- お役立ち記事
- Python and OpenAI Integration for Chatbot Development
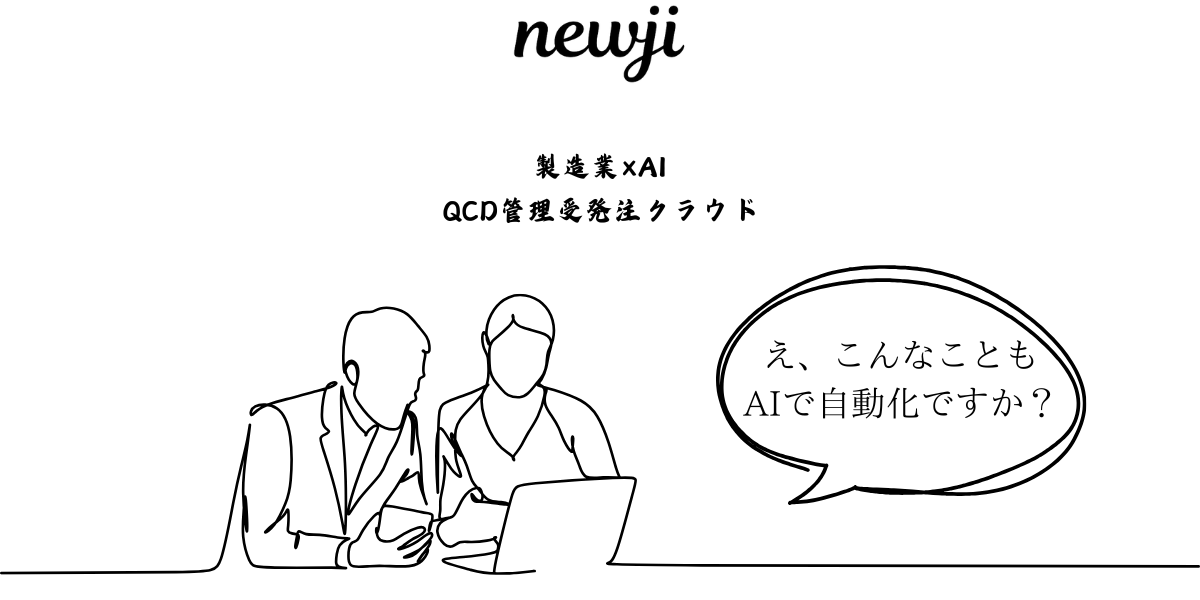
Python and OpenAI Integration for Chatbot Development
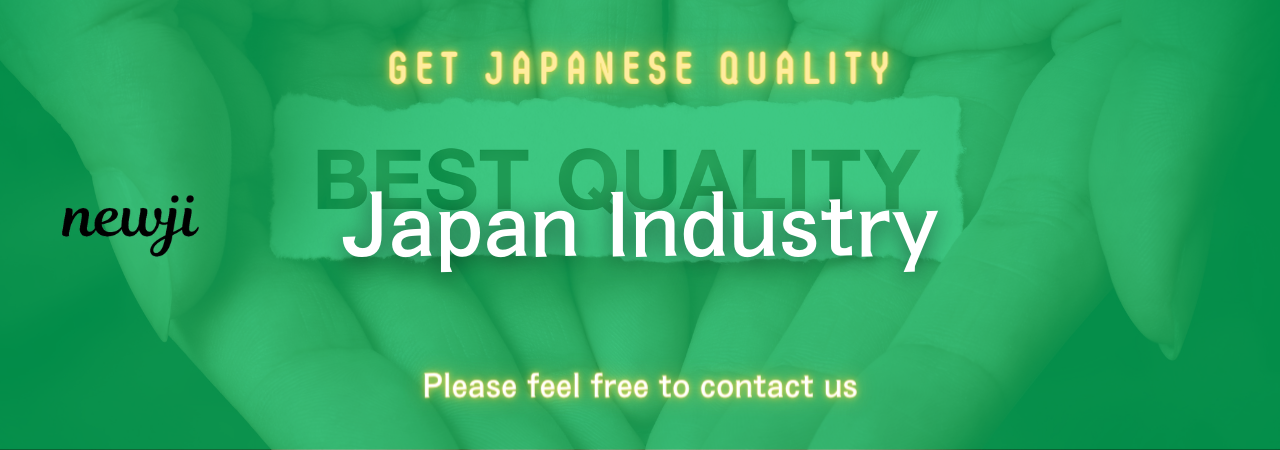
目次
Introduction to Python and OpenAI
Python, a versatile and powerful programming language, is often favored by developers for its simplicity and readability.
Its wide range of libraries and extensive community support make it an ideal choice for developing various applications, including chatbots.
On the other hand, OpenAI, a leading research organization, specializes in artificial intelligence and machine learning models, providing powerful tools like the GPT series for natural language understanding and generation.
