- お役立ち記事
- Python techniques to remember
Python techniques to remember
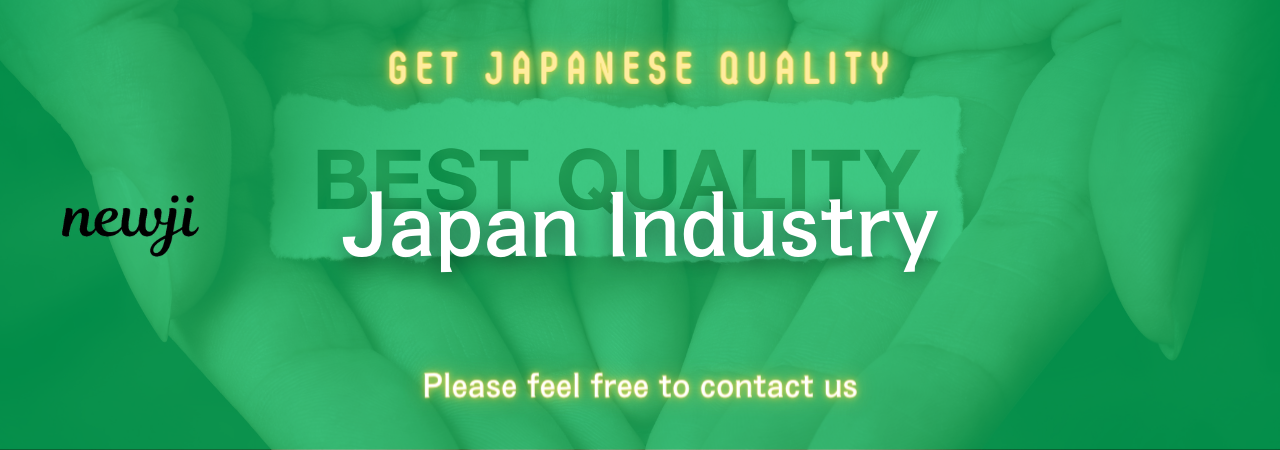
目次
Understanding Python and Its Importance
Python is a powerful and popular programming language used worldwide.
It’s known for its simplicity and versatility, making it an excellent choice for beginners and experienced programmers alike.
Python is often used in web development, data analysis, artificial intelligence, scientific computing, and more.
Its readability and straightforward syntax help even elementary-level students start coding.
Python is an open-source language, which means anyone can use and modify it for free.
This accessibility has contributed to its widespread adoption and the development of a vast community that constantly improves its ecosystem.
Essential Python Techniques for Beginners
1. Understanding Variables and Data Types
Variables are like containers that store data in a program.
In Python, you don’t need to declare the data type explicitly; Python figures it out based on the assigned value.
Common data types include:
– **Integers** (whole numbers)
– **Floats** (decimal numbers)
– **Strings** (text)
– **Booleans** (True or False)
2. Mastering Basic Operators
Operators are symbols that perform operations on variables and values.
Basic operators in Python include:
– **Arithmetic operators**: perform mathematical operations.
Examples: `+`, `-`, `*`, `/`
– **Comparison operators**: compare two values.
Examples: `==`, `!=`, `>`, `<`
- **Logical operators**: combine conditional statements.
Examples: `and`, `or`, `not`
3. Working with Lists and Tuples
– **Lists** are ordered collections that are mutable, meaning they can be changed.
You can append, remove, or modify elements.
Example:
“`python
my_list = [1, 2, 3, 4]
my_list.append(5)
“`
– **Tuples**, unlike lists, are immutable.
Once created, their content cannot be changed.
Example:
“`python
my_tuple = (1, 2, 3, 4)
“`
4. Utilizing Dictionaries
Dictionaries store data in key-value pairs.
They are similar to lists but more efficient when you need to find data quickly using keys.
Example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 25}
print(my_dict[‘name’]) # Outputs: Alice
“`
Intermediate Python Techniques
1. Understanding Functions
Functions are blocks of reusable code that perform specific tasks.
Defining functions helps make programs organized and reduces redundancy.
Example of a function:
“`python
def greet(name):
return f”Hello, {name}!”
print(greet(“Bob”)) # Outputs: Hello, Bob!
“`
2. Working with Loops
Loops allow you to repeat a set of instructions.
There are two main types:
– **For loop**: iterates over a sequence (like a list, tuple, or string).
Example:
“`python
for number in [1, 2, 3]:
print(number)
“`
– **While loop**: continues executing a block of code as long as a condition is true.
Example:
“`python
i = 1
while i < 4:
print(i)
i += 1
```
3. List Comprehensions
This is a concise way to create lists based on existing lists.
It is more readable and often faster than traditional loops.
Example:
“`python
squares = [x**2 for x in range(5)]
print(squares) # Outputs: [0, 1, 4, 9, 16]
“`
Advanced Python Techniques
1. Understanding Modules and Packages
Modules are Python files containing code that can be reused across various programs.
Packages are collections of modules.
This system encourages code reusability and organization.
To use a module, you must import it:
“`python
import math
print(math.sqrt(16)) # Outputs: 4.0
“`
2. Decorators
Decorators are a powerful tool for modifying the behavior of a function or method.
They are often used to add code before and after a function runs.
Example:
“`python
def my_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
@my_decorator
def say_hello():
print(“Hello!”)
say_hello()
“`
3. Understanding File Operations
Python can handle files operations, such as reading and writing to files.
This is crucial for logging data or interacting with databases.
Example of reading a file:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
Conclusion
Mastering Python techniques is essential for anyone looking to become proficient in programming.
From basic operations like handling variables and loops to advanced concepts like decorators and file handling, Python offers a vast array of tools to help you achieve your goals.
Its simplicity makes it accessible, yet its depth allows you to build robust and scalable solutions for real-world applications.
By continuously practicing and exploring new techniques, you can leverage Python’s full potential to innovate and create in the digital world.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)