- お役立ち記事
- Fundamentals of image processing technology using Python, programming, and application to feature extraction and object detection
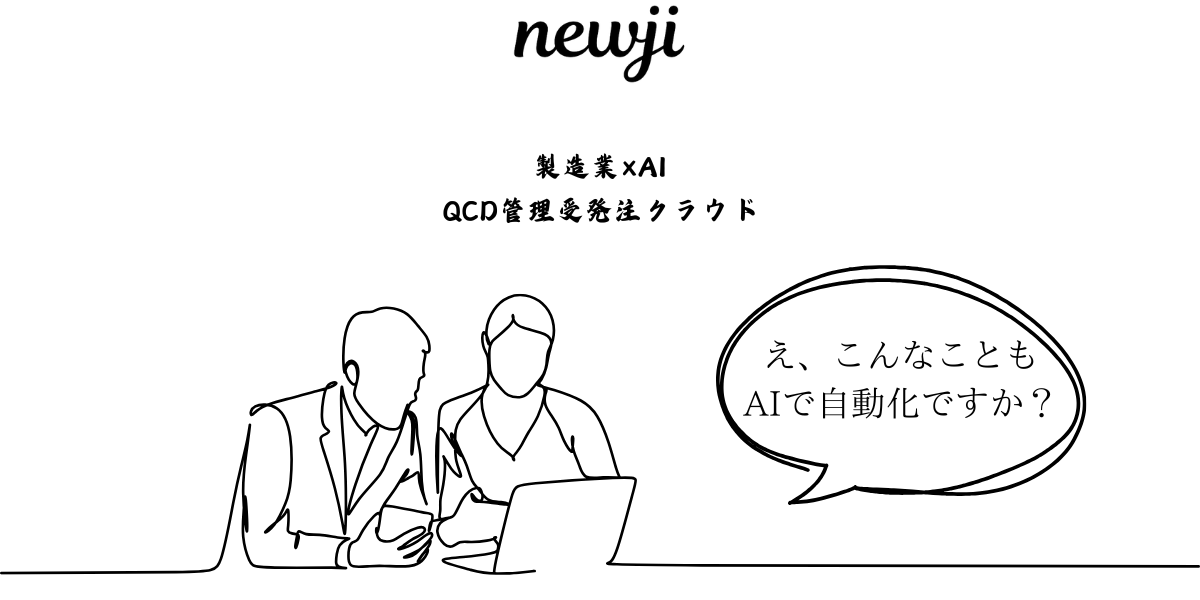
Fundamentals of image processing technology using Python, programming, and application to feature extraction and object detection
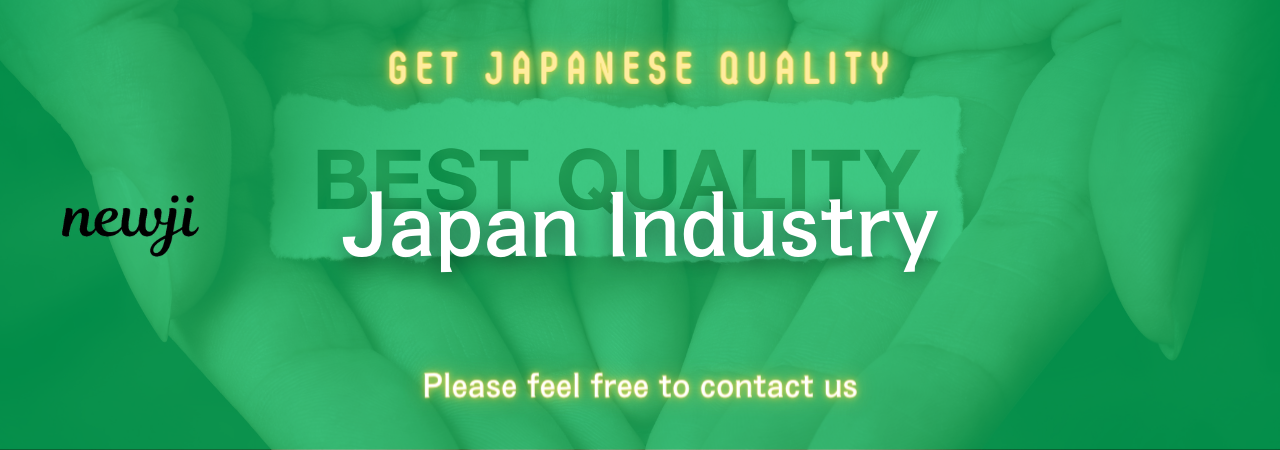
目次
Introduction to Image Processing with Python
Image processing is a critical skill in the field of computer science and data analysis.
