- お役立ち記事
- Practical Python programming basics and practice
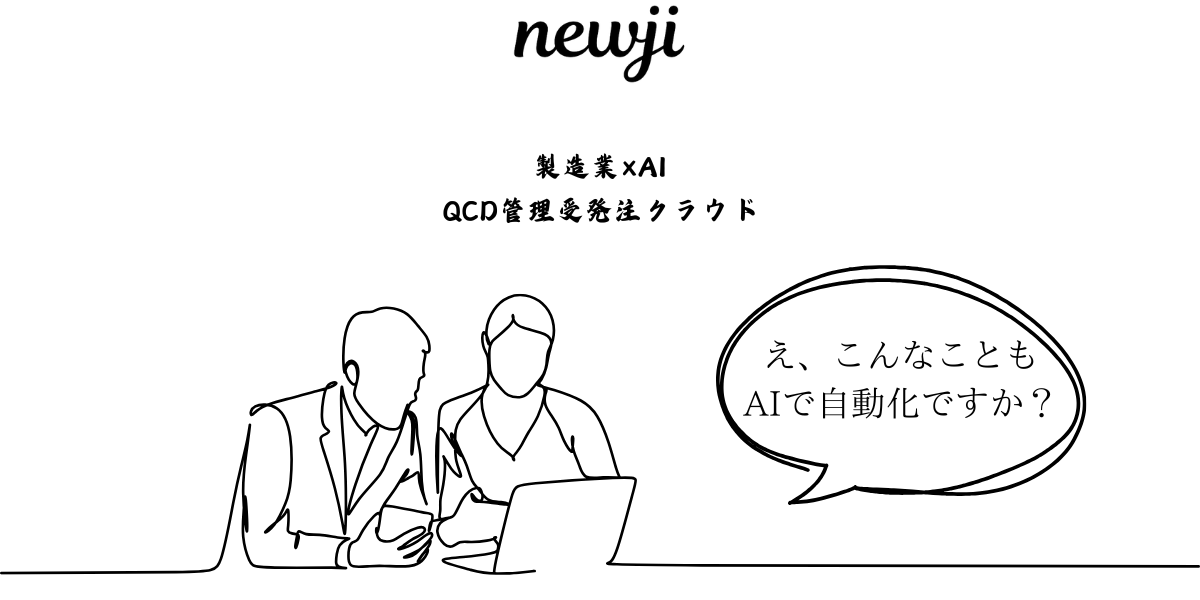
Practical Python programming basics and practice
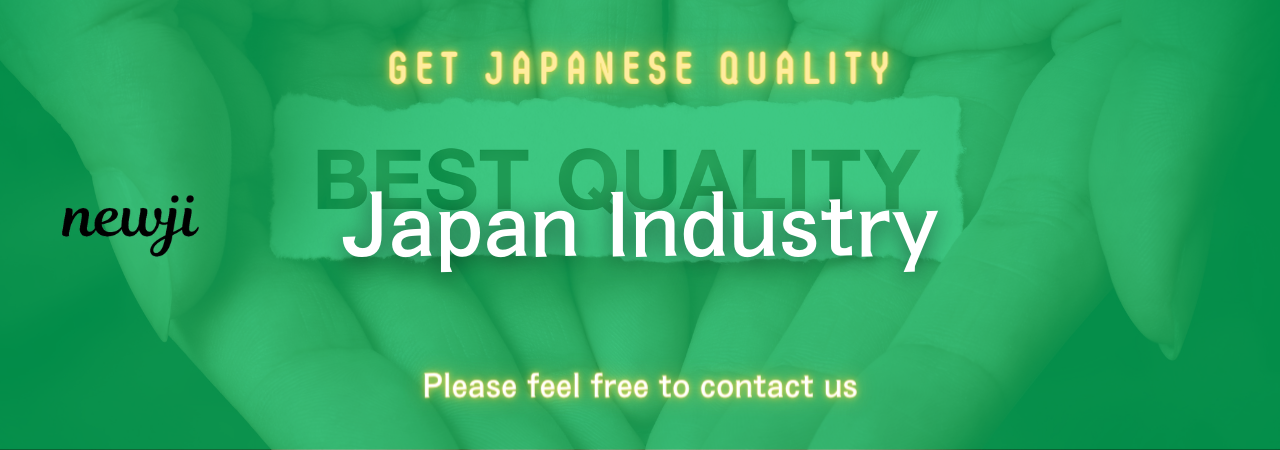
目次
What is Python?
