- お役立ち記事
- How to implement deep learning with Chainer and its key points
月間77,185名の
製造業ご担当者様が閲覧しています*
*2025年2月28日現在のGoogle Analyticsのデータより
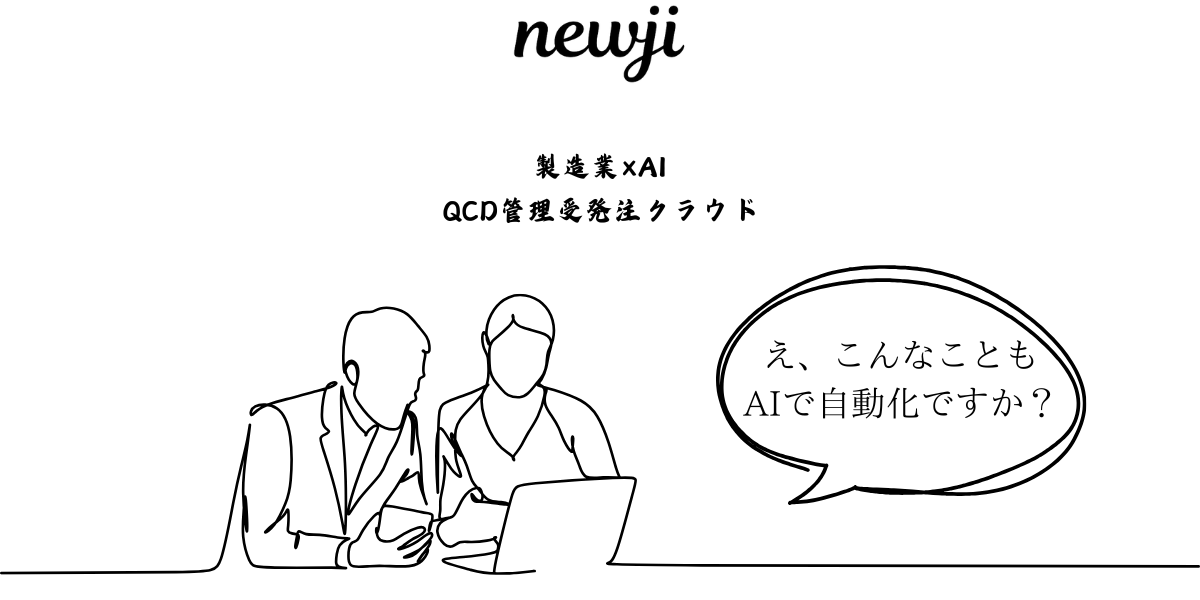
How to implement deep learning with Chainer and its key points
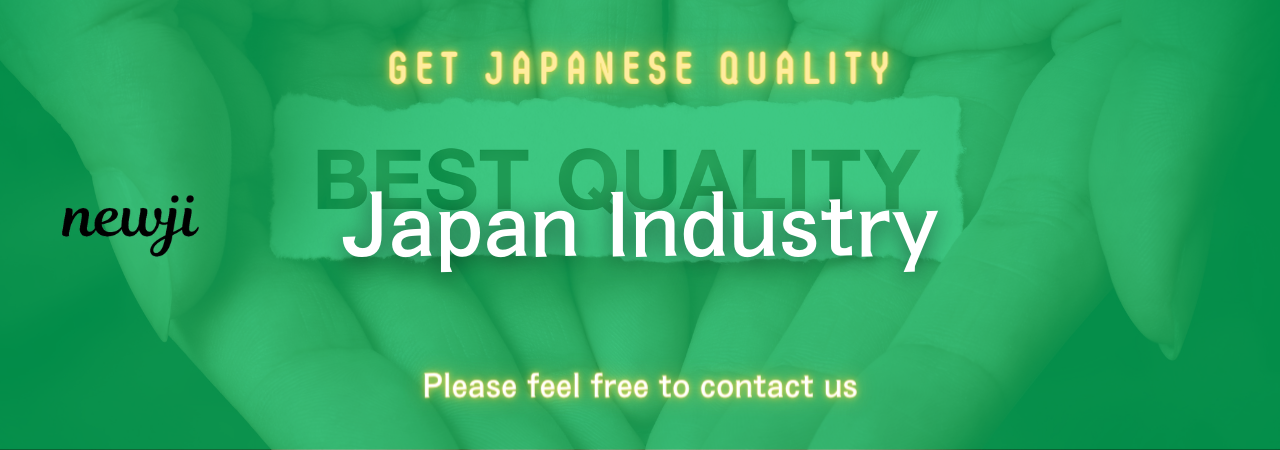
目次
Understanding Deep Learning and Chainer
Deep learning is a subset of machine learning that uses neural networks with many layers, known as deep neural networks, to analyze patterns in data.
It enables computers to perform human-like tasks such as image recognition, language translation, and more by mimicking the way the human brain operates.
