- お役立ち記事
- Fundamentals of information security and cryptography, implementation and application using Python
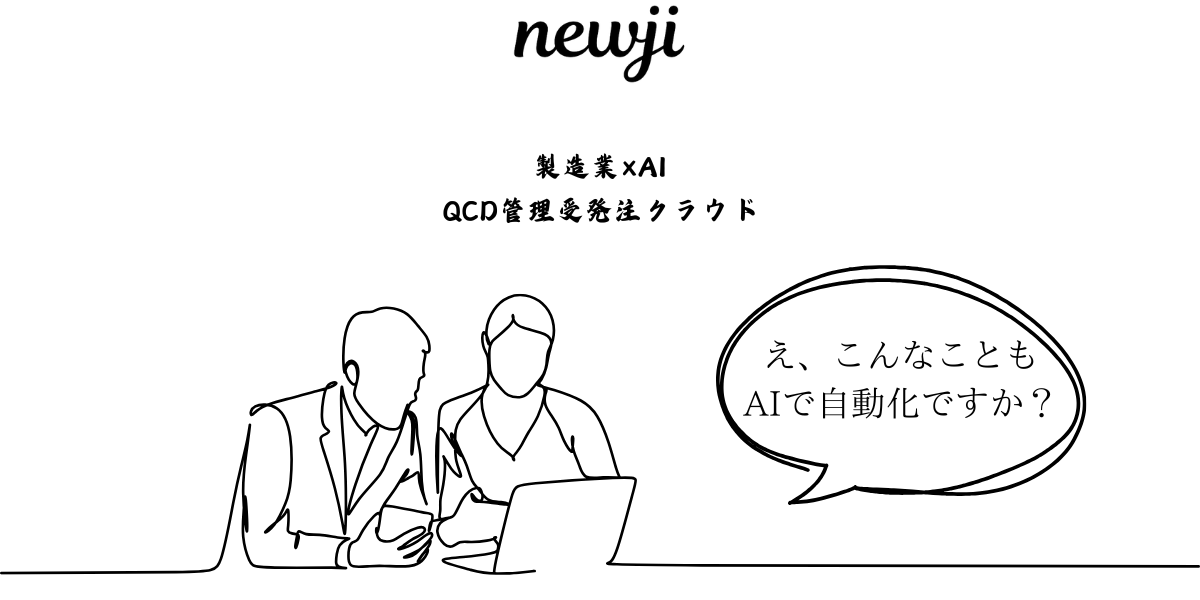
Fundamentals of information security and cryptography, implementation and application using Python
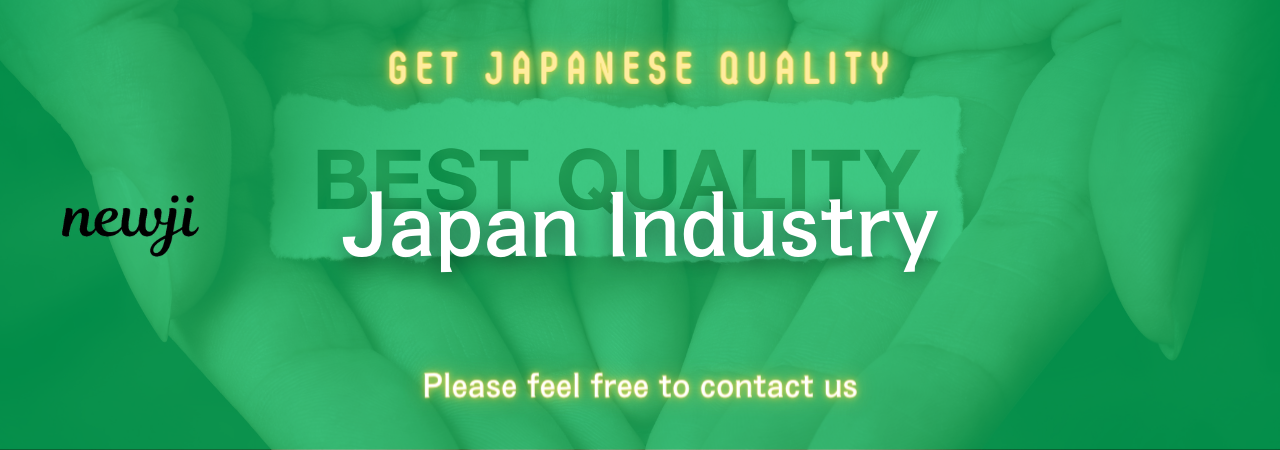
目次
Understanding Information Security
Information security is a critical aspect of modern technology use and deployment.
It involves protecting information and systems from unauthorized access, disclosure, alteration, and destruction.
The fundamental goal of information security is to ensure confidentiality, integrity, and availability of data.
