- お役立ち記事
- Basics of supervised machine learning, overfitting suppression method, and Python implementation
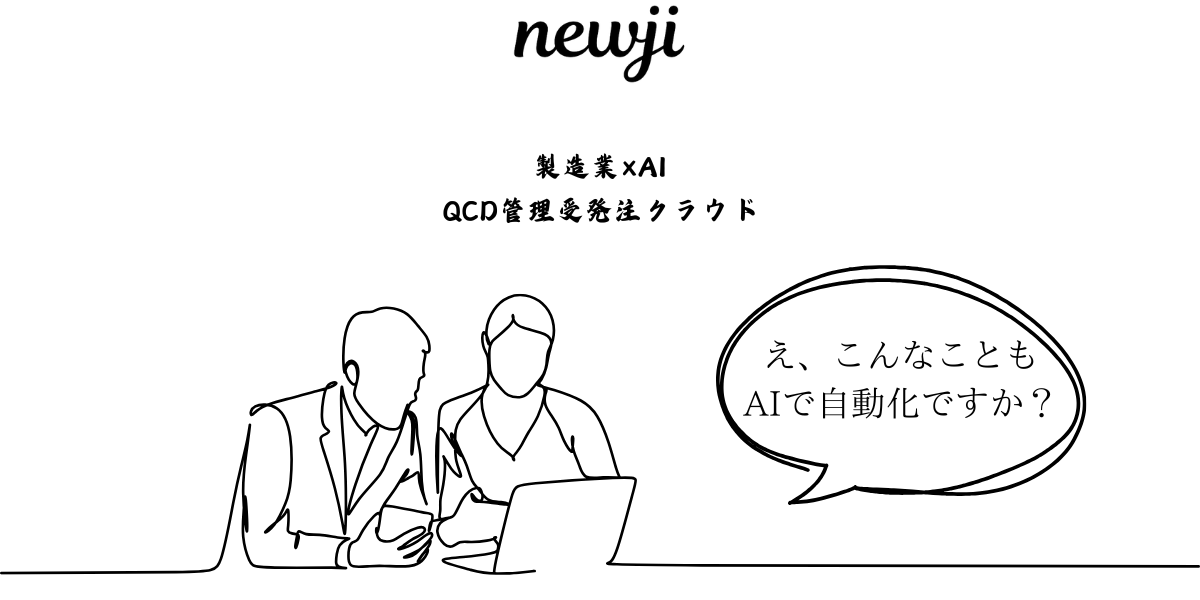
Basics of supervised machine learning, overfitting suppression method, and Python implementation
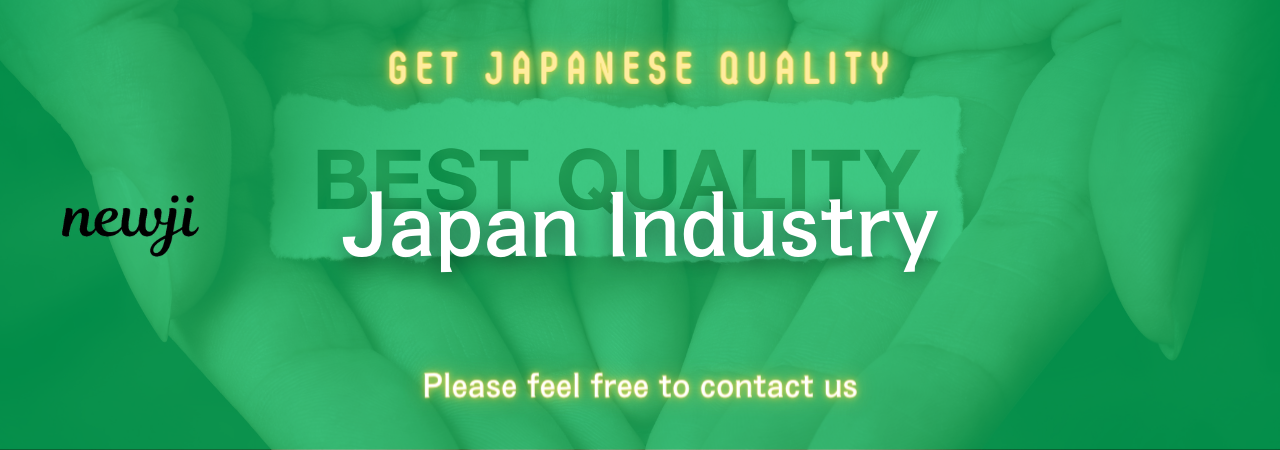
目次
Understanding Supervised Machine Learning
Supervised machine learning is a subfield of artificial intelligence that involves training models using labeled data.
