- お役立ち記事
- Basics of GNN (Graph Neural Network) and implementation points using Python
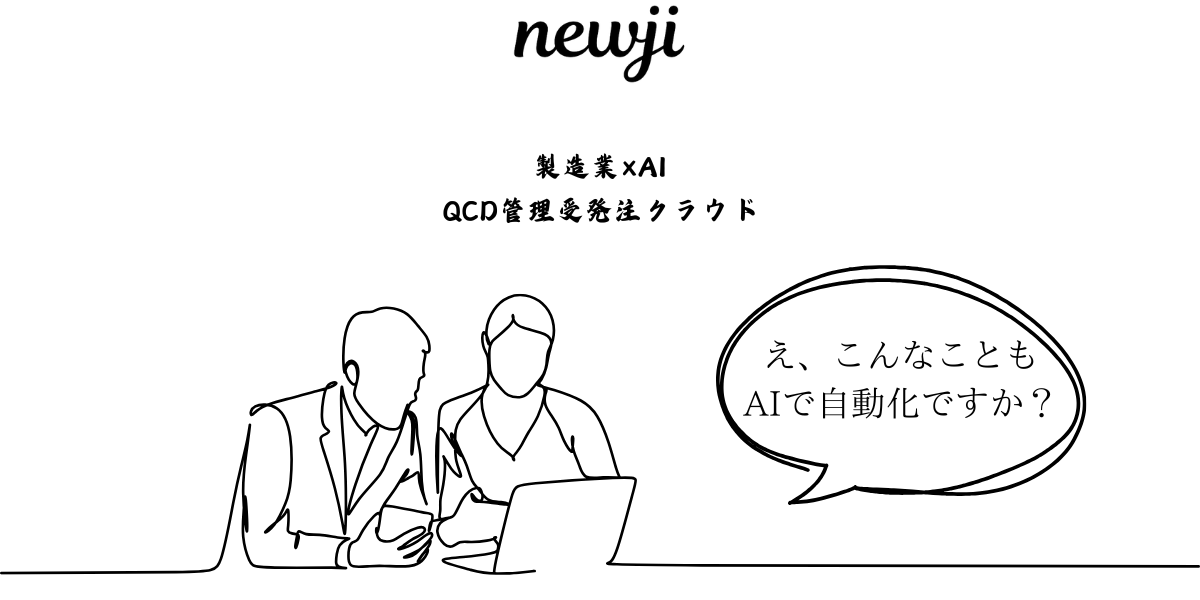
Basics of GNN (Graph Neural Network) and implementation points using Python
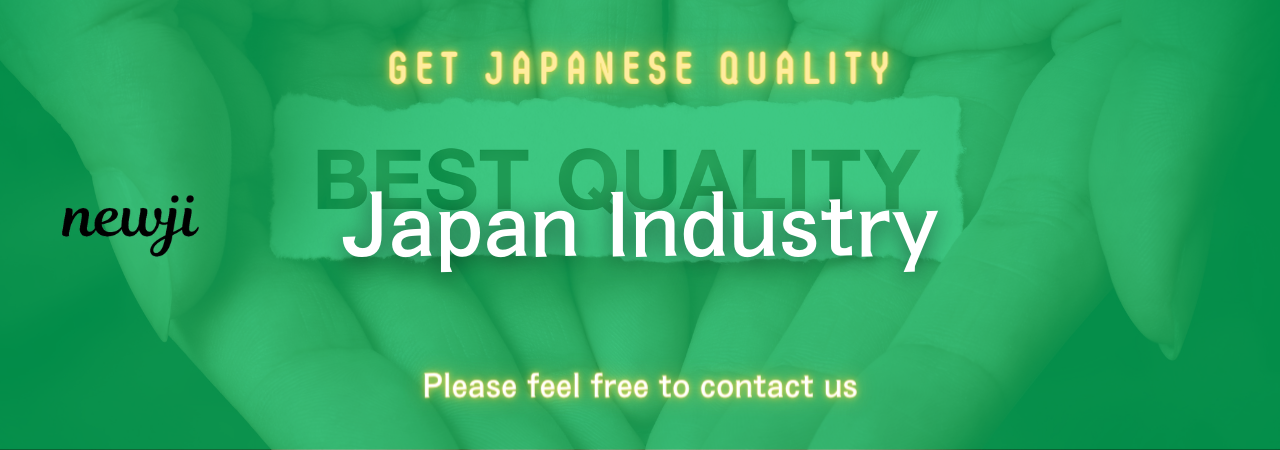
目次
Understanding Graph Neural Networks (GNN)
Graph Neural Networks (GNN) are a type of neural network designed to work with data that can be represented as graphs.