Integrating Python with OpenAI can open up numerous possibilities for developing intelligent chatbots that can interact seamlessly with users.
Why Use Python for Chatbot Development?
Python is considered the language of choice for many developers when it comes to building chatbots.
There are several reasons for this:
Simplicity and Readability
Python’s syntax is simple and clear, making it accessible to both beginners and experienced developers.
This simplicity aids in writing clean and maintainable code, which is crucial in developing scalable chatbot applications.
Rich Ecosystem
Python offers a vast array of libraries and frameworks, such as TensorFlow, PyTorch, and NLTK.
These tools provide pre-built functions and capabilities that are essential for machine learning, natural language processing, and data analysis, making the chatbot development process more efficient.
Community Support
Python has a large and active community, which means there is extensive documentation and a wealth of resources available online.
Developers can easily find solutions to their problems and share knowledge through community platforms.
OpenAI’s Role in Chatbot Development
OpenAI has been at the forefront of AI research, providing state-of-the-art models capable of understanding and generating human-like text.
The OpenAI GPT (Generative Pre-trained Transformer) models are particularly famous for their natural language understanding and generation capabilities.
These models can be easily integrated into Python applications, enabling developers to create sophisticated, AI-driven chatbots.
GPT’s Natural Language Understanding
GPT models are designed to understand the nuances of human language, allowing them to carry out complex conversations.
They can comprehend context, answer questions, and even generate creative content, making them ideal for chatbot applications.
Scalability and Flexibility
OpenAI models can be easily scaled to handle different workloads, ensuring that chatbots remain responsive and efficient regardless of the user base size.
They are also highly flexible, allowing developers to fine-tune them for specific domains or tasks, enhancing the chatbot’s effectiveness in delivering targeted responses.
Integrating Python with OpenAI for Chatbot Development
Combining Python with OpenAI provides developers with the tools necessary to build intelligent and interactive chatbots.
Here’s how you can leverage this integration to create a functional chatbot.
Setting Up the Environment
Before diving into integration, ensure that you have the necessary environment set up.
You should have Python installed on your machine, along with a package manager like pip to install required libraries.
Create a virtual environment for your project to manage dependencies efficiently.
This can be done using the `venv` module in Python.
“`bash
python -m venv my_chatbot_env
source my_chatbot_env/bin/activate # On Windows use `my_chatbot_env\Scripts\activate`
“`
Installing Required Libraries
You’ll need the OpenAI Python client library to access their models.
You can install it using pip:
“`bash
pip install openai
“`
Additionally, you may want to install libraries for making HTTP requests and handling JSON data:
“`bash
pip install requests
pip install json
“`
Configuring OpenAI API
To interact with OpenAI’s models, you’ll need an API key.
Sign up on the OpenAI platform if you haven’t already, and obtain your API key from the API section.
In your Python script, configure the OpenAI API client by setting your API key:
“`python
import openai
openai.api_key = ‘your-api-key-here’
“`
Building the Chatbot
To create a chatbot, you’ll need a function that sends and receives messages from the OpenAI model.
You can achieve this by utilizing the `openai.Completion.create` method to make requests and handle responses.
Here’s a basic template:
“`python
def chat_with_openai(prompt):
response = openai.Completion.create(
engine=”text-davinci-003″,
prompt=prompt,
max_tokens=150
)
return response.choices[0].text.strip()
“`
You can use this function in a loop to continuously interact with the user:
“`python
def main():
print(“Hello! I’m your chatbot. How can I help you today?”)
while True:
user_input = input(“You: “)
if user_input.lower() in [“exit”, “quit”]:
print(“Goodbye!”)
break
bot_response = chat_with_openai(user_input)
print(f”Bot: {bot_response}”)
if __name__ == “__main__”:
main()
“`
This simple setup allows your chatbot to read user inputs, send them to OpenAI, and print out the generated responses.
Enhancing the Chatbot Functionality
With the basic chatbot running, you can explore ways to enhance its functionality and make it more robust.
Context Management
Implementing context management can allow the chatbot to hold a deeper context about ongoing conversations.
By appending past interactions to the prompt, you can retain a coherent conversation across multiple turns.
Fine-Tuning Models
Consider fine-tuning OpenAI models with domain-specific data.
This process customizes the model responses, making them more relevant and accurate for specific applications.
Incorporating Feedback
Deploying a feedback loop enables the chatbot to learn from user interactions.
By analyzing feedback, you can iteratively improve the bot’s conversational abilities.
Conclusion
Integrating Python with OpenAI for chatbot development presents an exciting opportunity to create highly interactive and intelligent interfaces.
Whether it’s for customer service, data gathering, or entertainment, using Python alongside OpenAI’s powerful models can significantly enhance the capabilities of your chatbot.
By following the steps outlined above, you can embark on a journey towards building a more engaging and responsive chatbot that meets the demands of modern-day users.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)