- お役立ち記事
- Machine learning/anomaly detection programming using Python and its practice
Machine learning/anomaly detection programming using Python and its practice
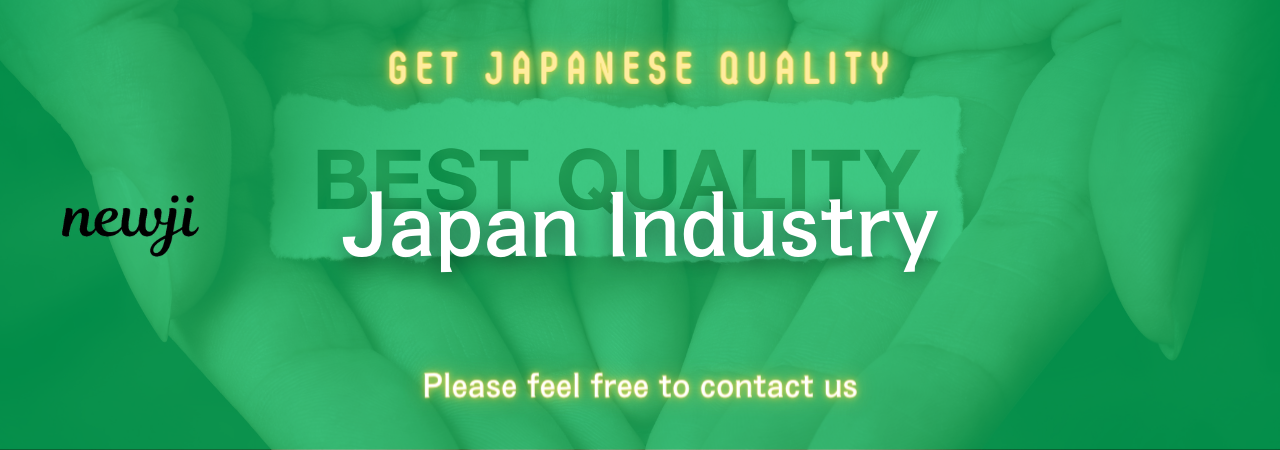
目次
Introduction to Machine Learning and Anomaly Detection
In today’s digital age, data is generated at a staggering rate.
From social media posts to online shopping transactions, data is everywhere.
With the volume of data being so vast, it becomes imperative to have efficient systems that can process and analyze this data.
One of the most effective tools in this realm is machine learning.
Machine learning allows computers to learn from data patterns and make decisions with minimal human intervention.
One of the exciting applications of machine learning is anomaly detection.
Anomaly detection is the process of identifying data points in a dataset that deviate from the norm.
These anomalies can signify potential issues, fraud, or rare events.
Being able to automatically detect these anomalies has become crucial for businesses, cybersecurity, and even medical diagnoses.
In this article, we’ll dive into how you can leverage the power of Python for machine learning and anomaly detection.
We’ll also explore some practical applications and how to get started with a simple project.
Understanding Anomaly Detection
Anomalies, also known as outliers, are data points that differ significantly from other observations.
Detecting these outliers is essential because they might represent critical events or errors.
Anomalies can be the result of fraudulent transactions, network intrusions, or even equipment malfunctions.
In machine learning, we can categorize anomaly detection methods into three primary types:
1. **Supervised Anomaly Detection**: In this method, the training dataset is labeled as normal or anomalous. The model is trained to classify new data into these categories.
2. **Unsupervised Anomaly Detection**: This method doesn’t rely on labeled data. Instead, it identifies anomalies based on patterns and distributions in the dataset.
3. **Semi-supervised Anomaly Detection**: This method is used when the training data only contains normal observations. The model learns what constitutes “normal behavior” and flags data that deviates from this learned norm.
Now that we understand the basics let’s see how Python can be used to implement these methods.
Getting Started with Python for Machine Learning
Python, a versatile programming language, is a popular choice for data science and machine learning tasks.
It offers a rich set of libraries and tools to simplify and enhance the process.
To start with machine learning in Python, you’ll need to have Python installed on your computer.
Along with that, some essential libraries include:
– **NumPy**: For numerical computations and handling arrays.
– **Pandas**: For data manipulation and analysis.
– **scikit-learn**: A robust library for machine learning tasks.
– **Matplotlib and Seaborn**: For data visualization.
Once you have these tools ready, you can embark on building your anomaly detection system.
Implementing Anomaly Detection Using Python
To implement a basic anomaly detection system, we’ll use the scikit-learn library.
Let’s walk through a simple example:
1. **Prepare Your Data**: First, gather and prepare the dataset you wish to analyze.
For this example, we can use a simple dataset that contains numbers with anomalies inserted.
2. **Load and Explore the Data**: Use Pandas to load and inspect the data.
“`python
import pandas as pd
# Load data
data = pd.read_csv(‘your_dataset.csv’)
# Display first few rows
print(data.head())
“`
3. **Feature Scaling**: Normalize or standardize your data.
This step is essential to ensure that all features contribute equally to the anomaly detection process.
“`python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data_scaled = scaler.fit_transform(data)
“`
4. **Choose an Anomaly Detection Model**: For this example, we’ll use the Isolation Forest algorithm, a widely-used model for anomalous pattern detection.
“`python
from sklearn.ensemble import IsolationForest
model = IsolationForest(contamination=0.1)
model.fit(data_scaled)
# Predict anomalies
anomalies = model.predict(data_scaled)
“`
5. **Visualize the Results**: Use Matplotlib or Seaborn to visualize the detected anomalies.
“`python
import matplotlib.pyplot as plt
import seaborn as sns
sns.scatterplot(x=data[‘Feature1’], y=data[‘Feature2’], hue=anomalies)
plt.title(‘Anomaly Detection’)
plt.show()
“`
Here, a contamination rate of 0.1 implies that we expect 10% of our dataset to contain anomalies.
You can adjust this parameter based on your data’s characteristics and needs.
Real-World Applications of Anomaly Detection
Anomaly detection has diverse applications across various domains.
Let’s explore some practical scenarios where this technology is making a significant impact:
Fraud Detection in Finance
Financial institutions leverage anomaly detection to identify fraudulent activities.
By analyzing transaction patterns, systems can flag suspicious activities, such as unusual spending in atypical locations or abrupt account changes.
Network Security
In the realm of cybersecurity, identifying unauthorized network access or unusual traffic patterns is crucial.
Anomaly detection tools can monitor network behavior in real-time, promptly alerting security teams to potential threats.
Healthcare and Medical Diagnostics
In healthcare, anomaly detection aids in recognizing unusual patterns in patient data, helping in early disease diagnoses or monitoring patient vitals for irregularities.
Conclusion
Machine learning and anomaly detection are transforming the way we handle and process data.
Python, with its comprehensive libraries, simplifies the task of implementing complex algorithms for these purposes.
By following the steps outlined above, you can embark on your journey to harness the power of machine learning for anomaly detection.
As technology continues to evolve, the importance of quick and accurate data analysis becomes even more pronounced.
Whether you’re working in finance, cybersecurity, or any field that deals with significant amounts of data, mastering anomaly detection will be invaluable.
Dive into Python, explore its capabilities, and start building smarter systems today!
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)