Graphs are structured data consisting of nodes (also known as vertices) and edges connecting them.
This structure makes GNNs useful for applications where the relationships between data points are as important as the data itself.
Unlike traditional neural networks that operate on grid-like data, such as images or sequences, GNNs can capture complex relationships and dependencies that are inherent in many real-world datasets.
Because of this capability, GNNs have found applications in various fields like social network analysis, recommendation systems, and bioinformatics.
Applications of GNN
GNNs excel in any domain where data can be structured as a graph.
Some typical applications include:
Social Network Analysis
In social networks, individuals are represented as nodes, and the relationships between them are edges.
GNNs help analyze community structures, predict missing links, and identify influential nodes.
Recommendation Systems
For recommendation engines, users and products form nodes, while edges represent interactions such as purchases or reviews.
GNNs can model these interactions to provide more personalized recommendations.
Bioinformatics
Proteins and other biological molecules often interact in complex networks.
Nodes might represent different molecules, while edges signify interactions.
GNNs help in predicting molecular functions or identifying potential drug targets.
Fraud Detection
In financial networks, users, transactions, and bank accounts form nodes, and transactions create edges.
GNNs can detect unusual patterns indicating fraudulent activities.
How Graph Neural Networks Work
The basic idea behind GNNs is to use a message-passing process to aggregate information from a node’s neighbors (or adjacent nodes) to update the node’s state.
This aggregation process enables the GNN to learn patterns and relationships from the data.
Message Passing
In the message-passing framework, each node gathers information from its neighbors and updates its state.
This process usually consists of two main steps:
1. **Aggregation**: Each node collects information from its neighboring nodes.
2. **Update**: The collected information is used to update the node’s current state.
This process is repeated for a fixed number of iterations, allowing information to propagate through the graph.
Readout Function
After message passing, a readout function aggregates node states into a single vector representation, which can be used for graph-level tasks like classification or regression.
Implementing GNNs with Python
Python, with its rich ecosystem of libraries, is a great choice for implementing GNNs.
You can leverage popular libraries such as PyTorch and DGL (Deep Graph Library) to get started.
Installing Required Libraries
Before implementing a GNN, ensure you have the necessary libraries installed.
You can use pip to install them:
“`python
pip install torch dgl
“`
Simple Graph Neural Network Implementation
Here’s a basic example of implementing a GNN using PyTorch and DGL:
“`python
import torch
import torch.nn as nn
import dgl
import dgl.function as fn
from dgl.nn import GraphConv
class SimpleGNN(nn.Module):
def __init__(self, in_feats, hidden_size, num_classes):
super(SimpleGNN, self).__init__()
self.conv1 = GraphConv(in_feats, hidden_size)
self.conv2 = GraphConv(hidden_size, num_classes)
def forward(self, g, features):
x = self.conv1(g, features)
x = torch.relu(x)
x = self.conv2(g, x)
return x
# Sample usage
# Assume ‘g’ is a dgl.DGLGraph object and ‘features’ is a node feature tensor
model = SimpleGNN(in_feats=features.size(1), hidden_size=16, num_classes=2)
output = model(g, features)
“`
Key Points in Implementation
– **Data Preparation**: Ensure your data is correctly formatted with nodes and edges.
Graph data often comes from edge lists or adjacency matrices.
In DGL, a graph object can be easily constructed using APIs like `dgl.graph()`.
– **Feature Importance**: Just like any neural network, the quality of features can greatly influence the performance of the GNN.
Node features can be attributes like user interactions, molecule bonds, or any other relevant data.
– **Hyperparameter Tuning**: Experiment with different hyperparameters such as the number of layers, hidden units, learning rates, and dropout rates to optimize your model.
Advantages and Limitations of GNNs
Advantages
– **Flexibility**: GNNs can handle various graph structures and are applicable to diverse domains.
– **Insightful Relationships**: They effectively capture dependencies and relationships in data that traditional models might miss.
Limitations
– **Scalability Issues**: Processing large graphs can be computationally intensive.
It’s crucial to consider hardware capabilities and efficient graph sampling techniques.
– **Data Complexity**: Preprocessing graph data and ensuring it is complete and accurate can be complex compared to structured data.
Conclusion
Graph Neural Networks stand out as a robust tool for capturing relationships in graph-structured data.
Their ability to handle complex data structures makes them invaluable across various fields where relationships between data points are crucial.
By understanding and implementing GNNs using Python and libraries like PyTorch and DGL, you can unlock new insights and potential in your data-driven projects.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)