- お役立ち記事
- How to use OpenCV with Python
月間73,982名の
製造業ご担当者様が閲覧しています*
*2025年1月31日現在のGoogle Analyticsのデータより
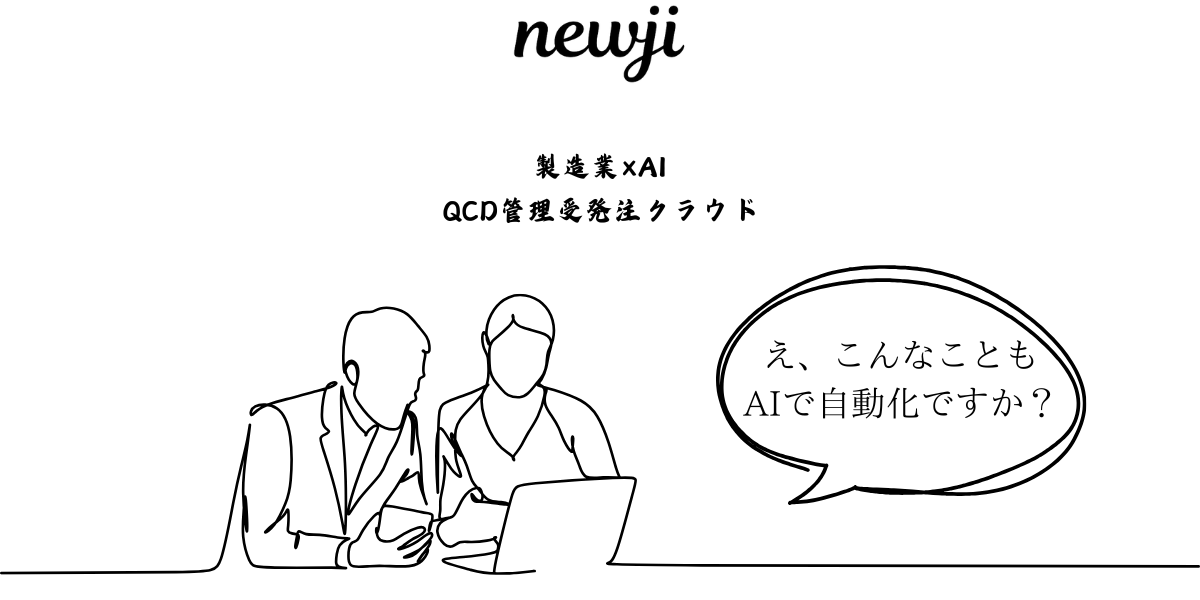
How to use OpenCV with Python
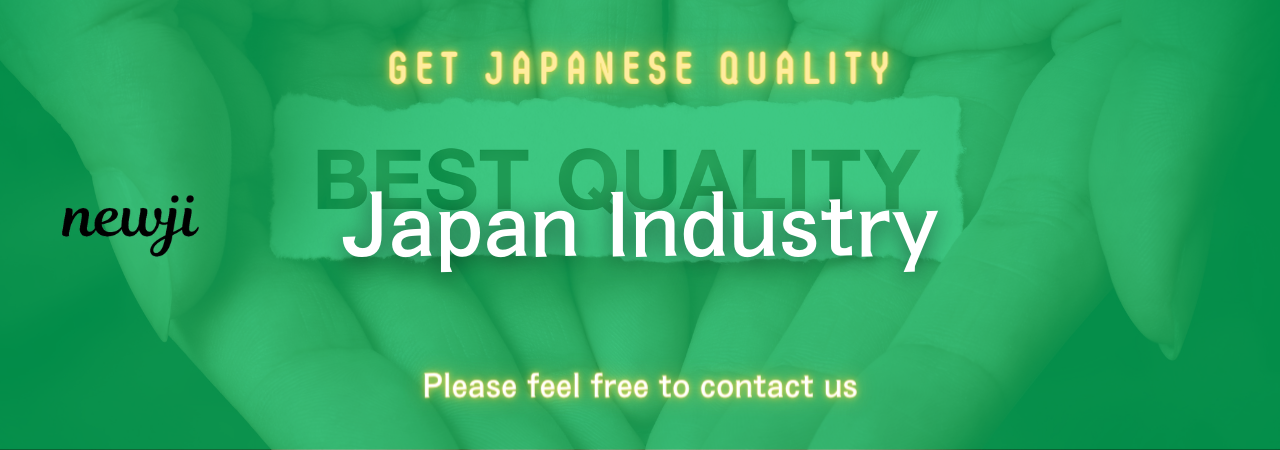
目次
Introduction to OpenCV and Python
OpenCV, or Open Source Computer Vision Library, is an open-source computer vision and machine learning software library.
