- お役立ち記事
- Basics of Python programs and application and practice to efficient Excel data processing
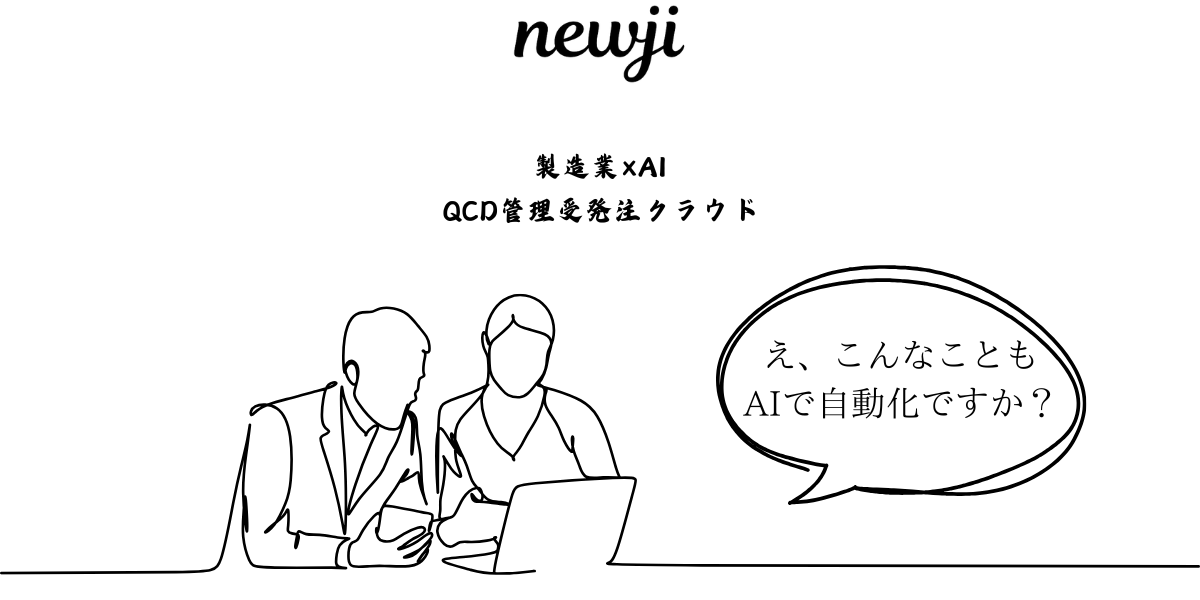
Basics of Python programs and application and practice to efficient Excel data processing
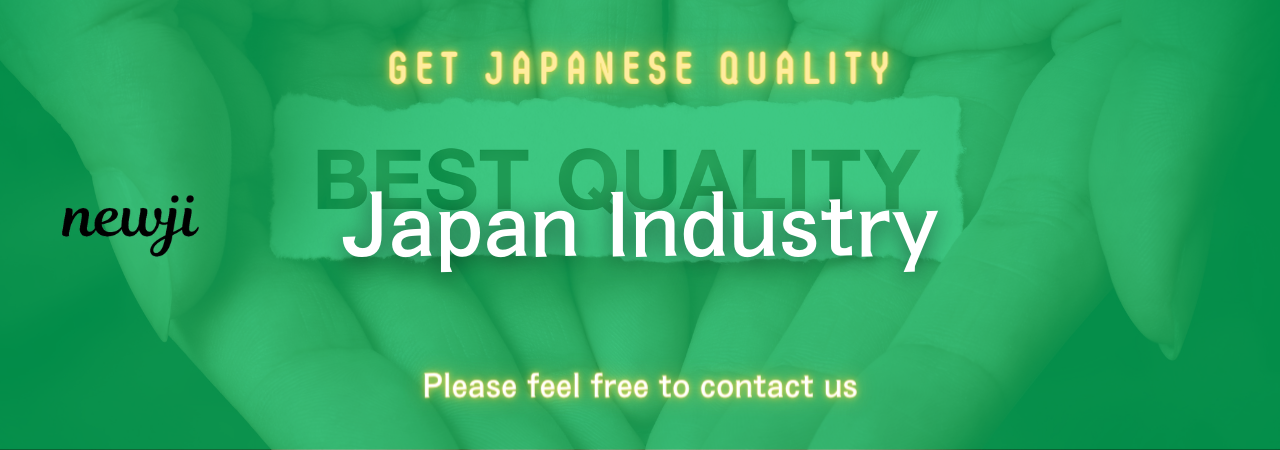
目次
Introduction to Python Programming
Python is a versatile and popular programming language, recognized for its simplicity and readability, making it an excellent choice for both beginners and experienced developers.
