- お役立ち記事
- Fundamentals of Go language programming and application to application development
Fundamentals of Go language programming and application to application development
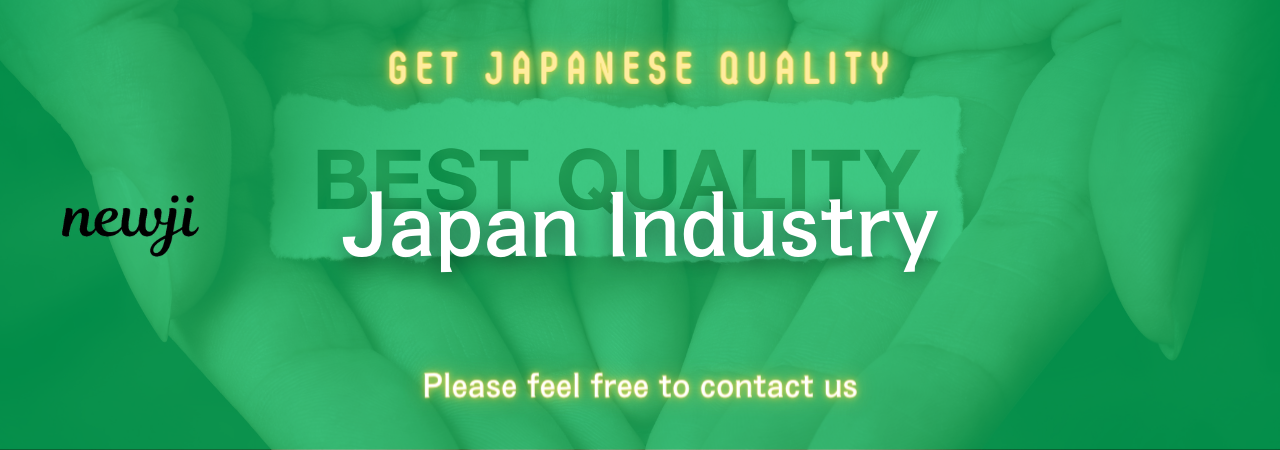
目次
What is Go Language?
Go, also known as Golang, is a statically typed, compiled programming language designed by Google.
It was first introduced in 2009, and it has gained a lot of popularity due to its simplicity, efficiency, and robustness.
Go is often compared to C in terms of performance but comes with modern language features that make it easier to use.
It is known for its efficient handling of concurrency, which is the ability to execute multiple processes simultaneously.
Key Features of Go Language
Simplicity and Efficiency
Go is designed with simplicity in mind.
Its syntax is clean and straightforward, making it easy for developers to learn and use.
Despite being simple, Go does not compromise on performance.
It compiles quickly into efficient machine code, providing performance comparable to low-level programming languages.
Concurrency Support
One of Go’s most praised features is its excellent support for concurrency.
Goroutines in Go allow functions to run concurrently with other functions.
This makes Go particularly well-suited for server-side applications and handling numerous network connections.
Garbage Collection
Go comes with an automatic garbage collection feature.
This means that Go automatically removes unused memory allocations and prevents memory leaks.
This is crucial for long-running applications, ensuring they use resources efficiently.
Strong Standard Library
The standard library in Go is rich and comprehensive, providing built-in support for many tasks including I/O operations, networking, cryptography, and more.
This extensive library reduces the need for external dependencies, streamlining the development process.
Cross-Platform
Go is designed to be cross-platform.
You can write code on one operating system and compile it to run on another.
This feature makes Go highly versatile and adaptable for diverse projects.
Application of Go Language in Development
Web Development
Go’s powerful standard library and fast performance make it an excellent choice for web development.
It includes packages for HTTP, URL handling, HTML templates, and more.
With frameworks like Gin and Echo, developers can create fast, robust, and scalable web applications.
Cloud Services
Go is widely used in cloud computing due to its concurrency model and efficient performance.
It’s employed in developing cloud-native applications and microservices.
Google’s App Engine and popular platforms like Docker and Kubernetes are built using Go, highlighting its significance in cloud service development.
Network Programming
The rich set of networking libraries and support for concurrent processes make Go ideal for network programming.
Developers can create efficient network servers, proxy servers, and other network infrastructure with ease.
Command-Line Tools
Go is often used to develop command-line tools and utilities.
Its straightforward syntax, fast performance, and cross-platform nature make it a natural choice for building tools that need to run efficiently on different systems.
Getting Started with Go
Installing Go
Getting started with Go is simple.
Visit the official Go website and download the installer package suitable for your operating system.
Once installed, you can verify the installation by running `go version` in your command line.
Writing a Simple Go Program
A simple Go program typically starts with defining a package, followed by importing required libraries and declaring a `main` function.
Here’s a basic example:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
This program prints “Hello, World!” and serves as a foundation for understanding Go syntax.
Running a Go Program
To run your Go program, save your code in a `.go` file, and use the `go run` command followed by the file name in your terminal.
The Go compiler will execute your code and display the output.
Best Practices in Go Programming
Code Organization
Organize your code into packages for better readability and maintainability.
Place related files in the same package and keep a clean directory structure.
Error Handling
Proper error handling is crucial in Go.
Make use of Go’s built-in error type to handle errors gracefully.
Always check for errors when calling functions that can fail.
Testing
Use Go’s built-in testing framework to write tests for your code.
Automated testing ensures code reliability and ease of maintenance.
Conclusion
Go language programming offers a balanced combination of simplicity, performance, and modern features, which appeals to a wide range of developers and applications.
From web development to cloud-based solutions, Go provides the tools and features needed to build efficient and robust applications.
By following best practices and starting with small projects, developers can harness the full potential of Go in their development endeavors.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)