Python is a popular programming language known for its simplicity and readability.
It was created by Guido van Rossum and first released in 1991.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Its syntax is designed to be clean and easy to understand, making it a great choice for beginners and experienced programmers alike.
Why Learn Python?
Python is widely used in many different areas such as web development, data analysis, artificial intelligence, scientific computing, and more.
Its versatility is one of the main reasons for its popularity.
As a beginner-friendly language, Python is an excellent starting point for new programmers.
Furthermore, because of its widespread use, there are a lot of resources and community support available for learning Python.
Python in Web Development
In web development, Python is used to create server-side web applications.
Frameworks like Django and Flask are popular for building web applications efficiently.
They provide tools and libraries that make it easier to handle tasks like database interaction, URL routing, and page rendering.
Python in Data Analysis
Python has become a big player in data analysis and data science.
With libraries like Pandas, NumPy, and Matplotlib, Python makes it easier to manipulate and visualize data.
It allows data analysts and scientists to quickly process large datasets and perform complex calculations.
Python for Artificial Intelligence
Artificial Intelligence and machine learning are fields where Python truly shines.
Libraries like TensorFlow, Keras, and Scikit-learn provide powerful tools for building and training models for predictive analytics.
Python’s simplicity allows developers to focus more on solving complex problems rather than on writing complex code.
Getting Started with Python
To begin programming in Python, you need to install the Python interpreter on your computer.
This can be done by downloading it from the official Python website.
Once installed, you can write Python code using an Integrated Development Environment (IDE) like PyCharm, VSCode, or even in a simple text editor.
Writing Your First Python Program
After setting up your environment, you can start writing your first Python program.
Here’s a simple example:
“`python
print(“Hello, World!”)
“`
This line of code is a traditional first program that outputs the text “Hello, World!” to the screen.
It demonstrates how easy it is to write and run Python code.
Understanding Python Syntax
Python uses indentation to define code blocks, unlike other languages that use braces or specific keywords.
This means that you’ll need to be careful with the number of spaces or tabs at the beginning of lines in your code.
For example, consider this basic `if` statement:
“`python
if 5 > 2:
print(“Five is greater than two!”)
else:
print(“Five is not greater than two.”)
“`
The indentation in the code helps the interpreter understand which lines of code belong to which control structure.
Python Basics
Before diving into complex programming, it’s essential to understand Python’s basic concepts.
Variables
Variables in Python are used to store data that can be different types, such as integers, strings, or more complex data structures like lists or dictionaries.
Example:
“`python
x = 5
y = “Hello”
print(x)
print(y)
“`
This code assigns the integer 5 to the variable `x` and the string “Hello” to the variable `y`.
Data Types
Python includes several built-in data types:
– Integers: Numbers without decimals (e.g., `3`, `100`, `-5`).
– Floats: Numbers with decimals (e.g., `3.14`, `0.5`).
– Strings: Text data (e.g., `”Hello, World!”`).
– Booleans: Represent True or False values.
– Lists: Ordered collections of items (e.g., `[1, 2, 3]`).
– Dictionaries: Collections of key-value pairs (e.g., `{“key”: “value”}`).
Working with Functions
Functions in Python help you organize your code into reusable pieces.
Defining a Function
You define a function using the `def` keyword, followed by the function name and parentheses:
“`python
def greet(name):
print(“Hello, ” + name)
“`
This function takes one argument, `name`, and prints a greeting.
Calling Functions
To call a function, use its name followed by parentheses:
“`python
greet(“Alice”)
greet(“Bob”)
“`
This will output:
“`
Hello, Alice
Hello, Bob
“`
Loops and Iteration
Loops allow you to execute a block of code multiple times.
For Loop
A `for` loop iterates over a sequence:
“`python
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
“`
This code prints each fruit in the list.
While Loop
A `while` loop repeats as long as a condition is true:
“`python
i = 1
while i < 6:
print(i)
i += 1
```
This loop will print numbers 1 through 5.
Conclusion
Python is a versatile and accessible programming language suitable for a variety of applications.
Its simple syntax and extensive libraries make it an excellent choice for both beginners and professionals.
By mastering Python’s basics, such as variables, data types, functions, and loops, you’ll be well-equipped to explore more complex programming topics and projects.
Whether you’re interested in web development, data analysis, or artificial intelligence, Python provides the tools you need to succeed.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)