- お役立ち記事
- Python grammar
Python grammar
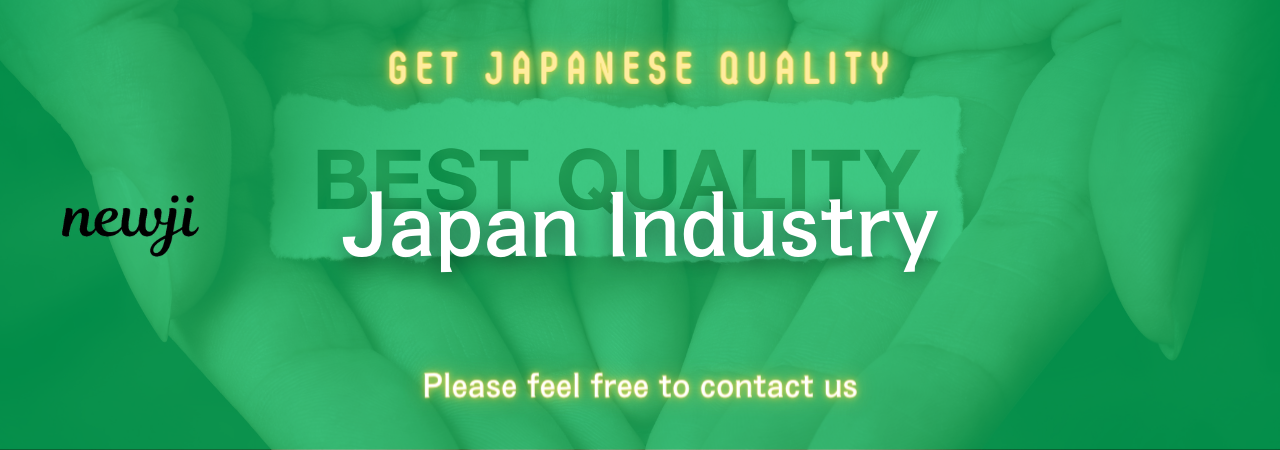
目次
Introduction to Python
Python is a popular programming language known for its simplicity and readability.
Its syntax is straightforward, making it an excellent choice for beginners and experienced programmers alike.
In this article, we’ll explore the basic grammar of Python, helping you understand its structure and how to write clean and efficient code.
Basic Elements of Python Syntax
Indentation
One of the key elements of Python’s grammar is indentation.
Unlike other programming languages, Python uses indentation to define the structure and hierarchy of the code.
This means that properly aligning your code is crucial to ensure it runs correctly.
Each indented block represents a suite of logic, such as the body of loops, conditionals, and function definitions.
Variables and Data Types
Variables in Python are used to store data, and they can be assigned with an equal sign (`=`).
Python is dynamically typed, meaning you don’t need to declare a variable’s type explicitly.
Some common data types include integers (`int`), floating-point numbers (`float`), strings (`str`), and booleans (`bool`).
Example:
“`python
age = 10
pi = 3.14
name = “Alice”
is_student = True
“`
Comments
Comments are an essential part of code for adding explanations and making your code more readable.
In Python, a comment starts with the hash mark (`#`) and extends to the end of the line.
Comments are ignored by the Python interpreter.
Example:
“`python
# This is a single-line comment
“`
Operators
Python provides a wide array of operators to perform operations on variables and values.
These include arithmetic operators like `+`, `-`, `*`, `/`; comparison operators such as `==`, `!=`, `>`, `<`; and logical operators like `and`, `or`, `not`.
Example:
```python
# Arithmetic
sum = 5 + 3
difference = 10 - 2
# Comparison
is_greater = 7 > 3
# Logical
result = True and False
“`
Control Flow
Conditional Statements
Python uses `if`, `elif`, and `else` statements to make decisions in code.
These control structures allow you to execute certain blocks of code based on specific conditions.
Example:
“`python
age = 18
if age >= 18:
print(“You are an adult.”)
elif age > 12:
print(“You are a teenager.”)
else:
print(“You are a child.”)
“`
Loops
Loops are used to execute a block of code repeatedly.
Python provides two types of loops: `for` loops and `while` loops.
A `for` loop iterates over a sequence, such as a list, string, or range.
Example:
“`python
for i in range(5):
print(“Iteration”, i)
“`
A `while` loop runs as long as a condition evaluates to `True`.
Example:
“`python
count = 0
while count < 5:
print("Count is", count)
count += 1
```
Functions
Functions are reusable blocks of code that perform a specific task.
You define a function using the `def` keyword, followed by the function name and parentheses.
Example:
“`python
def greet(name):
return “Hello, ” + name
print(greet(“Alice”))
“`
Functions can also have default parameters, making them versatile and easy to use.
You can pass multiple arguments and even return multiple values from a function.
Data Structures
Python provides several built-in data structures to store and manage collections of data.
Lists
Lists are ordered and mutable sequences, making them versatile tools for handling collections of items.
Example:
“`python
fruits = [“apple”, “banana”, “cherry”]
“`
Tuples
Tuples are similar to lists but are immutable, meaning once created, their elements cannot be changed.
Example:
“`python
point = (10, 20)
“`
Dictionaries
Dictionaries store data in key-value pairs, allowing for efficient data retrieval.
Example:
“`python
student = {“name”: “Bob”, “age”: 20}
“`
Sets
Sets are unordered collections of unique elements, useful for membership testing and eliminating duplicates.
Example:
“`python
numbers = {1, 2, 3, 3}
“`
Conclusion
Python’s straightforward grammar makes it an accessible language for new and experienced programmers.
By mastering the basics of Python syntax, you can write clean and effective code for a wide range of applications.
As you continue learning, you’ll discover Python’s extensive libraries and features that make it a powerful tool in the world of programming.
Keep practicing, experimenting, and building projects to enhance your Python skills.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)