To implement deep learning, several libraries and frameworks are available, with Chainer being one of the popular options.
Chainer is an open-source deep learning framework written in Python.
It focuses on facilitating research and prototyping, making it ideal for researchers and developers who want to quickly test and implement new ideas.
Chainer’s unique feature is its define-by-run approach, which enables dynamic computation graphs that are created on the fly, offering flexibility and user-friendliness to developers.
Setting Up Chainer for Deep Learning
Before diving into deep learning implementation with Chainer, you need to set up your environment correctly.
This typically involves installing Python and Chainer itself.
Here’s a step-by-step guide to setting up Chainer:
Step 1: Install Python
Ensure you have Python installed on your system.
Chainer supports Python version 3.5 or above.
You can download Python from its official website and follow the installation instructions specific to your operating system.
Step 2: Use a Virtual Environment
It’s a good practice to use a virtual environment to manage your Python packages.
It helps avoid conflicts between different packages.
You can create a virtual environment by using the ‘venv’ module or ‘virtualenv’ tool.
Activate the virtual environment once it’s created.
Step 3: Install Chainer
With your virtual environment activated, you can install Chainer using pip.
Run the following command:
“`
pip install chainer
“`
If you plan to use GPU for your deep learning tasks, you should also install CuPy, which is Chainer’s GPU support library.
Install it using:
“`
pip install cupy-cudaNN
“`
Replace ‘cudaNN’ with the version number that matches your CUDA version.
Building a Simple Neural Network with Chainer
Now that you’ve set up Chainer, let’s build a simple neural network model to get hands-on experience.
We’ll create a basic feed-forward neural network for image classification using the MNIST dataset, a standard dataset for handwritten digit recognition.
Step 1: Import Libraries
Begin by importing the necessary libraries:
“`python
import chainer
import chainer.functions as F
import chainer.links as L
from chainer import training
from chainer.training import extensions
from chainer.datasets import get_mnist
“`
Step 2: Define the Neural Network Model
Create a subclass of `chainer.Chain` to define the model.
Here’s a simple example:
“`python
class SimpleNN(chainer.Chain):
def __init__(self, n_units, n_out):
super(SimpleNN, self).__init__()
with self.init_scope():
self.l1 = L.Linear(None, n_units)
self.l2 = L.Linear(n_units, n_out)
def forward(self, x):
h1 = F.relu(self.l1(x))
return self.l2(h1)
“`
In this model, `self.l1` and `self.l2` are layer definitions.
The `L.Linear` function creates fully connected neurons, and `F.relu` applies the ReLU activation function.
Step 3: Setup and Train the Model
Next, load the MNIST dataset and set up the trainer:
“`python
train, test = get_mnist()
model = L.Classifier(SimpleNN(100, 10))
optimizer = chainer.optimizers.Adam()
optimizer.setup(model)
train_iter = chainer.iterators.SerialIterator(train, batch_size=128)
test_iter = chainer.iterators.SerialIterator(test, batch_size=128, repeat=False, shuffle=False)
updater = training.StandardUpdater(train_iter, optimizer, device=-1)
trainer = training.Trainer(updater, (20, ‘epoch’), out=’result’)
trainer.extend(extensions.Evaluator(test_iter, model, device=-1))
trainer.extend(extensions.LogReport())
trainer.extend(extensions.PrintReport([‘epoch’, ‘main/loss’, ‘validation/main/loss’, ‘main/accuracy’, ‘validation/main/accuracy’]))
trainer.run()
“`
This code sets up the optimizer (Adam), creates iterators for the training and test datasets, and defines a trainer to manage the training loop.
We extend the trainer to include evaluation and logging during the training process.
Key Points in Implementing Deep Learning with Chainer
Implementing deep learning with Chainer involves several key considerations for optimal performance and flexibility.
Dynamic Graph Creation
Chainer’s define-by-run architecture allows for dynamic computational graphs, which means the graph structure is defined by the actual computations performed.
This flexibility is particularly useful in situations where the network architecture needs to change based on input data.
Easy Prototyping and Debugging
Chainer’s dynamic nature supports easy experimentation with different models and architecture.
This feature makes prototyping fast and debugging much simpler as you can insert print statements or use a debugger to inspect variables or flow of execution.
Effective Use of GPU
To leverage Chainer’s GPU capabilities, ensure that CuPy is installed.
CuPy mimics the NumPy library but allows performing computations on GPUs, significantly accelerating the training processes for larger models and datasets.
High Scalability
Though designed for flexibility, Chainer can scale from single-node setups to distributed environments, making it suitable for large-scale research and production deployments.
Use the `chainer.training` module’s extensions to add functionality such as serialization, printing logs, or visualizing training statistics.
By understanding and applying these key points, you can effectively implement deep learning solutions using Chainer, overcoming challenges in research and development with ease.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)