- お役立ち記事
- Numerical calculation and simulation programming practical course using Python
Numerical calculation and simulation programming practical course using Python
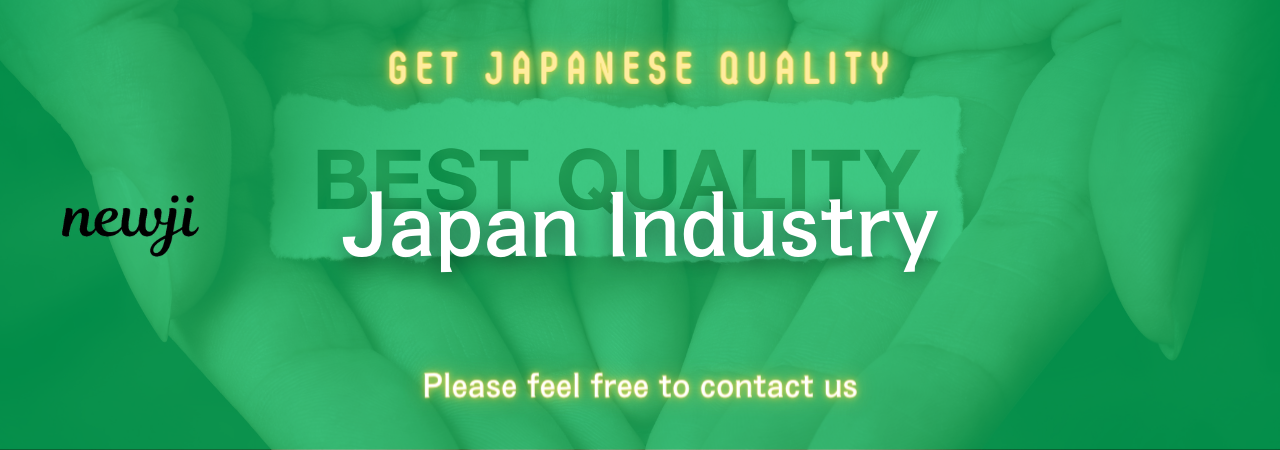
目次
Introduction to Numerical Calculations and Simulations
Numerical calculations and simulations are fundamental tools in various scientific and engineering disciplines.
They allow us to solve complex mathematical problems that would be impossible to tackle analytically.
In recent years, Python has emerged as a powerful language for numerical calculations due to its simplicity and robust libraries.
This article aims to guide you through the practical course of using Python for numerical calculations and simulation programming.
Why Use Python?
Python has gained immense popularity in the scientific community for several reasons.
Its syntax is easy to learn and read, making it accessible to beginners while still being powerful enough for experienced programmers.
Moreover, Python offers an extensive range of libraries such as NumPy, SciPy, and Matplotlib, which are specifically designed for numerical and scientific computations.
These libraries provide built-in functions and tools that simplify the process of numerical calculations and visualizations.
Numerical Calculations with Python
Numerical calculations involve algorithms and methods for solving mathematical problems.
These problems could range from simple arithmetic operations to complex differential equations.
Python, with its rich ecosystem, provides efficient ways to handle these calculations.
Using NumPy for Numerical Operations
One of the core libraries for numerical calculations in Python is NumPy.
NumPy provides support for arrays and matrices, along with a collection of mathematical functions to operate on these data structures.
Let’s look at some basic operations using NumPy.
“`python
import numpy as np
# Creating an array
array = np.array([1, 2, 3, 4, 5])
# Performing basic operations
sum_array = np.sum(array)
mean_array = np.mean(array)
“`
These operations are just the tip of the iceberg.
NumPy allows for more complex operations such as vector and matrix manipulations, which are essential in numerical calculations.
Simulation Programming with Python
Simulation programming is the process of creating a model that mimics a real-world process.
Simulations are widely used in fields like physics, biology, and economics to study complex systems and predict future behaviors.
Introduction to SciPy for Simulations
SciPy builds on NumPy and provides more advanced functions for mathematical operations, optimization, and simulation.
It is particularly useful for performing tasks such as numerical integration and solving differential equations.
“`python
from scipy.integrate import solve_ivp
def model(t, y):
dydt = -0.5 * y
return dydt
# Solving a simple differential equation
solution = solve_ivp(model, [0, 10], [1], t_eval=np.linspace(0, 10, 100))
“`
In the example above, we solve a differential equation using SciPy’s `solve_ivp` function.
This is an example of how Python can be utilized for simulation programming.
Visualizing Simulations with Matplotlib
Visualization is a crucial aspect of simulations as it helps in understanding the results better.
Matplotlib is a library in Python that makes it easy to plot data and visualize the outcomes of any numerical calculations and simulations.
“`python
import matplotlib.pyplot as plt
plt.plot(solution.t, solution.y[0])
plt.xlabel(‘Time’)
plt.ylabel(‘Solution’)
plt.title(‘Differential Equation Solution’)
plt.show()
“`
In this example, we plot the solution of our differential equation using Matplotlib.
Visualization provides a clearer picture of how the system behaves over time.
Advanced Topics in Numerical and Simulation Programming
Once you have a grasp of the basics, you can explore more advanced topics in numerical calculations and simulation programming.
Monte Carlo Simulations
Monte Carlo simulations use random sampling to obtain numerical results.
They are particularly useful in risk analysis and financial modeling.
Parallel Computing
For large-scale simulations, Python supports parallel computing, allowing the distribution of computational tasks across multiple processors.
This significantly improves the performance and efficiency of simulations.
Conclusion
Python is a versatile and powerful tool for numerical calculations and simulation programming.
Its comprehensive libraries, combined with its ease of use, make it an ideal choice for both beginners and professionals in scientific computing.
Whether you are looking to perform simple numerical operations or complex simulations, Python provides the necessary tools and resources to achieve your goals.
By mastering the skills discussed in this course, you will be well-equipped to tackle various computational challenges in your field.
資料ダウンロード
QCD調達購買管理クラウド「newji」は、調達購買部門で必要なQCD管理全てを備えた、現場特化型兼クラウド型の今世紀最高の購買管理システムとなります。
ユーザー登録
調達購買業務の効率化だけでなく、システムを導入することで、コスト削減や製品・資材のステータス可視化のほか、属人化していた購買情報の共有化による内部不正防止や統制にも役立ちます。
NEWJI DX
製造業に特化したデジタルトランスフォーメーション(DX)の実現を目指す請負開発型のコンサルティングサービスです。AI、iPaaS、および先端の技術を駆使して、製造プロセスの効率化、業務効率化、チームワーク強化、コスト削減、品質向上を実現します。このサービスは、製造業の課題を深く理解し、それに対する最適なデジタルソリューションを提供することで、企業が持続的な成長とイノベーションを達成できるようサポートします。
オンライン講座
製造業、主に購買・調達部門にお勤めの方々に向けた情報を配信しております。
新任の方やベテランの方、管理職を対象とした幅広いコンテンツをご用意しております。
お問い合わせ
コストダウンが利益に直結する術だと理解していても、なかなか前に進めることができない状況。そんな時は、newjiのコストダウン自動化機能で大きく利益貢献しよう!
(Β版非公開)