- お役立ち記事
- Machine learning basics and implementation programming practical course using Python
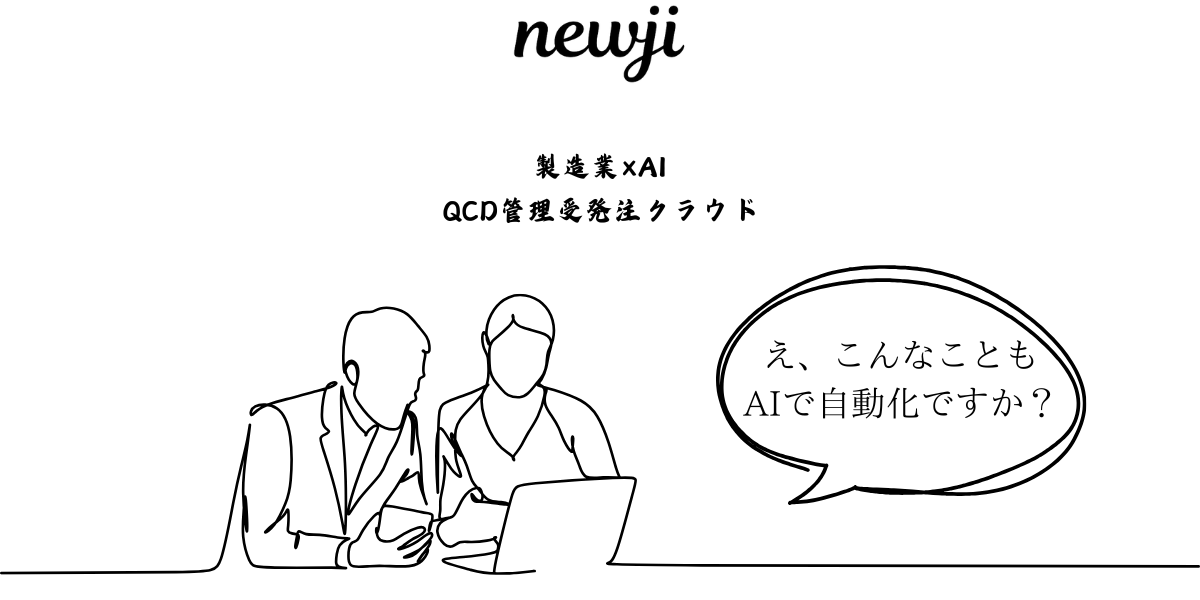
Machine learning basics and implementation programming practical course using Python
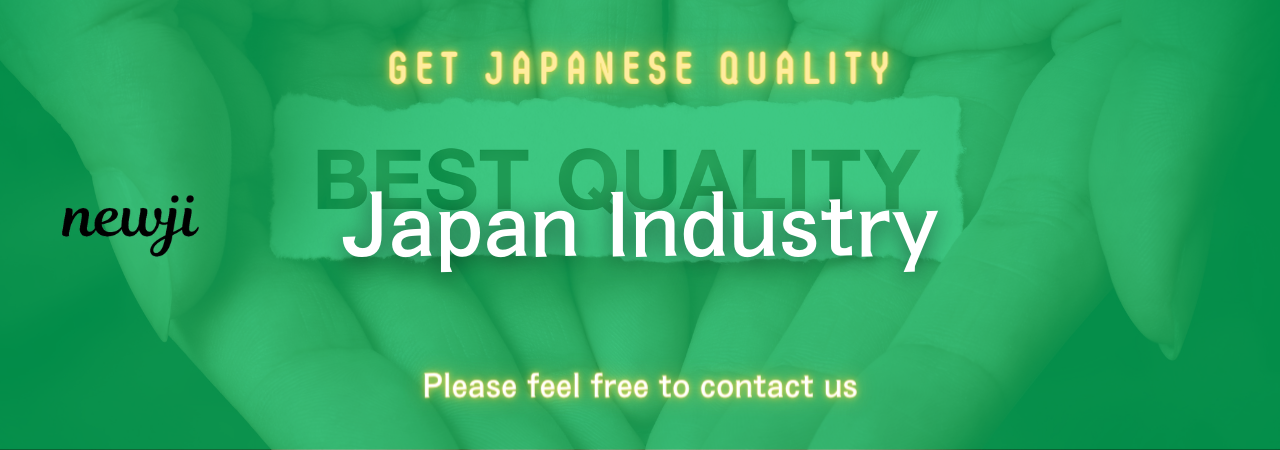
目次
Understanding Machine Learning
Machine learning is a branch of artificial intelligence that focuses on the use of data and algorithms to imitate the way humans learn.