It is widely used in web development, data analysis, artificial intelligence, scientific computing, and more.
In this article, we will delve into the basics of Python programming and explore how it can be applied to efficiently process Excel data.
Why Learn Python?
Python’s syntax is easy to learn and understand, which makes it a preferred choice for many people starting their programming journey.
It supports different programming paradigms, including procedural, object-oriented, and functional programming.
Furthermore, Python has an extensive standard library and a large ecosystem of third-party modules, facilitating a range of applications.
For data processing and analysis, Python provides several powerful libraries such as Pandas, NumPy, and Matplotlib, which enhance its capabilities.
These allow data scientists and analysts to handle vast amounts of data and extract meaningful insights without complex coding.
Basics of Python Programming
Python Syntax
The syntax of Python is straightforward and aims to improve code readability.
Here’s a simple example of a Python program that prints “Hello, World!” to the console:
“`python
print(“Hello, World!”)
“`
Notice how there are no complicated commands or semicolons required.
Python uses indentation to define blocks of code, which replaces the need for braces as seen in languages like C++ or Java.
Variables and Data Types
Python is dynamically typed, meaning you don’t have to declare the type of a variable when you create it.
Here’s how you can declare variables and assign values:
“`python
name = “Alice”
age = 25
height = 5.5
“`
In this example, `name` is a string, `age` is an integer, and `height` is a float.
These are some of the basic data types available in Python.
Control Structures
Python includes common control structures such as conditional statements and loops.
For instance, to execute code based on a condition, use an if statement:
“`python
if age > 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
Python also supports loops for iterating over sequences, like lists or strings, using `for` or `while` loops:
“`python
for i in range(5):
print(i)
“`
This loop will print numbers from 0 to 4.
Functions in Python
Functions in Python are declared using the `def` keyword.
They help break the code into modular sections and enhance reusability.
Here’s an example of a simple function:
“`python
def greet(name):
return f”Hello, {name}!”
“`
To call the function, simply pass the argument:
“`python
print(greet(“Alice”))
“`
Processing Excel Data with Python
Python’s ability to process and analyze data efficiently is largely due to powerful libraries like Pandas, openpyxl, and xlrd.
These libraries provide tools for loading, manipulating, and saving Excel files, making Python a popular choice for data analysts dealing with spreadsheets.
Introducing Pandas
Pandas is a fast, powerful, and flexible data analysis library built on top of the NumPy library.
It’s particularly useful for data manipulation tasks and supports reading and writing data between various formats, including Excel.
To get started, you’ll need to install Pandas and its dependencies:
“`bash
pip install pandas
“`
Loading Excel Data
With Pandas installed, you can load Excel spreadsheets into a Pandas DataFrame, a two-dimensional data structure with labeled axes, similar to a table in a database.
Here’s a simple example of how to read an Excel file:
“`python
import pandas as pd
data = pd.read_excel(“data.xlsx”, sheet_name=”Sheet1″)
“`
This code imports Pandas and loads the specified Excel sheet into a DataFrame object called `data`.
Manipulating Data
Once your data is loaded, Pandas offers a wealth of methods to explore and manipulate it.
For instance, you can display the first few rows of the DataFrame using:
“`python
print(data.head())
“`
To filter rows based on a condition:
“`python
filtered_data = data[data[“age”] > 18]
“`
This example selects rows where the `age` column is greater than 18.
Writing Data Back to Excel
After processing your data, you may want to save it back to an Excel file.
Pandas makes this easy with the `to_excel` method:
“`python
filtered_data.to_excel(“filtered_data.xlsx”, index=False)
“`
Here, we’ve saved the filtered data into a new Excel file, excluding the index from the DataFrame.
Conclusion
Python is a powerful tool for efficiently processing Excel data, thanks to its readable syntax and robust libraries like Pandas.
By learning the basics of Python programming and how to apply it to data manipulation tasks, you can significantly enhance your ability to handle and analyze large datasets.
Whether you’re a novice programmer or an experienced developer, Python’s extensive ecosystem provides the tools and resources needed to streamline your data processing workflows and drive impactful insights.
Embrace the power of Python, and you’ll find yourself well-equipped to tackle a wide range of data-related challenges efficiently and effectively.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)