- お役立ち記事
- Smart contract implementation with Solidity
Smart contract implementation with Solidity
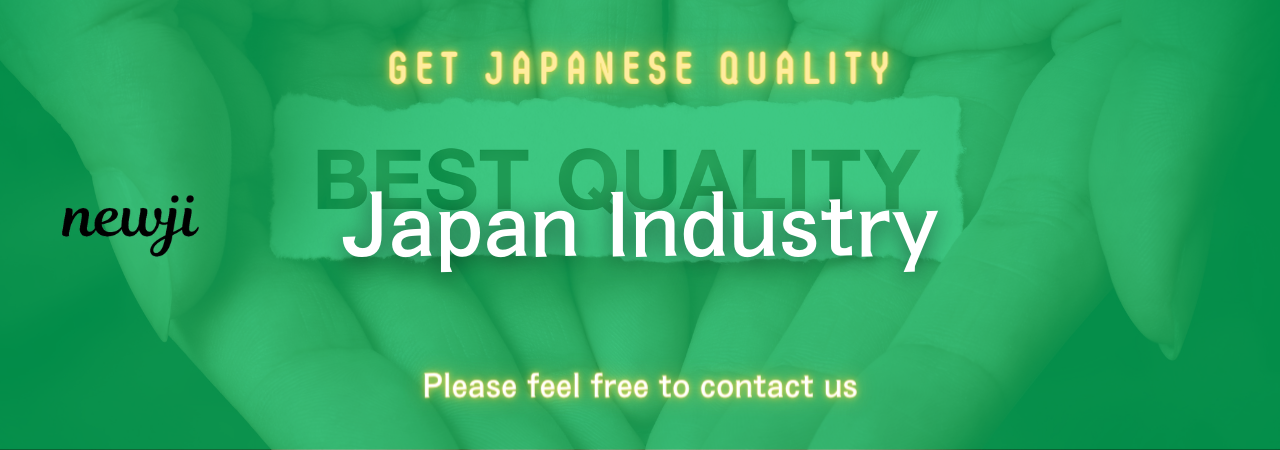
目次
What is a Smart Contract?
A smart contract is a self-executing contract with the terms of the agreement directly written into lines of code.
They run on blockchain technology, allowing users to execute transactions without the need for a central authority or intermediary.
Smart contracts are immutable and transparent, meaning once they are deployed on the blockchain, they cannot be altered, and all transactions are visible to the network.
Introduction to Solidity
Solidity is a programming language specifically designed for developing smart contracts on the Ethereum blockchain.
It is similar to JavaScript, making it relatively accessible for those familiar with the latter.
The language allows developers to write programs that run exactly as programmed without downtime, fraud, control, or interference.
Why Use Solidity?
Solidity is the go-to language for Ethereum because of its ability to provide a secure and tested framework for smart contracts.
It supports complex user-defined types, libraries, and inheritance, making it flexible and functional for various applications.
Furthermore, Solidity is constantly updated to improve security and efficiency, ensuring developers can leverage the latest advancements in blockchain technology.
Setting Up Your Environment
Before you start writing a smart contract in Solidity, you need to set up your development environment.
First, install Node.js and npm (Node Package Manager) on your machine.
They are essential for managing project dependencies.
Next, you’ll need the Ethereum development framework, Truffle.
Install it using the command: `npm install -g truffle`.
Truffle provides a suite of tools for writing, testing, and deploying smart contracts.
For a more user-friendly interface, you can also use Remix, a web-based IDE for Solidity development.
It offers features like syntax highlighting, compilation warnings, and debugging.
Writing Your First Smart Contract
Let’s start with writing a simple smart contract.
Create a new file with a `.sol` extension, e.g., `SimpleStorage.sol`.
Here’s a basic example:
“`solidity
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
“`
Breaking Down the Code
– `pragma solidity ^0.8.0;` specifies the version of Solidity.
This prevents issues from compiler changes.
– `contract SimpleStorage` defines a new contract named SimpleStorage.
– `uint256 storedData;` declares a state variable `storedData` of type `uint256`, which stores unsigned integers.
– `function set(uint256 x) public { … }` a public function to store the value of `x` in `storedData`.
– `function get() public view returns (uint256) { … }` a public function to retrieve the value of `storedData`.
Deploying Your Smart Contract
Once your contract is written, it’s time to deploy it to the blockchain.
If you’re using Remix, click the “Deploy & Run Transactions” tab.
Select “Injected Web3” to connect to a network like Ropsten or Rinkeby.
For Truffle, open your project folder in the terminal and run: `truffle migrate`.
Ensure you’re connected to a test network, as deploying costs gas fees.
Testing Your Smart Contract
After deployment, testing is crucial to ensure your smart contract functions correctly.
Truffle offers a testing suite using Mocha and Chai.
Create a test file in the `test` directory with a `.js` extension.
Here’s an example test:
“`javascript
const SimpleStorage = artifacts.require(“SimpleStorage”);
contract(“SimpleStorage”, () => {
it(“should store the value 89”, async () => {
const simpleStorageInstance = await SimpleStorage.deployed();
// Set value of 89
await simpleStorageInstance.set(89, { from: accounts[0] });
// Get stored value
const storedData = await simpleStorageInstance.get.call();
assert.equal(storedData, 89, “The value 89 was not stored.”);
});
});
“`
Run tests with: `truffle test`.
This checks if your smart contract stores data correctly.
Best Practices in Solidity Development
When developing with Solidity, adhere to best practices to ensure security and efficiency.
Security Considerations
Write audits through thorough testing and code reviews.
Always be cautious of reentrancy attacks and ensure proper input validation.
Update your contracts promptly to include security patches and improvements.
Gas Optimization
Gas costs are significant in Ethereum.
Optimize your contracts by minimizing storage use and avoiding redundant code.
Use more efficient data structures like mappings over arrays when possible.
Adopting New Standards
Stay updated with the Ethereum community’s standards and improvements.
Implement interfaces like ERC20 or ERC721 if your contract needs to integrate with other token platforms.
Conclusion
Solidity and smart contract development open new possibilities for decentralized applications on blockchain technology.
By following the steps outlined above, you can start implementing smart contracts that are not only functional but also efficient and secure.
With ongoing advancements in Solidity and Ethereum, developers can explore innovative solutions in various industries, paving the way for a decentralized future.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)