It’s a practical implementation of AI, designed to enable systems to learn and improve from experience without being explicitly programmed.
Machine learning can be found in various applications that influence our daily lives.
From personalized recommendations on streaming services to email filtering and smart assistants, these technologies are powered by machine learning algorithms.
Types of Machine Learning
There are three main types of machine learning: supervised learning, unsupervised learning, and reinforcement learning.
Supervised Learning
Supervised learning involves training a model on a labeled dataset.
In this scenario, the model learns from input-output pairs, using this experience to predict outcomes for new data.
Common applications include image recognition, fraud detection, and forecasting.
Unsupervised Learning
Unsupervised learning does not use labeled data.
Instead, it identifies patterns or structures within the input data.
It’s often used for clustering, association, and dimensionality reduction.
Market basket analysis and customer segmentation are examples of unsupervised learning applications.
Reinforcement Learning
Reinforcement learning involves an agent that learns to make decisions by performing actions and receiving feedback.
This type of learning is suitable for robotics, gaming, and any scenario requiring strategic decision making.
Getting Started with Python for Machine Learning
Python is a popular language for implementing machine learning due to its simplicity and the availability of powerful libraries.
To start programming for machine learning, you’ll need to set up a suitable environment.
Installing Python
First, install Python on your computer if it’s not already available.
The latest version can be downloaded from the official Python website.
It’s recommended to use Python version 3.0 or above to access the latest features and libraries.
Setting Up a Virtual Environment
Virtual environments ensure that your projects are isolated from each other, keeping dependencies confined.
Use the following commands to create and activate a virtual environment:
“`bash
# To create a virtual environment
python -m venv myenv
# To activate the virtual environment on Windows
myenv\Scripts\activate
# On macOS and Linux
source myenv/bin/activate
“`
Installing Essential Libraries
Several libraries offer robust support for machine learning in Python.
Some of the essential libraries include:
– **NumPy**: Provides support for large multi-dimensional arrays and matrices.
– **Pandas**: Used for data manipulation and analysis.
– **Scikit-learn**: A central library for machine learning in Python, covering a wide array of algorithms.
– **Matplotlib**: Used for creating static, interactive, and animated visualizations in Python.
Install these libraries using pip:
“`bash
pip install numpy pandas scikit-learn matplotlib
“`
Implementing a Simple Machine Learning Model
Let’s walk through a simple example of implementing a machine learning model in Python using the Scikit-learn library.
Linear Regression Model
Linear regression is a basic predictive technique that explores the relationship between a dependent and an independent variable.
We will use Scikit-learn to create a straightforward linear regression model.
Data Preparation
Before building the model, prepare your data.
For this tutorial, consider a simple dataset with a single feature and a continuous target value.
“`python
import numpy as np
import pandas as pd
# Sample data
data = {‘feature’: [1, 2, 3, 4, 5], ‘target’: [5, 7, 9, 11, 13]}
df = pd.DataFrame(data)
# Separate the independent and dependent variables
X = df[[‘feature’]]
y = df[‘target’]
“`
Fitting the Model
Load the linear regression model and fit the data.
“`python
from sklearn.linear_model import LinearRegression
model = LinearRegression()
# Fit the model
model.fit(X, y)
“`
Making Predictions
Once the model is trained, you can make predictions using new data.
“`python
# New data for prediction
new_data = np.array([[6], [7]])
predictions = model.predict(new_data)
print(“Predictions:”, predictions)
“`
Evaluating the Model
Evaluate the performance of your model using standard metrics such as Mean Absolute Error, Mean Squared Error, or R-squared.
“`python
from sklearn.metrics import mean_squared_error, r2_score
# Predictions on the training data
y_pred = model.predict(X)
# Calculate evaluation metrics
mse = mean_squared_error(y, y_pred)
r2 = r2_score(y, y_pred)
print(“Mean Squared Error:”, mse)
print(“R-squared:”, r2)
“`
Conclusion
Machine learning is a continually evolving field with vast potential.
Python serves as a great starting point for beginners due to its ease of use and the plethora of tools available.
By mastering the basics of machine learning and implementing simple models, like the linear regression example above, you can start exploring more complex algorithms and their applications.
As you gain more experience, consider diving deeper into other libraries and frameworks like TensorFlow or PyTorch for more advanced machine learning challenges.
Ultimately, practical experience coupled with continuous learning will enhance your understanding and skills in this exciting domain.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)