- お役立ち記事
- Practical points for data analysis using Python and application to predictive model creation
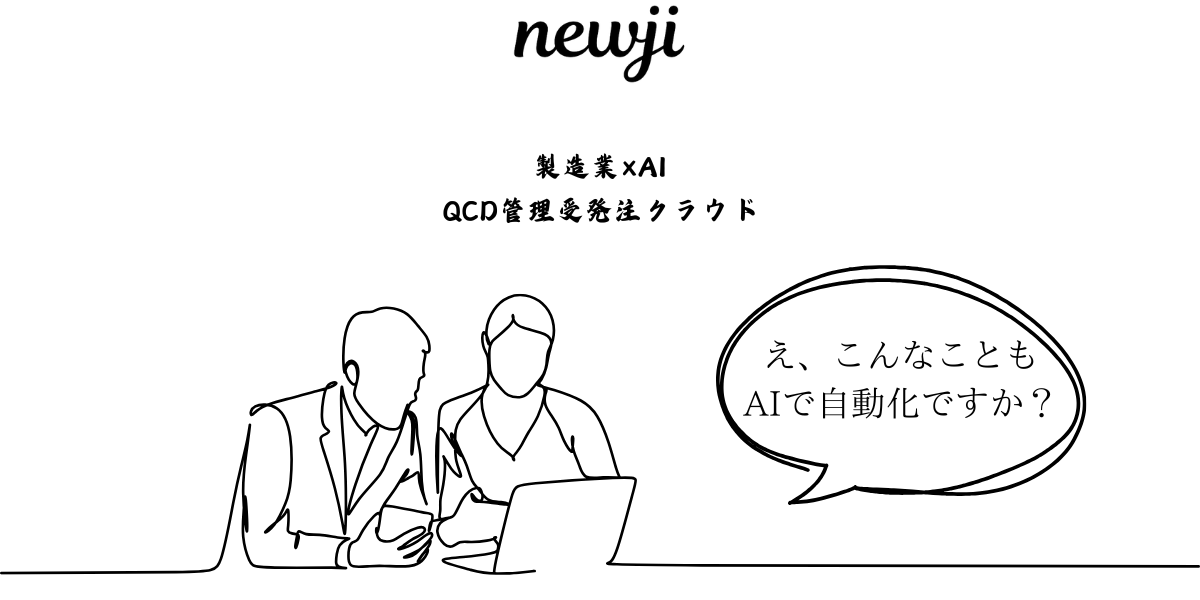
Practical points for data analysis using Python and application to predictive model creation
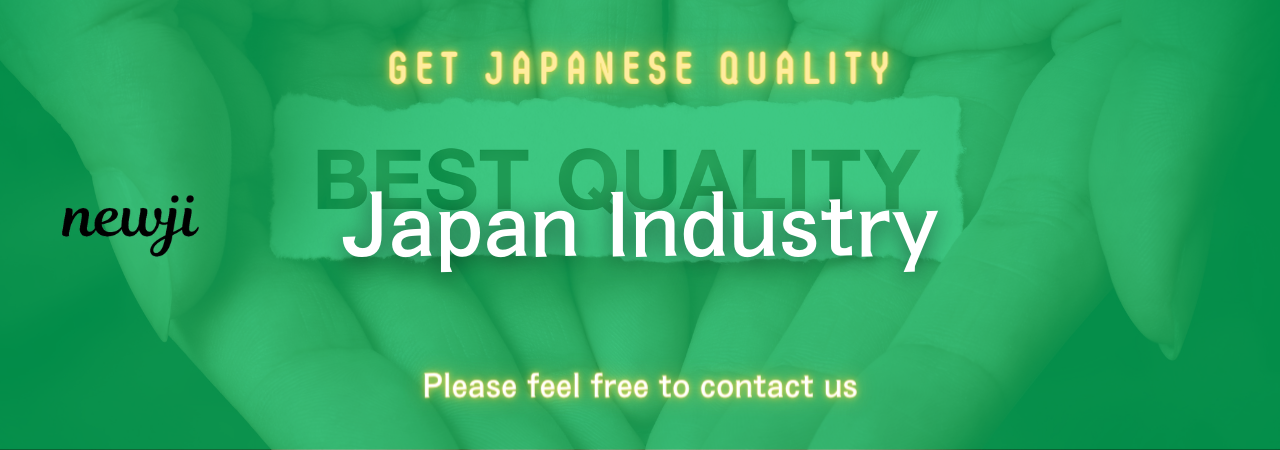
目次
Introduction to Data Analysis with Python
Data analysis is a crucial aspect of modern business and research, helping organizations and individuals make informed decisions based on empirical evidence.
