- お役立ち記事
- Implementation of cryptographic technology using Python
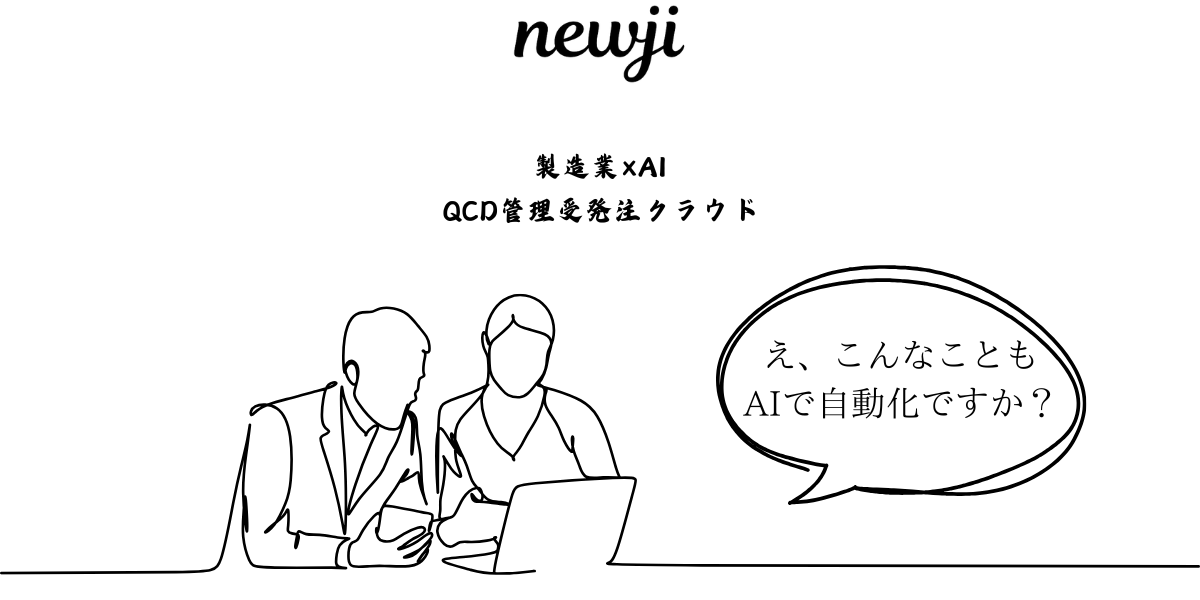
Implementation of cryptographic technology using Python
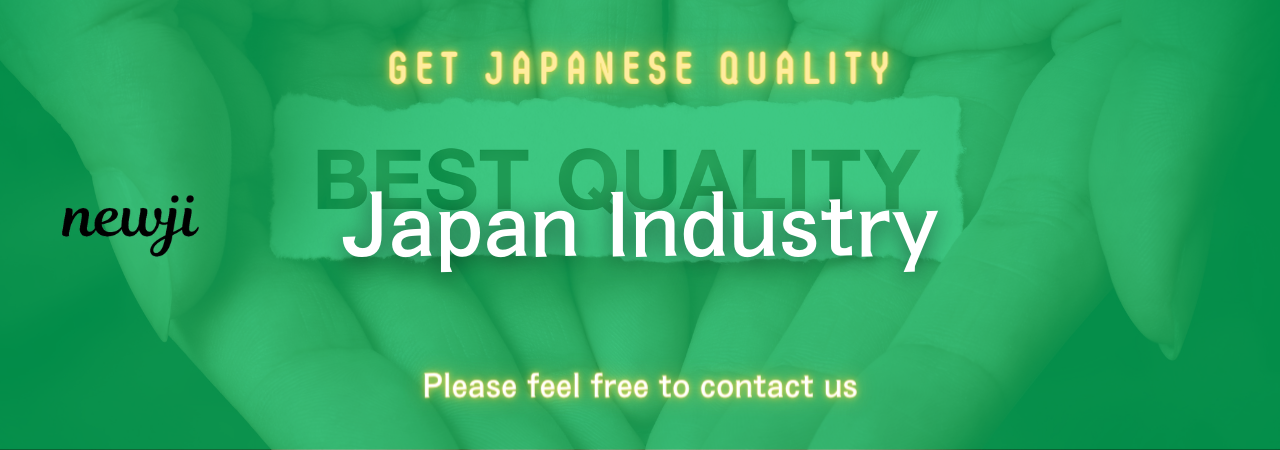
目次
Understanding Cryptographic Technology
Cryptographic technology has become a vital part of information security in the digital age.
It ensures that data is protected from unauthorized access and potential tampering.