In this approach, the model learns to map input data to corresponding output labels, allowing it to make predictions on new, unseen data.
The process is akin to teaching a child by example.
We provide the algorithm with descriptive data (inputs) and the correct answers (outputs), and it learns to generalize from this information.
There are two primary types of tasks in supervised learning: classification and regression.
Classification involves predicting categorical labels, such as determining whether an email is spam or not.
Regression, on the other hand, deals with predicting continuous values, like forecasting house prices based on various features.
Key Steps in Supervised Learning
To effectively use supervised learning, several steps are crucial:
1. **Data Collection**: Gather a labeled dataset relevant to the problem you’re trying to solve.
2. **Data Preprocessing**: Clean and prepare your data, dealing with missing values and scaling features for improved performance.
3. **Model Selection**: Choose an appropriate algorithm based on the nature of your problem, such as linear regression for continuous data or decision trees for classification tasks.
4. **Training**: Feed the labeled data into the model and use it to learn the underlying patterns.
5. **Evaluation**: Assess the model’s performance using metrics such as accuracy for classification or mean squared error for regression.
6. **Hyperparameter Tuning**: Optimize your model by adjusting parameters to improve its performance.
7. **Prediction**: Use the trained model to make predictions on new, unseen data.
Challenges in Supervised Learning: Overfitting
A major challenge in supervised learning is overfitting.
This occurs when a model learns the training data too well, capturing noise and details that don’t generalize to new data.
As a result, the model performs exceptionally on the training dataset but poorly on unseen data.
Overfitting typically happens with overly complex models that have too many parameters relative to the amount of training data.
These models can fit almost any dataset, but they fail to generalize beyond it.
Consider it like memorizing the answers to a practice test rather than understanding the material thoroughly.
Indicators of Overfitting
1. **High Training Accuracy, Low Test Accuracy**: When the model performs substantially better on the training data compared to test data, it suggests overfitting.
2. **Complexity**: The model complexity outweighs the simplicity of the problem, leading to fitting noise in the data.
3. **Learning Curves**: A large gap between training and validation performance curves indicates that the model is not generalizing well.
Strategies to Prevent Overfitting
Several techniques can help mitigate overfitting:
1. **Cross-Validation**: Use techniques like k-fold cross-validation to ensure the model generalizes well across different subsets of data.
2. **Simplifying the Model**: Use simpler models with fewer parameters to reduce the risk of fitting noise.
3. **Pruning**: In decision trees, prune unnecessary branches to simplify the model without compromising performance.
4. **Regularization**: Add regularization terms, such as L1 and L2, to the loss function to discourage overly complex models.
5. **Dropout**: In neural networks, apply dropout during training to randomly ignore certain neurons, reducing reliance on any specific feature.
6. **Early Stopping**: Halt training when validation performance ceases to improve, preventing further fitting to the noise.
7. **Data Augmentation**: Increase the size of your training data using techniques like rotation, translation, or adding noise to images.
Python Implementation of a Supervised Learning Model
Implementing a supervised learning model in Python can be done efficiently with the help of libraries like scikit-learn.
Below is a simple implementation of a linear regression model to demonstrate supervised learning:
“`python
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Generate synthetic data
np.random.seed(0)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
# Split into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a linear regression model
model = LinearRegression()
# Train the model on the training data
model.fit(X_train, y_train)
# Make predictions on the test data
y_pred = model.predict(X_test)
# Evaluate the model’s performance
mse = mean_squared_error(y_test, y_pred)
print(f’Mean Squared Error: {mse}’)
“`
This code performs the following tasks:
1. **Data Generation**: Creates synthetic data for demonstration purposes.
2. **Data Splitting**: Divides the data into training and testing sets, ensuring the model is evaluated on unseen data.
3. **Model Creation**: Initializes a linear regression model.
4. **Training**: Fits the model to the training dataset.
5. **Prediction and Evaluation**: Predicts the outcomes for the test data and evaluates the model with mean squared error.
Conclusion
Supervised machine learning is a powerful tool with applications in various domains, from medicine to finance.
Understanding the fundamental concepts, such as the difference between classification and regression, and being aware of challenges like overfitting, are crucial for successful model building.
By employing techniques to prevent overfitting and leveraging Python libraries like scikit-learn, practitioners can build robust models that make accurate predictions on real-world data.
As you continue to explore machine learning, remember that practice and experimentation are key to mastering the concepts and fine-tuning your models for optimal performance.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)