- お役立ち記事
- How to use modules and visualize data
How to use modules and visualize data
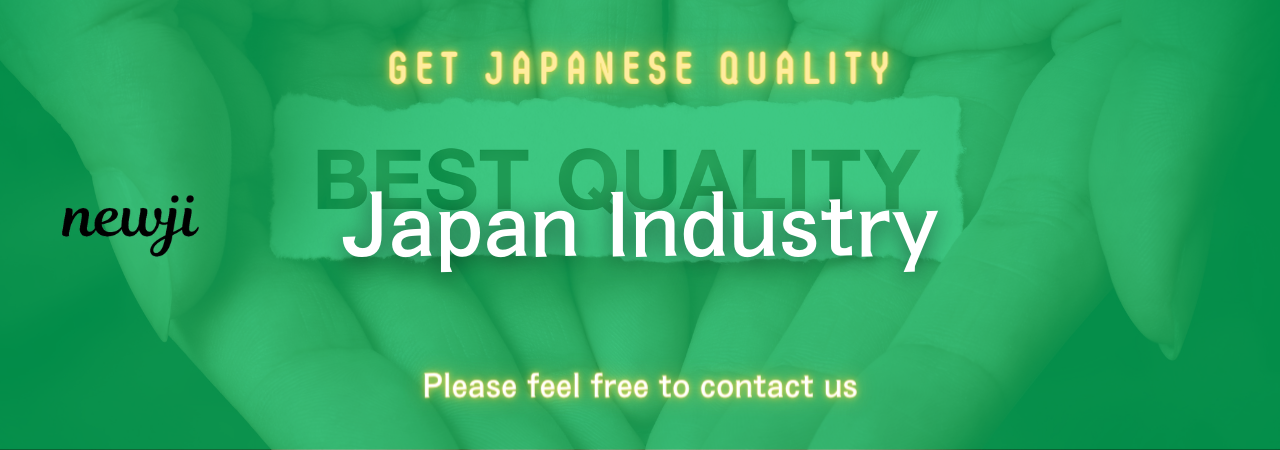
目次
Understanding Modules in Python
Python has gained immense popularity due to its simplicity and versatility.
One of the reasons for its widespread use is due to its modular nature, allowing developers to break up complex applications into smaller, manageable pieces called modules.
When you use modules, you organize your code better, enhance reusability, and make collaboration with others more straightforward.
Modules are essentially Python files (with a .py extension) that contain functions, variables, and classes.
By organizing your code into separate files, you can import and reuse these components across different projects or within the same project multiple times.
Creating and Using Modules
Creating a Python module is as simple as writing a Python script.
For example, if we have a file named `mymath.py` that contains functions like `add` and `subtract`, these can be used in other scripts.
Here’s how you might structure `mymath.py`:
“`python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
“`
To use this module in another script, you simply import it:
“`python
import mymath
result = mymath.add(5, 3)
print(“The sum is:”, result)
“`
You can also use specific functions from the module:
“`python
from mymath import add
result = add(5, 3)
print(“The sum is:”, result)
“`
Modules can be imported entirely, or you can import just the parts you need, making them highly flexible to work with.
Benefits of Using Modules
One primary advantage of using modules is code reusability.
By placing commonly used functions into a module, you can avoid rewriting code and reduce error rates.
Modules also help in maintaining code organization.
Instead of having one massive file, you can segment your code into logical groups, making it easier to navigate and manage.
Additionally, modules promote collaboration.
Different team members can work on separate modules without interfering with each other’s work, streamlining the development process.
Visualizing Data with Python
Data visualization is a crucial aspect of data analysis.
It enables you to see patterns, trends, and outliers, which might not be immediately obvious in raw data.
Python proves to be a powerful tool for data visualization thanks to its supportive community and a vast range of visualization libraries.
Popular Python Visualization Libraries
Several libraries in Python can help you create visual representations of your data quickly and efficiently.
Some of the most popular include:
1. **Matplotlib**: This is a plotting library that provides an object-oriented API for embedding plots into applications.
It’s ideal for creating static, interactive, and animated visualizations in Python.
2. **Seaborn**: Built on top of Matplotlib, Seaborn offers a more visually appealing and more straightforward interface for complex statistical plots.
It simplifies the creation of common plot types, and it’s excellent for creating aesthetically pleasing visualizations.
3. **Plotly**: Known for creating interactive plots, Plotly is fantastic for when you need more interactive visualizations.
It supports numerous chart types and can handle both 2D and 3D data visualizations.
4. **Pandas Visualization**: Pandas itself provides built-in plotting capabilities, using Matplotlib at its core.
For quick and simple visualizations, Pandas can be very handy.
Creating a Simple Visualization
Let’s walk through how to create a simple plot using Matplotlib.
Suppose you have data on sales figures for different months and want to visualize this information.
First, install Matplotlib if it’s not already available:
“`bash
pip install matplotlib
“`
Next, and create a basic line plot:
“`python
import matplotlib.pyplot as plt
months = [‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’, ‘May’]
sales = [15, 25, 8, 12, 30]
plt.plot(months, sales)
plt.title(‘Monthly Sales Data’)
plt.xlabel(‘Month’)
plt.ylabel(‘Sales’)
plt.show()
“`
This code will display a line graph showing sales trends over five months, allowing you to identify which months had the highest or lowest sales effortlessly.
Using Seaborn for Advanced Visualizations
While Matplotlib is powerful, Seaborn can help you create more complex visualizations with less code.
Consider using Seaborn when you need to work with datasets that require detailed statistical visualizations.
Here’s how you can create a bar plot using Seaborn:
First, install Seaborn if necessary:
“`bash
pip install seaborn
“`
Use the following example to visualize the same sales data:
“`python
import seaborn as sns
import matplotlib.pyplot as plt
months = [‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’, ‘May’]
sales = [15, 25, 8, 12, 30]
sns.barplot(x=months, y=sales)
plt.title(‘Monthly Sales Data’)
plt.xlabel(‘Month’)
plt.ylabel(‘Sales’)
plt.show()
“`
This bar plot provides a clearer view of the differences between sales figures for each month.
Conclusion
Using Python modules to organize your code and leveraging its extensive visualization libraries to present data effectively unlocks immense potential in data analysis and application development.
By breaking down your code into easily manageable modules, you improve readability and reusability.
Meanwhile, data visualization tools like Matplotlib and Seaborn bring your data to life, making patterns and insights more accessible.
Whether you’re a beginner or an experienced programmer, these techniques can significantly enhance your Python programming projects.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)