By transforming readable data, or plaintext, into an unreadable format, or ciphertext, cryptographic technology safeguards sensitive information.
Importance of Cryptography
The importance of cryptography cannot be overstated.
It plays a crucial role in securing communication, authenticating identities, and maintaining data integrity.
In an era where cyber threats are rampant, cryptography provides a safeguard for transmitting data securely over networks.
Without cryptography, sensitive information like credit card numbers, personal messages, and business communications could be intercepted and used maliciously.
Python as a Tool for Cryptography
Python is a widely used programming language that offers numerous libraries and tools for implementing cryptographic technology.
Its straightforward syntax and vast community support make it an excellent choice for developers looking to work with cryptography.
Advantages of Using Python for Cryptography
There are several reasons why Python is favored for cryptographic implementations:
– **Ease of Use**: Python’s syntax is easy to read and write, allowing developers to quickly implement cryptographic functions.
– **Extensive Libraries**: Python offers libraries like `Cryptography`, `PyCrypto`, and `Hashlib`, which provide robust cryptographic functionalities.
– **Community Support**: The Python community is active and vast, providing plenty of resources, tutorials, and forums to help developers.
Implementing Basic Cryptographic Operations in Python
Python enables developers to perform various cryptographic operations, such as hashing, encryption, and decryption.
Hashing with Python
Hashing converts data into a fixed-size string of characters, which typically represents the data’s hash code.
It plays an essential role in ensuring data integrity.
Here’s an example of how you can use Python to hash a piece of text:
“`python
import hashlib
def hash_text(text):
return hashlib.sha256(text.encode()).hexdigest()
text = “Hello, World!”
hashed_text = hash_text(text)
print(f”Original Text: {text}”)
print(f”Hashed Text: {hashed_text}”)
“`
In this code, `hashlib` is a built-in library for hashing messages using algorithms like SHA-256.
The `hash_text` function converts a string into its hash equivalent using SHA-256.
Encrypting and Decrypting Messages
Encryption involves converting plaintext into ciphertext using an algorithm and a key, while decryption is the reverse process.
Here is an example using the `Fernet` symmetric encryption from the `cryptography` library:
“`python
from cryptography.fernet import Fernet
def encrypt_message(message, key):
fernet = Fernet(key)
encrypted_message = fernet.encrypt(message.encode())
return encrypted_message
def decrypt_message(encrypted_message, key):
fernet = Fernet(key)
decrypted_message = fernet.decrypt(encrypted_message).decode()
return decrypted_message
# Generate a key
key = Fernet.generate_key()
message = “This is a secret message.”
encrypted = encrypt_message(message, key)
decrypted = decrypt_message(encrypted, key)
print(f”Original Message: {message}”)
print(f”Encrypted Message: {encrypted}”)
print(f”Decrypted Message: {decrypted}”)
“`
This example demonstrates how to encrypt and decrypt messages using a symmetric key.
The `cryptography` library handles the intricate processes of converting text back and forth between plaintext and ciphertext.
Choosing the Right Cryptographic Method
Selecting the appropriate cryptographic technique depends on the security needs of your application.
The key factors include:
Symmetric vs. Asymmetric Encryption
Symmetric encryption uses the same key for both encryption and decryption, which makes it faster and suitable for encrypting large amounts of data.
However, the key distribution can pose security risks.
Asymmetric encryption uses a pair of keys — a public key for encryption and a private key for decryption.
It is more secure for key distribution but is slower than symmetric encryption.
Hashing Algorithms
Choosing the right hashing algorithm is crucial for ensuring data integrity and security.
Algorithms like SHA-256 and SHA-3 are widely used due to their strong security properties.
It’s important to ensure that chosen algorithms are resistant to collision attacks, where two different inputs produce the same hash.
Enhanced Security Measures
Implementing cryptographic technology requires more than just encryption and hashing.
Additional measures should be considered to ensure complete security.
Secure Key Management
Properly managing cryptographic keys is crucial to maintaining security.
Keys should be stored securely and rotated regularly to prevent unauthorized access.
Regular Security Audits
Regularly auditing your cryptographic implementations and exploring newer, more secure algorithms can help maintain the integrity of your data protection mechanisms.
As technology advances, ensuring that your cryptographic methods keep pace with the latest security standards is crucial.
Conclusion
Cryptographic technology is essential for securing digital communications and sensitive data.
Python, with its comprehensive libraries and ease of use, provides a robust platform for implementing cryptographic solutions.
By understanding the basics of hashing, encryption, and decryption, and choosing suitable methods based on security needs, developers can build secure applications that protect data integrity and confidentiality.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)