- お役立ち記事
- Practical course on Excel data processing and automation programming using Python
Practical course on Excel data processing and automation programming using Python
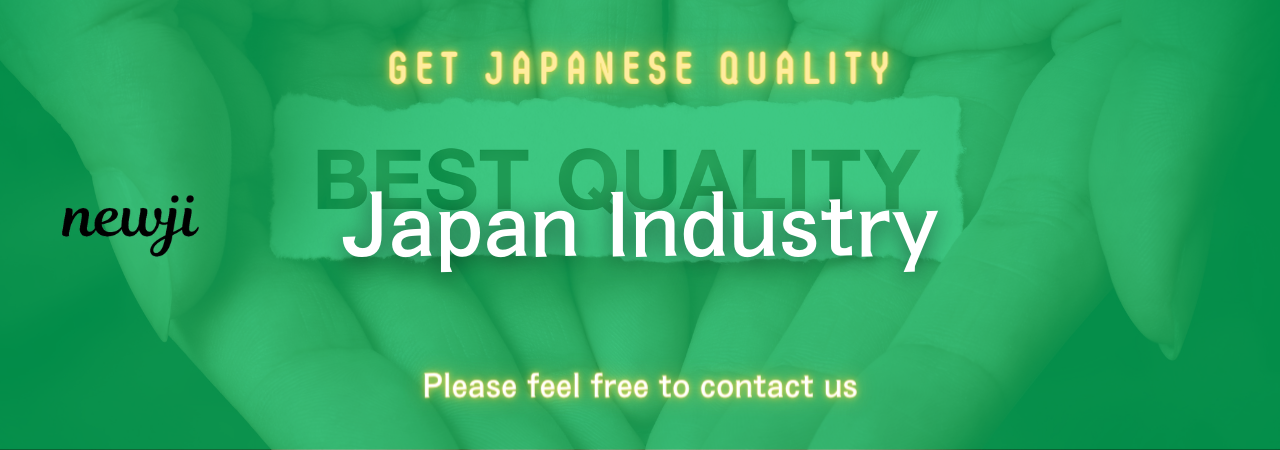
目次
Introduction to Excel Data Processing with Python
Excel is one of the most widely used tools for data management, analysis, and visualization.
However, as your data grows in complexity and volume, manual Excel operations can become cumbersome and error-prone.
This is where Python, a versatile and powerful programming language, comes to the rescue.
Python offers excellent libraries that can automate and enhance Excel data processing.
In this guide, we will explore practical ways to leverage Python for Excel data processing and automation.
Whether you’re a beginner or an experienced Excel user, this course will introduce you to simple automation techniques that can save you time and reduce errors.
Getting Started with Python
Before diving into the specifics of Excel automation, it’s essential to set up your Python environment.
If you haven’t installed Python yet, you can start by downloading the latest version from the official Python website.
Popular integrated development environments (IDEs) like PyCharm, Visual Studio Code, or even Jupyter Notebook provide excellent platforms for Python development.
With Python installed, you’ll also need some packages specifically designed for Excel manipulation.
Two of the most popular libraries are `pandas` and `openpyxl`.
You can easily install them via pip by typing:
“`
pip install pandas openpyxl
“`
Reading and Writing Excel Files
One of the most common tasks is reading and writing Excel data.
Python makes this straightforward with the `pandas` library.
`pandas` provides a powerful `DataFrame` object that handles tabular data effortlessly.
Reading Excel Files
To read an Excel file, you can use the `read_excel` function from `pandas`:
“`python
import pandas as pd
# Load the Excel file
df = pd.read_excel(‘your_file.xlsx’, sheet_name=’Sheet1′)
# Display the first few rows
print(df.head())
“`
This snippet reads data from a sheet named ‘Sheet1’ in ‘your_file.xlsx’ and displays the first few rows, allowing you to verify the data structure quickly.
Writing Excel Files
Similarly, writing data to Excel files is just as easy:
“`python
# Save the DataFrame to an Excel file
df.to_excel(‘output_file.xlsx’, index=False)
“`
This code will save your DataFrame `df` to ‘output_file.xlsx’, excluding the DataFrame’s index to keep the output clean.
Data Cleaning and Preparation
Cleaning data is a crucial step in any data processing task.
Python, particularly `pandas`, offers a suite of functions for data cleaning and preparation before analysis or visualization.
Removing Missing Values
Missing values are common in datasets and can be dealt with using `pandas`:
“`python
# Remove rows with missing values
df_clean = df.dropna()
# Fill missing values with a default value
df_filled = df.fillna(0)
“`
These commands provide two options: removing rows with missing data or filling them with a specified value.
Data Transformation
Sometimes data must be transformed to extract useful insights.
With `pandas`, you can perform operations like adding new columns, changing data types, or applying complex transformations:
“`python
# Add a new column calculating the total price
df[‘Total Price’] = df[‘Quantity’] * df[‘Unit Price’]
“`
This example demonstrates using an arithmetic operation to create a new column in the DataFrame.
Automating Excel Tasks with Python
One of Python’s strengths is its ability to automate repetitive tasks, making your workflow more efficient.
Batch Processing Multiple Excel Files
When dealing with multiple Excel files, manually processing each file is inefficient.
Python can batch process files in a directory:
“`python
import os
directory = ‘excel_files/’
for filename in os.listdir(directory):
if filename.endswith(‘.xlsx’):
df = pd.read_excel(os.path.join(directory, filename))
# Perform operations on the DataFrame
df.to_excel(f’processed_{filename}’, index=False)
“`
This script reads all Excel files in the ‘excel_files/’ directory, performs operations, and saves them with a ‘processed_’ prefix.
Automating Report Generation
Generating reports can be streamlined using Python scripts that create charts and summaries:
“`python
import matplotlib.pyplot as plt
# Generate a bar chart for sales data
sales_data = df.groupby(‘Product’)[‘Total Price’].sum()
sales_data.plot(kind=’bar’)
plt.title(‘Total Sales by Product’)
plt.show()
“`
This code automatically groups sales data by product and generates a bar chart visualizing the results.
Advanced Excel Automation Techniques
As you become comfortable with basic tasks, you can explore more advanced automation techniques.
Using VBA Scripts with Python
Python can interact with VBA (Visual Basic for Applications) scripts to extend Excel’s functionality.
The `pywin32` library allows Python to run VBA macros, providing you with the best of both worlds.
“`python
import win32com.client
excel = win32com.client.Dispatch(“Excel.Application”)
workbook = excel.Workbooks.Open(‘your_file.xlsm’)
# Run a macro stored in the workbook
excel.Application.Run(“your_macro”)
workbook.Close(SaveChanges=True)
excel.Quit()
“`
This code snippet demonstrates opening an Excel macro-enabled workbook and executing a VBA macro using Python.
Scheduling Automated Tasks
For entirely hands-off automation, you can schedule Python scripts to run at specific intervals using task schedulers like Windows Task Scheduler or cron jobs on Unix-based systems.
Conclusion
Integrating Python with Excel creates a powerful toolset for data processing and automation.
By mastering the techniques outlined in this guide, you can execute complex analyses, generate reports, and handle large datasets effortlessly.
Remember, practice and exploration are crucial to becoming proficient in Python-based Excel automation.
As you continue experimenting with different scenarios, you will discover innovative ways to streamline your operations and enhance your data processing capabilities.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)