Python has emerged as a powerful tool for data analysis due to its versatility and ease of use.
In this article, we’ll explore practical points for data analysis using Python and delve into its application in creating predictive models.
Python offers a rich ecosystem of libraries and tools that make data analysis both efficient and accessible.
By harnessing these tools, data analysts and scientists can uncover meaningful insights and build robust predictive models.
Getting Started with Python for Data Analysis
The first step in utilizing Python for data analysis is to set up the appropriate environment.
This typically involves installing Python and key libraries such as NumPy, pandas, Matplotlib, and SciPy.
These libraries form the backbone of data analysis in Python, each serving a distinct purpose.
Installing Essential Libraries
To get started, ensure Python is installed on your system.
You can download it from the official Python website.
Once installed, you can use a package manager like pip to install the necessary libraries:
“`
pip install numpy pandas matplotlib scipy
“`
This command will install the mentioned libraries, providing the fundamental tools required for your data analysis tasks.
Data Manipulation with pandas
Pandas is a powerful data manipulation library in Python.
It enables you to load, manipulate, and analyze data efficiently.
With pandas, you can handle various data structures and perform tasks such as filtering, grouping, and aggregating data.
One of the key data structures in pandas is the DataFrame, which allows you to store and manipulate tabular data.
DataFrames are akin to Excel spreadsheets or SQL tables, making them intuitive for those familiar with these tools.
Loading Data
You can load data from various file formats, including CSV, Excel, and SQL databases.
Here’s an example of how to read a CSV file into a DataFrame:
“`python
import pandas as pd
data = pd.read_csv(‘data.csv’)
print(data.head())
“`
This code snippet reads data from ‘data.csv’ and displays the first few rows, giving you a quick glimpse of your dataset.
Exploring Data
Once you’ve loaded your data, it’s essential to explore and understand its structure.
Pandas provides various methods to help you explore your data:
“`python
print(data.info())
print(data.describe())
“`
The `info()` method gives you a summary of your DataFrame, including data types and null values, while `describe()` provides statistical insights such as mean and standard deviation.
Data Visualization with Matplotlib
Visualizing data is crucial for gaining insights and communicating findings effectively.
Matplotlib is a popular library for creating static, interactive, and animated visualizations in Python.
It offers a wide range of plotting options to suit different needs.
Creating Basic Plots
You can create line plots, scatter plots, bar charts, and more using Matplotlib.
Here’s an example of a simple line plot:
“`python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.xlabel(‘X-axis Label’)
plt.ylabel(‘Y-axis Label’)
plt.title(‘Simple Line Plot’)
plt.show()
“`
This code generates a basic line plot, allowing you to visualize the relationship between the `x` and `y` variables.
Building Predictive Models
Predictive modeling is a powerful application of data analysis that uses statistical algorithms and machine learning techniques to predict future outcomes.
Python provides several libraries for building predictive models, including scikit-learn, TensorFlow, and Keras.
Understanding Model Building
Before building a predictive model, it’s essential to preprocess your data.
This involves tasks such as scaling, encoding categorical variables, and splitting data into training and test sets.
Once your data is ready, you can select an appropriate algorithm and fit your model to the data.
Common algorithms include linear regression, decision trees, and support vector machines.
Evaluating Model Performance
After building your model, evaluate its performance using metrics such as accuracy, precision, recall, and F1-score.
It is crucial to validate your model using a separate test dataset to ensure it generalizes well to unseen data.
“`python
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Splitting data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Training the model
model = LinearRegression()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
# Evaluating the model
mse = mean_squared_error(y_test, predictions)
print(f’Mean Squared Error: {mse}’)
“`
This code snippet demonstrates a typical workflow for building and evaluating a linear regression model using scikit-learn.
Conclusion
Data analysis using Python is a potent means to extract valuable insights and build predictive models that shape the future of data-driven decision-making.
By leveraging powerful libraries such as pandas for data manipulation and Matplotlib for visualization, and scikit-learn for model building, users can efficiently navigate through data complexities.
Whether you’re a beginner or an experienced data analyst, Python’s ecosystem provides the tools necessary to empower your data analysis journey.
With continuous learning and practice, you can enhance your skills and make impactful contributions across various fields.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)