- お役立ち記事
- Machine learning/anomaly detection programming using Python and its practice
Machine learning/anomaly detection programming using Python and its practice
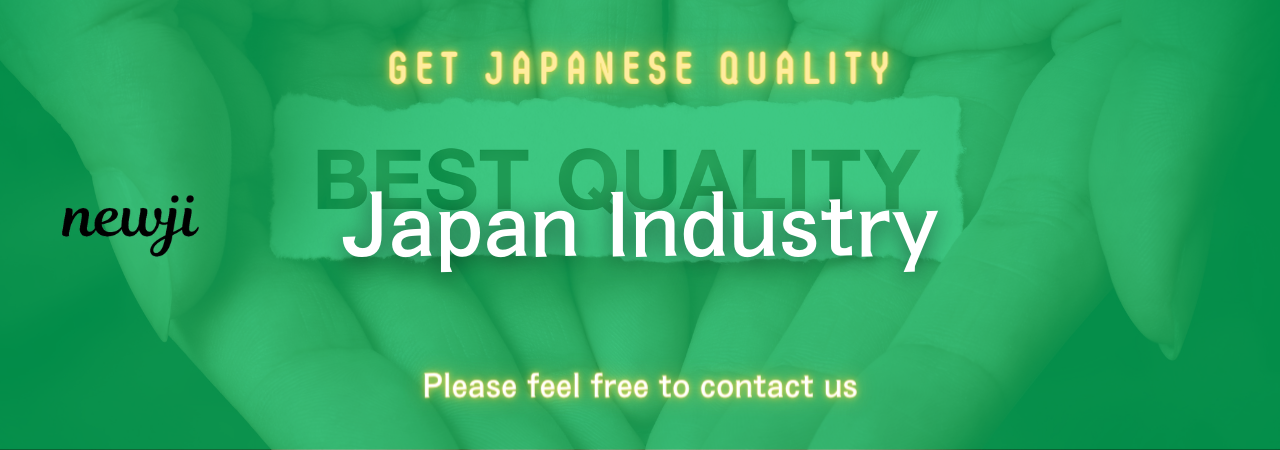
目次
Understanding Machine Learning and Anomaly Detection
Machine learning is a branch of artificial intelligence that enables computers to learn from data and improve their performance without being explicitly programmed.
It has become a vital part of many applications, ranging from simple tasks like email filtering to complex operations in finance, healthcare, and autonomous vehicles.
Anomaly detection, a key aspect of machine learning, is a process that identifies unusual patterns or outliers in the data.
Anomalies are instances that deviate significantly from the majority of data points and can indicate critical incidents, faults, or changes that require attention.
Why Use Python for Machine Learning?
Python is an excellent choice for machine learning and anomaly detection due to its simplicity, readability, and vast library support.
It enables developers to write less code with fewer bugs and supports integration with other languages and platforms.
Python’s machine learning libraries, such as Scikit-Learn, TensorFlow, and PyTorch, offer robust tools for building and deploying machine learning models.
These libraries provide pre-built functions and algorithms that ease the development process, allowing you to focus on resolving the core problem efficiently.
Getting Started with Python Programming
To get started with Python for machine learning and anomaly detection, you need to have a basic understanding of programming concepts and familiarity with Python syntax.
Installing Python and setting up an integrated development environment (IDE) like Jupyter Notebook or PyCharm can greatly enhance your coding experience.
Once the environment is set, installing necessary libraries using Python’s package manager, pip, is straightforward.
For most machine learning tasks, libraries such as NumPy, Pandas, Matplotlib, and Scikit-Learn are essential.
These tools provide capabilities ranging from numerical analysis to data visualization and machine learning algorithms.
Preparing and Exploring Your Data
Before diving into anomaly detection, it’s crucial to prepare and understand your dataset.
Data preprocessing involves cleaning, transforming, and organizing the data to make it suitable for analysis.
Using Pandas, you can handle data in Python effectively, performing operations like filtering, grouping, and aggregating with ease.
Visualizing data with Matplotlib or Seaborn can help uncover trends, correlations, and potential anomalies that might exist in your dataset.
Building Anomaly Detection Models
Once your data is prepared, the next step is to choose an appropriate anomaly detection technique.
There are several methods to consider, each with its advantages depending on your specific requirements and the nature of your data.
Statistical Methods
Statistical methods assume that normal data follows a certain distribution and detects deviations from this pattern.
Commonly used statistical techniques include Z-score analysis and Gaussian distribution fitting.
Clustering-Based Methods
Clustering algorithms like K-means and DBSCAN can help group similar data points together.
Anomalies are identified as those points that do not fit well into any cluster.
Classification-Based Methods
If you have labeled data, classification algorithms like decision trees, support vector machines, or neural networks can be trained to detect anomalies as a classification problem.
Implementing Anomaly Detection in Python
Let’s consider a simple example of implementing anomaly detection using the Isolation Forest algorithm available in Scikit-Learn.
“`python
import numpy as np
import pandas as pd
from sklearn.ensemble import IsolationForest
import matplotlib.pyplot as plt
# Load the dataset
data = pd.read_csv(‘data.csv’)
# Preprocess the data
features = data[[‘feature1’, ‘feature2’]]
# Create an Isolation Forest model
model = IsolationForest(n_estimators=100, contamination=0.05, random_state=42)
# Fit the model to the data
model.fit(features)
# Predict anomalies
data[‘anomaly’] = model.predict(features)
# Visualize the anomalies
plt.scatter(data[‘feature1’], data[‘feature2’], c=data[‘anomaly’], cmap=’coolwarm’)
plt.title(‘Anomaly Detection with Isolation Forest’)
plt.xlabel(‘Feature 1’)
plt.ylabel(‘Feature 2’)
plt.show()
“`
This code demonstrates a basic implementation of anomaly detection.
Here, we load data, preprocess it by selecting relevant features, and use the Isolation Forest algorithm to identify anomalies.
Finally, we visualize the results using Matplotlib.
Evaluating Anomaly Detection Models
Evaluating the effectiveness of anomaly detection models can be challenging, especially when ground truth labels are unavailable.
Some common evaluation methods include:
Precision, Recall, and F1 Score
In case of available labeled data, you can use precision, recall, and F1 score metrics to evaluate the model’s performance.
Visualization
Visualizing predictions alongside the original data can offer insights into the model’s accuracy in detecting anomalies.
Domain Expert Analysis
In the absence of labeled data, collaborating with domain experts to validate detected anomalies can be valuable.
Practical Applications of Anomaly Detection
Anomaly detection is widely used in various industries for applications such as:
Fraud Detection
In financial services, anomaly detection helps identify fraudulent transactions or suspicious account activities.
Network Security
Detecting unusual patterns in network traffic can reveal potential cybersecurity threats and prevent data breaches.
Healthcare
In medical data, anomaly detection can assist in identifying outliers that may indicate health issues or misdiagnoses.
Manufacturing
In industrial settings, detecting equipment anomalies may signal the need for maintenance, reducing the risk of failure.
Conclusion
Python, with its rich ecosystem of libraries, offers an accessible and powerful platform for implementing machine learning and anomaly detection.
By understanding and selecting appropriate techniques, preparing and visualizing data, and evaluating models, you can build effective anomaly detection systems tailored to your needs.
As the field continues to evolve, staying informed about the latest advancements and best practices is crucial for leveraging machine learning and anomaly detection to solve real-world problems efficiently.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)