- お役立ち記事
- Image processing/machine learning programming using Python and its practice
Image processing/machine learning programming using Python and its practice
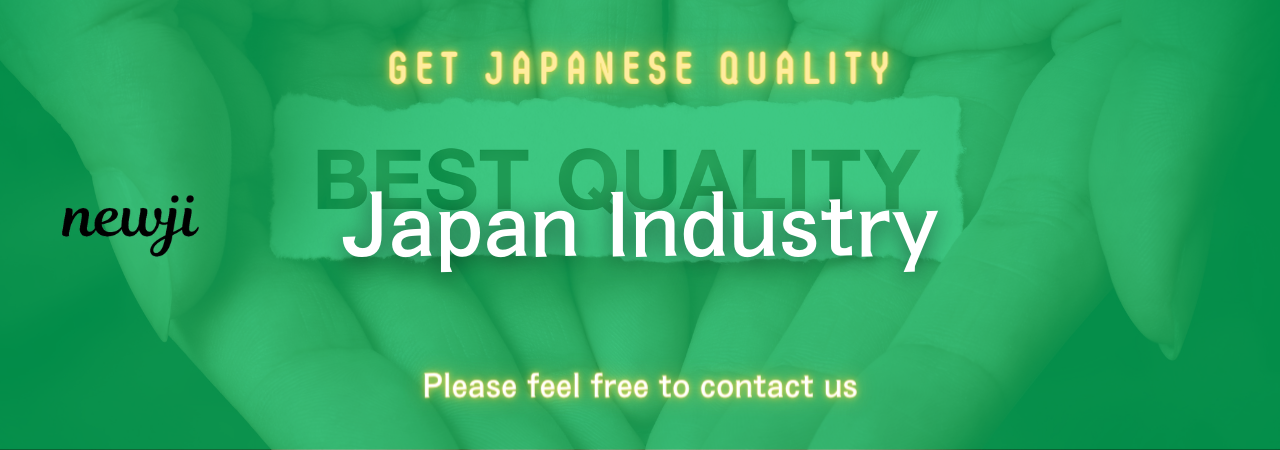
Introduction to Image Processing and Machine Learning with Python
Python has become one of the most popular programming languages for both image processing and machine learning due to its simplicity and the vast array of libraries available.
This guide will walk you through the basics of how to harness Python for these tasks and give you a practical overview of the tools you can use.
Why Use Python for Image Processing?
Python offers several powerful libraries specifically designed for image manipulation.
Two of the most commonly used libraries are OpenCV and PIL (Pillow).
They allow for a range of actions, from simple manipulations like cropping and resizing images to more advanced processes like filtering and transformations.
OpenCV, or Open Source Computer Vision Library, is particularly useful for real-time applications.
It supports a wide variety of image capture and processing techniques.
Pillow provides a more straightforward interface for simple image manipulation tasks.
Combining these libraries can yield effective tools for many image processing needs.
Basics of Image Processing
Image processing involves the handling and transformation of images into a preferred format or state.
This can include noise reduction, enhancing image quality, extracting features, and more.
To begin with Python, you’ll first need to install the necessary libraries.
You can use pip, Python’s package manager, as follows:
“`
pip install opencv-python
pip install pillow
“`
Once installed, you can start loading and manipulating images.
Consider a simple task like converting a colored image into a grayscale one:
“`python
from PIL import Image
image = Image.open(‘path_to_image.jpg’)
gray_image = image.convert(“L”)
gray_image.show()
“`
This code snippet opens an image, converts it to grayscale, and displays it.
Working with images in code opens up numerous possibilities for editing and enhancement.
Introduction to Machine Learning for Image Processing
Machine learning enables a system to learn from data patterns and make predictions or decisions without being explicitly programmed.
In image processing, machine learning can perform tasks like object detection, facial recognition, and image classification.
Popular Machine Learning Libraries in Python
Several libraries in Python provide advanced capabilities for building machine learning models:
– **Scikit-learn:** Known for its ease of use and straightforward API, scikit-learn offers many efficient tools for data mining and data analysis.
– **TensorFlow and Keras:** These libraries are essential for deep learning implementations, allowing for neural network construction and training.
– **PyTorch:** Known for its dynamic computational graph, PyTorch is a favorite among researchers for its flexibility and speed.
Setting Up a Basic Machine Learning Model
To illustrate machine learning for image processing, let’s construct a basic image classification task using a neural network.
First, ensure you have installed TensorFlow and Keras:
“`
pip install tensorflow
“`
Next, consider this simplified example using the famous MNIST dataset, which features handwritten digits:
“`python
import tensorflow as tf
from tensorflow.keras import datasets, layers, models
# Load and prepare the dataset
(train_images, train_labels), (test_images, test_labels) = datasets.mnist.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
# Build the model
model = models.Sequential([
layers.Flatten(input_shape=(28, 28)),
layers.Dense(128, activation=’relu’),
layers.Dense(10, activation=’softmax’)
])
# Compile the model
model.compile(optimizer=’adam’,
loss=’sparse_categorical_crossentropy’,
metrics=[‘accuracy’])
# Train the model
model.fit(train_images, train_labels, epochs=5)
# Evaluate the model
test_loss, test_acc = model.evaluate(test_images, test_labels)
print(f’\nTest accuracy: {test_acc}’)
“`
In this code block, we construct a simple neural network using Keras to classify images of digits.
The model is trained over five epochs and then evaluated for accuracy.
Practical Applications
Image processing and machine learning can solve numerous real-world problems.
– **Medical Imaging:** AI models can assist in detecting diseases in medical images, such as X-rays and MRIs, by highlighting abnormalities that medical professionals should examine further.
– **Self-driving cars:** Algorithms can recognize objects like pedestrians and traffic signals, enhancing road safety.
– **Security systems:** Facial recognition systems can identify individuals in access-controlled environments.
Conclusion
Python’s versatility makes it ideal for both image processing and machine learning endeavors.
Whether you’re a seasoned developer or a beginner, the availability of powerful libraries allows you to dive into complex projects with relative ease.
Experimenting with these tools can yield creative and impactful results that can translate into real-world applications.
So, get started with Python today, and delve into the fascinating intersection of image processing and machine learning.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)