“`
Scikit-Learn for Machine Learning
Machine learning has seen wide adoption, and Scikit-learn is a go-to library in Python.
It provides simple and efficient tools for data mining and data analysis, built on NumPy, SciPy, and Matplotlib.
Scikit-learn includes a range of supervised and unsupervised learning algorithms, alongside tools for model evaluation, preprocessing, and cross-validation.
“`python
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
# Train a model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Evaluate the model
predictions = model.predict(X_test)
print(‘Accuracy:’, accuracy_score(y_test, predictions))
“`
Flask and Django for Web Development
Python’s reach extends beyond data science into web development, where libraries like Flask and Django are popular.
Flask is a lightweight and micro web framework, great for small applications or services.
Django, on the other hand, is a high-level framework that encourages rapid development and clean, pragmatic design, suitable for large-scale applications.
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
Best Practices for Using Libraries Effectively
While libraries enhance Python’s functionality, using them effectively is crucial.
Here are some best practices:
– **Read the Documentation**: Understanding a library’s documentation can save you time and help you utilize its features fully.
– **Stay Updated**: Libraries frequently update to improve features and security.
Ensure you’re using the latest version to benefit from these updates.
– **Use Virtual Environments**: Isolate dependencies in a virtual environment using `venv` or `virtualenv`.
This helps avoid version conflicts and ensures reproducible setups.
– **Write Modular Code**: Organize your code into functions and modules to make it reusable and easier to maintain.
– **Benchmark and Optimize**: Libraries can sometimes add overhead.
If performance is critical, use profilers to identify bottlenecks and optimize your code accordingly.
Understanding the basic principles of Python programming along with the strategic use of libraries can greatly enhance your productivity and expand the range of projects you can tackle.
With practice, you can harness the power and versatility of Python to develop everything from simple scripts to large-scale applications.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)