It is designed to process images and video to identify objects, faces, or even the handwriting of a human.
With libraries like OpenCV, programmers can create real-time applications for various complex visual tasks.
Python, being a user-friendly language with an easy-to-read syntax, has become one of the favorite programming languages to work with OpenCV.
Python’s extensive collection of libraries and its flexibility enhance OpenCV’s capabilities, enabling developers to create robust and efficient applications.
Setting Up OpenCV in Python
Step 1: Install Python
Before getting started with OpenCV, you need to ensure that Python is installed on your system.
You can download and install it from Python’s official website.
It’s advisable to download the latest version for optimal functionality and features.
Step 2: Install OpenCV Library
Once Python is installed, the next task is to install the OpenCV library.
This can be done easily with the package manager `pip`.
Open your command prompt or terminal and type the following command:
“`
pip install opencv-python
“`
If you need the additional functionality offered by OpenCV’s additional modules (like `cv2`), you’ll also need to install:
“`
pip install opencv-contrib-python
“`
Step 3: Verify the Installation
After the installation process, verify that OpenCV has been successfully installed by typing the subsequent command in the Python prompt:
“`python
import cv2
print(cv2.__version__)
“`
If no error is thrown and the version number is printed, your installation was successful.
Basic Operations with OpenCV
Reading and Displaying Images
One of the fundamental operations in OpenCV is reading an image and displaying it.
Here is a simple Python code snippet for this task:
“`python
import cv2
# Read image
image = cv2.imread(‘example.jpg’)
# Display image
cv2.imshow(‘Displayed Image’, image)
# Wait until a key is pressed
cv2.waitKey(0)
# Destroy all windows
cv2.destroyAllWindows()
“`
Converting Color Spaces
In computer vision, changing color spaces is quite common.
For instance, to convert an image from BGR (Blue, Green, Red) to Grayscale, you can use:
“`python
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
“`
Advanced Image Processing Techniques
Edge Detection
One of the exciting features of OpenCV is its ability to perform edge detection.
Here’s how you can achieve that using the Canny edge detection method:
“`python
edges = cv2.Canny(image, 100, 200)
cv2.imshow(‘Edges’, edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
This code will reveal the edges present in an image which can be particularly useful in object detection.
Image Thresholding
Image thresholding is a technique for segmenting an image.
In OpenCV, you can do this by:
“`python
_, thresholded_image = cv2.threshold(gray_image, 127, 255, cv2.THRESH_BINARY)
cv2.imshow(‘Thresholded Image’, thresholded_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
Here, the threshold value is set to 127.
Pixel values below this threshold are turned to black, while those above are turned to white.
Working with Videos
Capturing Video from a Camera
Apart from static image processing, OpenCV excels in video processing.
This includes capturing video from a connected camera:
“`python
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
cv2.imshow(‘Video Frame’, frame)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
cv2.destroyAllWindows()
“`
Pressing ‘q’ will quit the video capture loop and close the window.
Writing Video to a File
OpenCV can also write frames to a video file:
“`python
fourcc = cv2.VideoWriter_fourcc(*’XVID’)
out = cv2.VideoWriter(‘output.avi’, fourcc, 20.0, (640, 480))
while True:
ret, frame = cap.read()
if not ret:
break
out.write(frame)
cv2.imshow(‘Frame’, frame)
if cv2.waitKey(1) & 0xFF == ord(‘q’):
break
cap.release()
out.release()
cv2.destroyAllWindows()
“`
This snippet writes the video capture to ‘output.avi’ file in Xvid format.
Conclusion
OpenCV and Python together make a powerful duo for anyone interested in computer vision or image processing tasks.
By following the steps and code snippets above, even beginners can quickly start experimenting with basic and advanced image processing techniques.
As with any skill, practice and continual learning will unlock more complex and exciting projects.
So dive in, explore, and transform the way you interact with images and video using the power of OpenCV and Python.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)