With the rise of artificial intelligence and machine learning, understanding image processing can open the door to various applications, such as feature extraction and object detection.
Python, with its simplicity and extensive libraries, has become a popular language for tackling these challenges.
In this article, we will explore the basics of image processing using Python.
We’ll discuss programming techniques and applications that will help you harness the power of this technology.
What is Image Processing?
Image processing refers to the manipulation of an image to produce a desired outcome.
This can involve enhancing the image to improve visual quality or extracting valuable information from the image.
In essence, it’s about teaching computers to “see” and interpret visual data.
There are several tasks that fall under image processing, including:
1. Image Enhancement
Image enhancement involves improving the visual aspects of an image.
This can mean adjusting brightness, contrast, or sharpening details to improve clarity.
2. Feature Extraction
Feature extraction is the process of identifying and highlighting specific characteristics within an image.
For example, an algorithm may detect edges, corners, or textures to understand the content of the image.
3. Object Detection
Object detection involves identifying and locating objects within an image.
This task is often used in applications such as facial recognition, autonomous vehicles, and surveillance systems.
Getting Started with Python for Image Processing
Python provides a plethora of libraries that simplify image processing tasks.
Let’s look at some essential libraries and how you can use them for basic image processing.
1. OpenCV
OpenCV (Open Source Computer Vision Library) is one of the most widely used tools for image and video processing.
It provides a wide range of functionalities, from basic image manipulation to more complex operations like object detection.
To start using OpenCV, you first need to install it:
“`bash
pip install opencv-python
“`
Once installed, you can start using OpenCV for various tasks.
Let’s begin with reading and displaying an image:
“`python
import cv2
# Read an image
image = cv2.imread(‘example.jpg’)
# Display the image
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
2. NumPy
NumPy is a fundamental package for scientific computing with Python.
It is particularly useful in image processing for performing operations on arrays, as images can be represented as multi-dimensional arrays.
You can install NumPy using:
“`bash
pip install numpy
“`
With NumPy, you can perform operations like cropping, rotating, and transforming images:
“`python
import numpy as np
# Crop an image
cropped_image = image[50:150, 50:150]
# Rotate an image
rotated_image = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
“`
3. Matplotlib
Matplotlib is a library for creating static, interactive, and animated visualizations in Python.
It is commonly used alongside NumPy for displaying images.
To install Matplotlib, use:
“`bash
pip install matplotlib
“`
You can display images with Matplotlib for quick plotting:
“`python
import matplotlib.pyplot as plt
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.title(‘Example Image’)
plt.show()
“`
Application of Image Processing in Feature Extraction
Feature extraction is a crucial step in the preprocessing of images.
By extracting essential features, you can make subsequent tasks like classification and recognition more effective.
Edge Detection
Detecting edges in an image can help in identifying objects and shapes.
The Canny Edge Detection algorithm is one of the most popular methods for this purpose:
“`python
edges = cv2.Canny(image, 100, 200)
plt.imshow(edges, cmap=’gray’)
plt.title(‘Edge Detection’)
plt.show()
“`
Contour Detection
Contours are curves that bound objects.
By identifying contours, you can detect the shape of an object within an image:
“`python
contours, _ = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# Draw contours
cv2.drawContours(image, contours, -1, (0, 255, 0), 3)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.title(‘Contour Detection’)
plt.show()
“`
Object Detection with Python
Object detection is a field of image processing that involves identifying and classifying objects within an image.
Using Python, you can perform object detection using pre-trained models like Haar Cascades or leveraging deep learning frameworks.
Using Haar Cascades
Haar cascades are a simple and effective method for object detection, particularly for real-time applications.
They can be trained to detect various objects, such as faces, eyes, and smiles.
Here’s an example of using Haar cascades for face detection:
“`python
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + ‘haarcascade_frontalface_default.xml’)
# Convert to grayscale for detection
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Detect faces
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# Draw rectangles around faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.title(‘Face Detection’)
plt.show()
“`
Conclusion
Image processing with Python is both powerful and accessible due to its rich ecosystem of libraries.
By exploring tools like OpenCV, NumPy, and Matplotlib, you can perform a wide range of image processing tasks, from basic manipulation to advanced feature extraction and object detection.
Understanding image processing not only enhances your programming skills but also equips you with the tools to tackle complex problems in machine learning and computer vision.
As you continue to explore, you’ll find countless opportunities to apply these techniques in real-world applications.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)