- お役立ち記事
- Fundamentals of reinforcement learning, algorithm implementation in Python, and its applications
月間76,176名の
製造業ご担当者様が閲覧しています*
*2025年3月31日現在のGoogle Analyticsのデータより
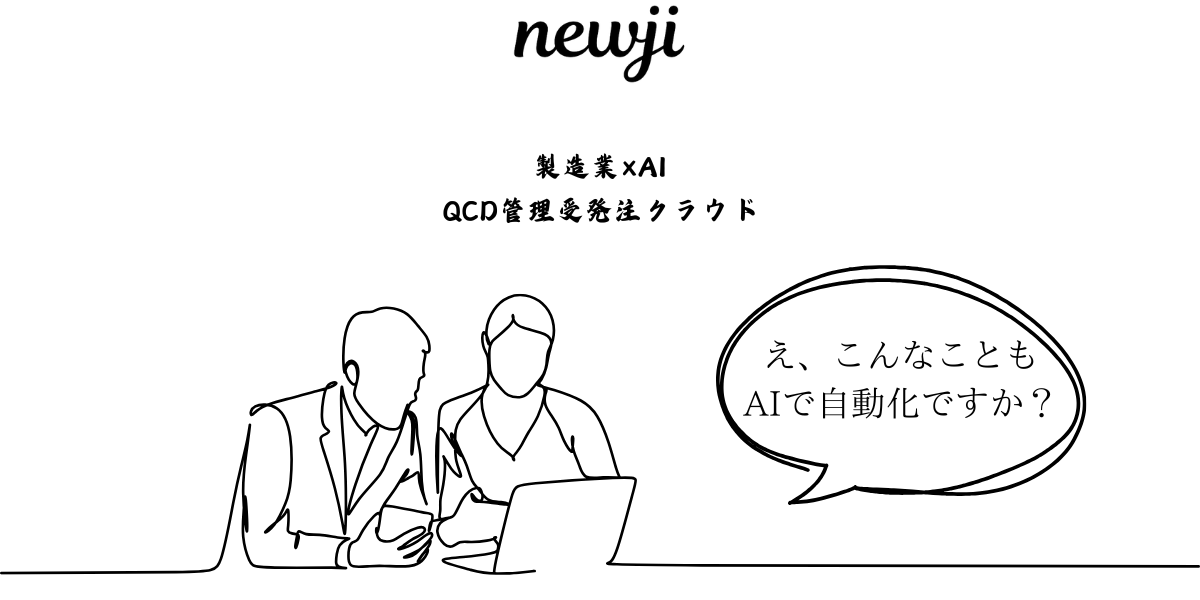
Fundamentals of reinforcement learning, algorithm implementation in Python, and its applications
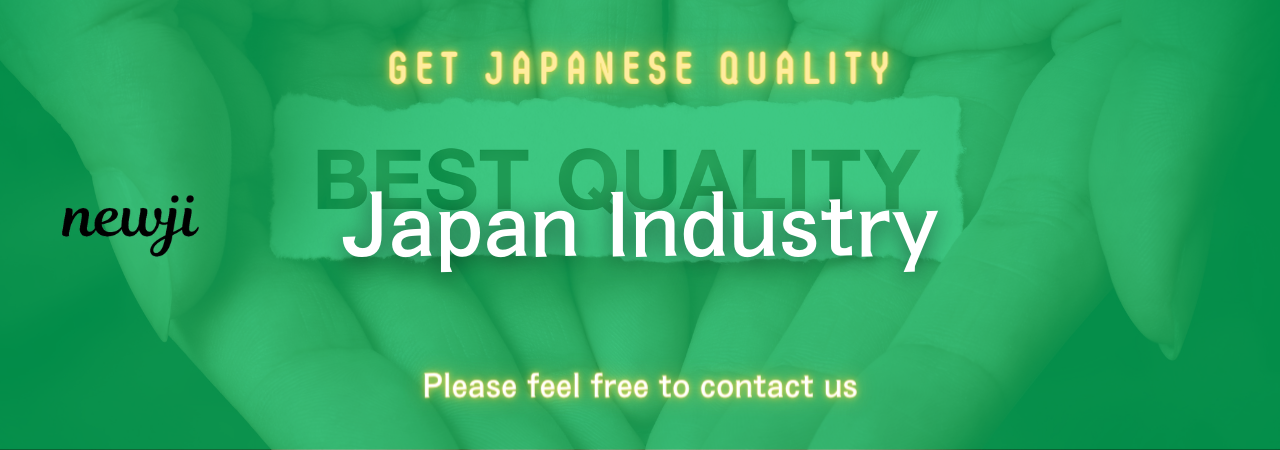
目次
Understanding Reinforcement Learning
Reinforcement learning (RL) is a fascinating field of artificial intelligence that focuses on how agents should take actions in an environment to maximize some notion of cumulative reward.