Confidentiality ensures that sensitive information is accessible only to those who have the necessary permissions.
Integrity involves maintaining the accuracy and reliability of data by preventing unauthorized modifications.
Availability ensures that information and resources are accessible to authorized users when needed.
Key Components of Information Security
Information security is a vast field, encompassing multiple disciplines.
These include network security, application security, data security, endpoint security, and identity management, among others.
– **Network Security:** This involves protecting the infrastructure of an organization’s networks by employing various tactics and tools to prevent unauthorized access and misuse.
– **Application Security:** This component focuses on safeguarding applications from vulnerabilities during development and throughout their life cycle.
– **Data Security:** This involves protecting data from unauthorized access or alterations, ensuring only those with the right permissions can handle sensitive data.
– **Endpoint Security:** This aspect focuses on securing end-user devices such as laptops and smartphones from malicious threats.
– **Identity Management:** It involves ensuring that only authorized users have access to the organization’s systems and information using various authentication methods.
Cryptography: Protecting Information
Cryptography is a key component of information security that involves encoding information so that it can only be accessed by someone who knows how to decode it.
This technology is used to protect sensitive information from prying eyes, ensure secure communication, and protect personal and sensitive data.
Basics of Cryptography
Cryptography primarily involves two processes: encryption and decryption.
Encryption is the process of converting plain text into a coded format, known as ciphertext.
Decryption is the reverse process, converting the ciphertext back into plain text.
There are two primary types of cryptographic systems:
– **Symmetric Key Cryptography:** This system uses a single key for both encryption and decryption.
Hence, both the sender and receiver must share the key, which can be a potential security risk if not handled properly.
– **Asymmetric Key Cryptography (Public Key Cryptography):** This system uses a pair of keys – a public key that is shared with everyone and a private key that is kept secret by the owner.
This setup allows users to secure their communications without needing to share a secret key.
Common Cryptography Algorithms
Several algorithms provide the basis for applying cryptography in information security.
Some of the most common ones include:
– **AES (Advanced Encryption Standard):** A symmetric encryption algorithm that is widely used for securing data.
– **RSA (Rivest-Shamir-Adleman):** An asymmetric algorithm commonly used for secure data transmission.
– **SHA (Secure Hash Algorithm):** A family of cryptographic hash functions used to transform data into a fixed-size string of bytes.
These algorithms form the backbone of information security efforts in many electronic systems and are used extensively for securing communications, protecting personal data, and ensuring safe financial transactions.
Implementing Cryptography with Python
Python is a popular language for implementing cryptographic techniques due to its ease of use and rich library support.
The `cryptography` package in Python provides tools for hashing, encryption, and other cryptographic processes.
Setting Up the Cryptography Package
To use cryptography in Python, you need to install the `cryptography` package.
This can be done via pip with the following command:
“`bash
pip install cryptography
“`
Once installed, the package can be imported into your Python script for performing various cryptographic operations.
Example: AES Encryption and Decryption
Below is a simple example of how to perform AES encryption and decryption in Python using the `cryptography` package:
“`python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
import os
# Generate a random key for encryption
key = os.urandom(32) # 256-bit key
# Create a cipher object
cipher = Cipher(algorithms.AES(key), modes.CBC(os.urandom(16)), backend=default_backend())
# Encrypt the data
encryptor = cipher.encryptor()
plaintext = b”This is a secret message.”
ciphertext = encryptor.update(plaintext) + encryptor.finalize()
# Decrypt the data
decryptor = cipher.decryptor()
decrypted_text = decryptor.update(ciphertext) + decryptor.finalize()
print(“Plaintext:”, plaintext)
print(“Decrypted Text:”, decrypted_text)
“`
In this example, a random key is generated for AES encryption and decryption.
The `update()` method is used to encrypt and decrypt data in blocks, and the `finalize()` method ensures any remaining data is processed.
Applications of Cryptography
Cryptography finds applications in multiple areas due to its ability to secure communication and protect data integrity.
Secure Communications
Cryptography is used extensively in securing communications over the internet.
Protocols such as SSL/TLS use cryptographic methods to secure data transmission between web servers and clients.
Digital Signatures and Authentication
Digital signatures use cryptography to validate the authenticity and integrity of a message or document.
This is crucial in securing electronic communications and transactions.
Data Protection
Cryptographic techniques like encryption ensure that sensitive data, such as credit card numbers and personal identity information, remain secret.
This is crucial for financial institutions and e-commerce platforms to protect user data.
Conclusion
Information security and cryptography are foundational elements in safeguarding digital information.
Understanding these concepts and implementing them effectively—using languages like Python—ensures secure transactions and communications, protecting users from unauthorized access and data breaches.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)