- お役立ち記事
- Implementation of cryptographic technology using Python
月間76,176名の
製造業ご担当者様が閲覧しています*
*2025年3月31日現在のGoogle Analyticsのデータより
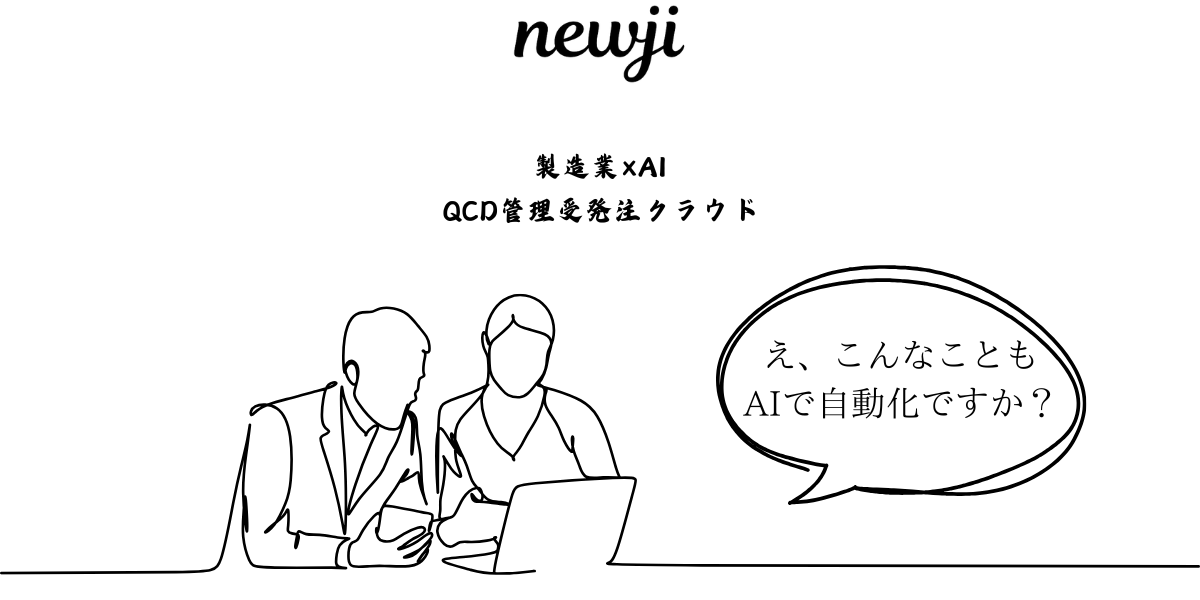
Implementation of cryptographic technology using Python
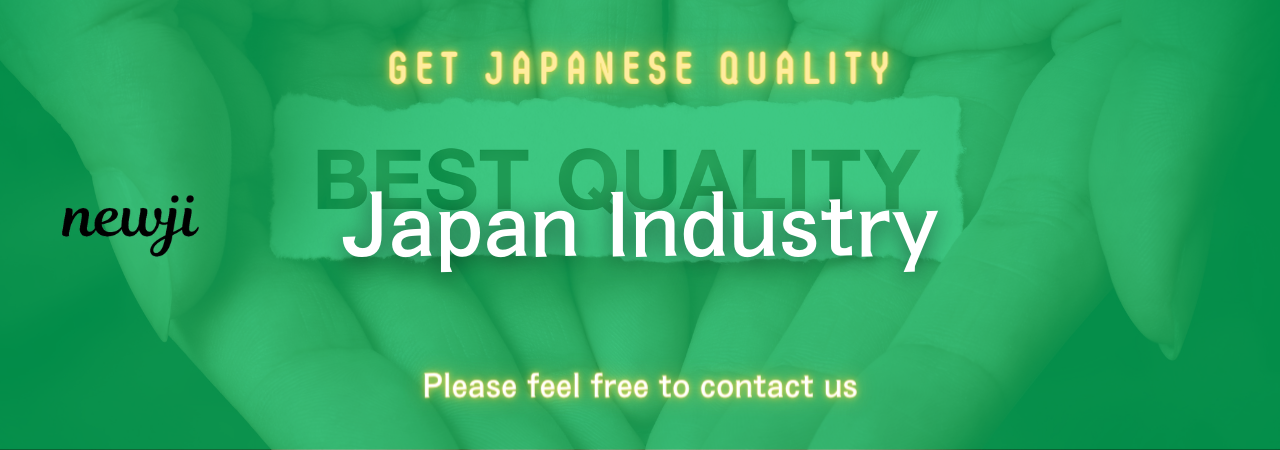
目次
Introduction to Cryptography and Python
Cryptography is the art of securing information by transforming it into a secure format.
It involves various methods of converting plain text into unintelligible data, ensuring that only authorized parties can understand it.
This process is crucial in safeguarding sensitive information from unauthorized access.
With the advancement of technology, cryptographic techniques have become more sophisticated, utilizing complex algorithms that are difficult to decipher without the proper decryption keys.