At the core, it’s about learning from interaction to achieve a long-term objective.
Unlike supervised learning, where the model learns from labeled data, reinforcement learning involves learning from experience and adapting to changes.
Reinforcement learning is inspired by behavioral psychology, where decisions are made based on the idea of receiving rewards and punishments.
An agent explores different actions and learns to associate them with good or bad outcomes.
Think of a robot learning to navigate a maze or a computer program learning to play a game.
The agent’s aim is to develop strategies that increase its reward over time.
Key Concepts in Reinforcement Learning
Reinforcement learning is built upon several key concepts:
– **Agent**: The learner or decision-maker.
– **Environment**: Everything the agent interacts with.
– **State**: A representation of the current situation in the environment.
– **Action**: The set of moves the agent can make.
– **Reward**: The feedback from the environment, which guides the learning process.
– **Policy**: The strategy used by the agent to decide actions based on the current state.
– **Value Function**: A prediction of future rewards expected over the long-term.
Algorithm Implementation in Python
Python is the go-to programming language for implementing reinforcement learning algorithms due to its simplicity and the vast number of available libraries.
We’ll explore a basic reinforcement learning algorithm implementation known as Q-learning.
Q-Learning Explained
Q-learning is one of the simplest and most popular RL algorithms.
It is a model-free algorithm that updates a Q-table to find the optimal action-selection policy.
The Q-table is a matrix where each row represents a state, each column represents an action, and each cell holds a Q-value representing the quality of an action in a particular state.
The Q-value is updated using the formula:
Q(s, a) = Q(s, a) + α [R + γ max Q(s’, a’) – Q(s, a)]
Where:
– s is the current state.
– a is the current action.
– α is the learning rate.
– R is the reward received after transitioning to the new state s’.
– γ is the discount factor, representing the importance of future rewards.
– max Q(s’, a’) is the maximum predicted value for the next state.
Implementing Q-Learning in Python
To implement Q-learning in Python, you need to follow these steps:
1. **Initialize the Q-table**: Create a table with random values or zeros.
2. **Choose an action** in the current state using the ε-greedy policy, which balances exploration and exploitation.
3. **Take the action** and observe the reward and the next state.
4. **Update the Q-value** using the Q-learning formula.
5. **Repeat the process** for a given number of episodes or until convergence.
Here’s a simple implementation of the Q-learning algorithm in Python:
“`python
import numpy as np
import gym
# Initialize environment
env = gym.make(‘FrozenLake-v0’)
# Parameters
epsilon = 0.9 # Exploration-exploitation balance
learning_rate = 0.8
discount_factor = 0.95
num_episodes = 1000
# Initialize Q-table
Q = np.zeros([env.observation_space.n, env.action_space.n])
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
# Choose action
if np.random.uniform(0, 1) < epsilon:
action = env.action_space.sample() # Exploration
else:
action = np.argmax(Q[state, :]) # Exploitation
# Take the action
next_state, reward, done, _ = env.step(action)
# Update Q-table
Q[state, action] = Q[state, action] + learning_rate * (reward + discount_factor * np.max(Q[next_state, :]) - Q[state, action])
# Move to the next state
state = next_state
print("Training completed!")
# Testing the learned policy
state = env.reset()
done = False
steps = 0
while not done:
action = np.argmax(Q[state, :])
state, reward, done, _ = env.step(action)
steps += 1
print(f"Reached goal in {steps} steps!")
```
Applications of Reinforcement Learning
Reinforcement learning has diverse applications across various fields.
Let’s explore some prominent use cases:
Gaming
One of the most well-known applications of reinforcement learning is in gaming.
Algorithms like AlphaGo and AlphaZero have demonstrated superhuman performance by learning complex strategies in games like Go, Chess, and Shogi.
Robotics
In robotics, RL is used to teach robots new skills, like walking, grasping, and navigating complex environments.
By learning from trial and error, robots can adapt to new tasks and improve efficiency.
Finance
In finance, reinforcement learning algorithms are applied to develop trading strategies and optimize portfolios.
These algorithms learn from historical data to make decisions that maximize returns while minimizing risk.
Healthcare
In healthcare, RL is used for personalizing treatment plans, optimizing the allocation of resources, and improving patient outcomes.
For instance, it can help recommend the best treatment strategies for chronic diseases based on individual patient data.
Autonomous Vehicles
Reinforcement learning plays a crucial role in the development of autonomous vehicles.
It helps in path planning, decision-making, and adapting to dynamic environments such as traffic by learning from experiences.
Conclusion
Reinforcement learning is a powerful tool in the realm of artificial intelligence, enabling machines to learn from their environment and improve their actions over time.
Its applications span a wide range of fields, from gaming to healthcare, showcasing its versatility and potential.
By understanding the fundamentals and implementing basic algorithms like Q-learning in Python, you can start exploring this fascinating area of machine learning.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)