- お役立ち記事
- Python programming and practice for image processing and machine learning
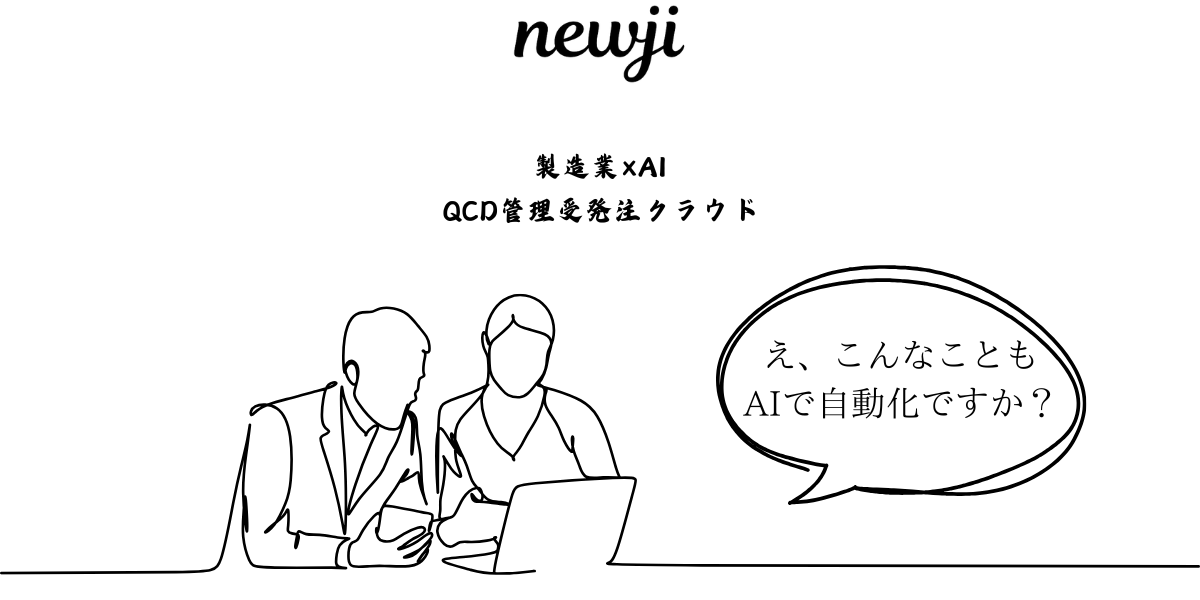
Python programming and practice for image processing and machine learning
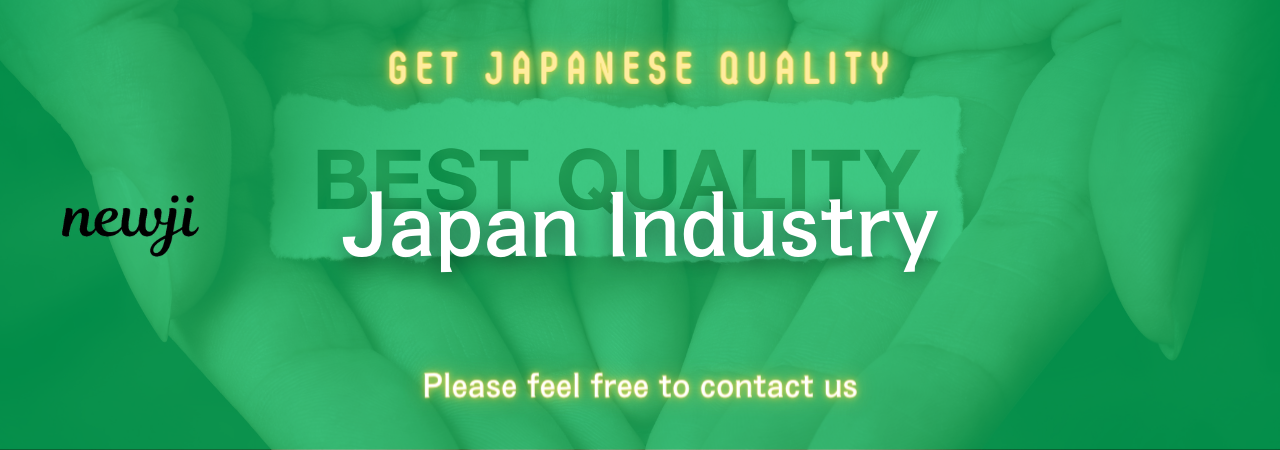
目次
Introduction to Python Programming
Python is a versatile and powerful programming language widely used in various fields, including image processing and machine learning.
Its simplicity and readability make it a popular choice for beginners and professionals alike.