Python, a versatile and powerful programming language, offers numerous libraries and tools for implementing cryptographic protocols.
Its simplicity and readability make it an ideal choice for developers looking to implement cryptographic functions in their applications.
In this article, we will explore how Python can be used to implement cryptographic techniques, ensuring the safety and security of your data.
Understanding Basic Cryptographic Concepts
Before diving into Python’s cryptographic capabilities, it is essential to understand some basic concepts of cryptography.
The primary goal of cryptography is to protect data from unauthorized access.
It achieves this through two main processes: encryption and decryption.
Encryption
Encryption is the process of converting plain text into cipher text using an encryption algorithm and a key.
The output, cipher text, is unreadable under normal circumstances.
Only someone with the appropriate decryption key can revert it back to its original form.
Decryption
Decryption is the reverse process of encryption.
It involves converting the cipher text back to the original plain text using a decryption key.
Without the correct decryption key, it is nearly impossible to decode the encrypted message.
Types of Cryptography
There are several types of cryptography, each serving different purposes and offering different levels of security.
Symmetric Key Cryptography
In symmetric key cryptography, the same key is used for both encryption and decryption.
This method is efficient for large volumes of data but requires secure distribution of the key among all parties involved.
Asymmetric Key Cryptography
Asymmetric key cryptography, also known as public-key cryptography, uses a pair of keys: a public key and a private key.
The public key is used for encryption, while the private key is used for decryption.
This approach eliminates the need for secure key distribution but can be slower than symmetric cryptography.
Hash Functions
Hash functions transform input data into a fixed-size string of characters, which is usually a hash value.
Hashes are predominantly used for data integrity, ensuring that the data has not been altered during transmission.
Python Libraries for Cryptography
Python provides several libraries that facilitate the implementation of cryptographic techniques.
These libraries simplify the process of encrypting and decrypting data, generating keys, and more.
Cryptography Library
One of the most popular libraries is the `cryptography` library.
It offers both high-level recipes and low-level interfaces for working with cryptographic operations.
Installation is straightforward using pip:
“`bash
pip install cryptography
“`
PyCryptoDome
PyCryptoDome is another library that provides various cryptographic services.
It is a `Cryptographic` library providing a versatile array of cryptographic implementations including both symmetric and asymmetric algorithms:
“`bash
pip install pycryptodome
“`
Fernet Encryption
Fernet is a type of symmetric encryption available in the cryptography library.
It ensures that the message encrypted cannot be manipulated or read without the key.
Implementing Cryptography in Python
Let’s explore how to implement different cryptographic techniques using Python’s libraries.
Encrypting and Decrypting with Fernet
Fernet is an implementation of symmetric key cryptography designed to create strongly encrypted data.
“`python
from cryptography.fernet import Fernet
# Generate a key for encryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
message = b”This is a secret message”
cipher_text = cipher_suite.encrypt(message)
print(“Cipher Text: “, cipher_text)
# Decrypt the message
plain_text = cipher_suite.decrypt(cipher_text)
print(“Plain Text: “, plain_text.decode())
“`
Using RSA for Asymmetric Encryption
RSA is a popular algorithm for asymmetric encryption and can be implemented using PyCryptoDome.
“`python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# Generating RSA keys
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
# Encrypting Data
cipher = PKCS1_OAEP.new(RSA.import_key(public_key))
encrypted_message = cipher.encrypt(b’This is a secret message’)
# Decrypting Data
cipher = PKCS1_OAEP.new(RSA.import_key(private_key))
decrypted_message = cipher.decrypt(encrypted_message)
print(“Decrypted Message: “, decrypted_message.decode())
“`
Creating Hashes
Hashing is used to ensure data integrity and can be easily implemented using Python’s hashlib module.
“`python
import hashlib
message = b’This is a secret message’
hash_object = hashlib.sha256(message)
print(“Hashed Message: “, hash_object.hexdigest())
“`
Best Practices in Cryptography
Implementing cryptographic techniques requires careful consideration to ensure data security.
Here are few best practices:
Use Strong Algorithms
Always choose well-vetted and strong cryptographic algorithms.
Avoid outdated algorithms that may have known vulnerabilities.
Keep Keys Secure
The strength of cryptography relies heavily on the security of the keys.
Employ secure management practices to protect keys from unauthorized access.
Stay Updated
Cryptography is a rapidly evolving field.
Make sure to keep your software updated to the latest versions which include security patches and improvements.
Conclusion
Python offers a robust set of tools for implementing cryptographic technologies efficiently.
Understanding the basics of cryptography allows developers to choose the appropriate techniques for securing their applications.
By utilizing libraries like `cryptography` and `PyCryptoDome`, and adhering to best practices, you can ensure your data’s confidentiality, integrity, and security.
Start exploring the world of cryptography in Python and protect your data from the ever-evolving threats.
資料ダウンロード
QCD管理受発注クラウド「newji」は、受発注部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の受発注管理システムとなります。
ユーザー登録
受発注業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた受発注情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
製造業ニュース解説
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(β版非公開)