In this article, we will explore the basics of Python programming and how it can be applied to image processing and machine learning projects.
Getting Started with Python
Before diving into image processing and machine learning, it’s essential to get familiar with Python’s basic syntax and functionality.
Python can be installed on any operating system, including Windows, macOS, and Linux.
You can download the latest version from the official Python website, and follow the installation instructions provided.
Once installed, you can use Python’s interactive shell, IDLE, or install an Integrated Development Environment (IDE) such as PyCharm or Anaconda for a more robust development experience.
These tools offer features like code completion, debugging, and library management that can greatly aid in developing Python projects.
Understanding Python Syntax
Python’s syntax is clean and intuitive, making it an excellent language for beginners.
Here are a few key components of Python syntax to get you started:
– **Variables and Data Types**: Python supports various data types including integers, floats, strings, lists, and dictionaries. Assigning a variable is as simple as typing `x = 10` or `name = “Alice”`.
– **Control Structures**: Python uses indentation to define the scope of loops and conditions. For example:
“`
if x > 5:
print(“x is greater than 5”)
else:
print(“x is 5 or less”)
“`
– **Functions**: Functions in Python are defined using the `def` keyword. For example:
“`
def add(a, b):
return a + b
“`
Python Libraries for Image Processing
Image processing is a crucial aspect of many machine learning projects, and Python provides a wealth of libraries to handle these tasks efficiently.
Two of the most commonly used libraries are OpenCV and Pillow.
OpenCV
OpenCV (Open Source Computer Vision Library) is a powerful library that supports various computer vision tasks.
With OpenCV, you can perform operations like image transformation, filtering, and face detection.
Here’s a simple example of how to read and display an image using OpenCV:
“`python
import cv2
# Read an image from file
image = cv2.imread(‘example.jpg’)
# Display the image in a window
cv2.imshow(‘Image’, image)
cv2.waitKey(0)
cv2.destroyAllWindows()
“`
OpenCV’s vast functionality allows for complex image processing pipelines suitable for any project.
Pillow
Pillow is another popular library for image manipulation in Python.
It’s a fork of the original PIL (Python Imaging Library) and is commonly used for basic image operations.
Here’s an example of how to open and save an image using Pillow:
“`python
from PIL import Image
# Open an existing image
image = Image.open(‘example.jpg’)
# Save it to a new file
image.save(‘new_example.jpg’)
“`
Pillow is user-friendly and provides easy methods for tasks like cropping, resizing, and filtering images.
Python for Machine Learning
Machine learning involves teaching computers to learn from data and make predictions or decisions.
Python provides robust libraries and frameworks to facilitate the development of machine learning models, with some notable ones being Scikit-Learn and TensorFlow.
Scikit-Learn
Scikit-Learn is a user-friendly library that includes simple and efficient tools for data mining and data analysis, built on NumPy, SciPy, and Matplotlib.
It’s an excellent choice for beginners and includes algorithms for classification, regression, clustering, and more.
Here’s a quick example of how to train a basic linear regression model with Scikit-Learn:
“`python
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([[1, 1], [2, 2], [3, 3]])
y = np.array([1, 2, 3])
# Create a linear regression model
model = LinearRegression()
# Fit the model
model.fit(X, y)
# Make a prediction
prediction = model.predict(np.array([[4, 4]]))
print(“Predicted value:”, prediction)
“`
Scikit-Learn simplifies the process of deploying machine learning models, making it accessible to developers with varying skill levels.
TensorFlow
TensorFlow is a powerful open-source library developed by Google for deep learning applications.
It’s used extensively for training neural networks, which are instrumental for tasks like image recognition and natural language processing.
Here’s a basic example of how to create a neural network using TensorFlow:
“`python
import tensorflow as tf
# Define a simple sequential model
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(128, activation=’relu’, input_shape=(784,)),
tf.keras.layers.Dense(10, activation=’softmax’)
])
# Compile the model
model.compile(optimizer=’adam’,
loss=’sparse_categorical_crossentropy’,
metrics=[‘accuracy’])
# Assume `x_train` and `y_train` are the datasets
# Fit the model
model.fit(x_train, y_train, epochs=5)
“`
TensorFlow’s flexibility and scalability make it suitable for both simple and complex machine learning projects.
Conclusion
Python is an exceptional language for image processing and machine learning due to its simplicity and the vast ecosystem of libraries available.
Whether you’re implementing basic image manipulation or developing intricate machine learning models, Python has the tools and resources to support your efforts.
By mastering the basics of Python and exploring libraries like OpenCV, Pillow, Scikit-Learn, and TensorFlow, you’ll be well-equipped to tackle a wide range of projects in these exciting fields.
Happy coding!
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)